Channels
Database function skipping data
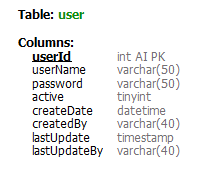
AssemblyLoadContext LoadfromAssemblyPath vs LoadFromNativeImagePath
SpotifyAPI - i dont understand things but i want this so bad...
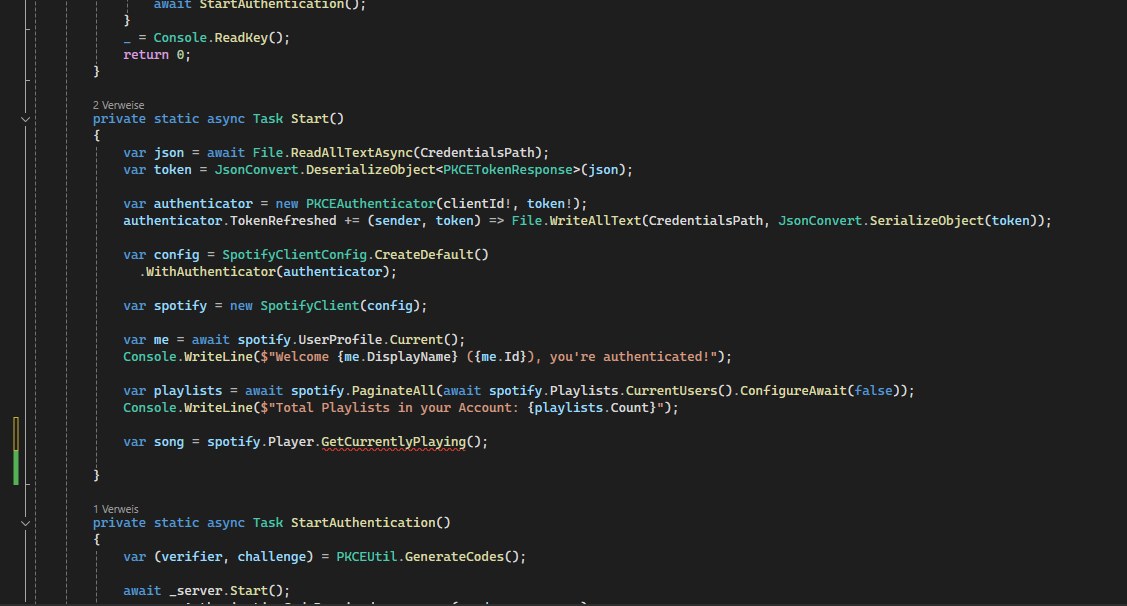
✅ WPF - how to approach a treeview displaying different types at different levels via MVVM
Does hangfire has a redudant delay?
help needed with accesing a variable in another script
Issue with websocket within a service
Help
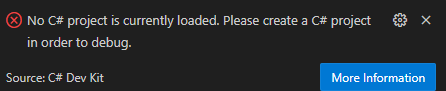
✅ How to export data from datagridview into excel file
Problem with Material Design Lib in wpf
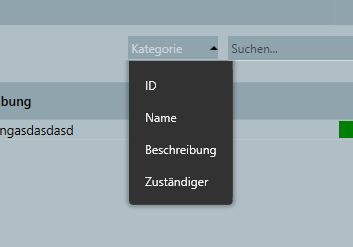
Title: Issues with Authentication and Authorization using JWT with AutoRender Mode in Blazor NET8
<Authorized>
attribute in components, as it does not retrieve the user's state. Additionally, I would appreciate guidance on how to implement RevalidatingServerAuthenticationStateProvider and Persist State in a client project.
If any of you have experience working on a project involving JWT with AutoRender Mode, I would greatly appreciate any insights or sample projects that could help me overcome these obstacles....✅ How do I reference a specific private instance of a class
Receiving Game Controller Input while Minimized (WinForms C#)
Configurations say that appsettings is returning null
✅ ASP.NET Core Web Api + SignalR
✅ .NET sdk not working