UnauthorizedAccessException [Answered]
So im trying to change a folders icon, setting it manually sometimes removes it again 🤷♂️ , so i hope this works for the time beeing.
34 Replies
im getting a UnauthorizedAccessException but i dont know why
this throwes the error
but how does it not have access if the file doesnt even exist?
The application may not have write access to the folder itself
UnauthorizedAccessException path specified a file that is read-only. -or- path specified a file that is hidden. -or- This operation is not supported on the current platform. -or- path is a directory. -or- The caller does not have the required permission.
well how do i check if my program has write access to the folder 

Try out DirectoryInfo of the target directory
Check its Attributes property which is of type FileSystemInfo


If this solves your issue, close the post by using command
/close
well kinda, im just trying to figure it out how to change that without having to go through every folder
Change its attributes so you can write to it?
like this?

Uhm, no?
Normal 128That's not a file
The file is a standard file that has no special attributes. This attribute is valid only if it is used alone. Normal is supported on Windows, Linux, and macOS.

You want Directory and without readonly
Probably best to separate out that declaration and assignment with a try-catch sector
Because you may not succeeed changing its attributes

Does it still say ReadOnly after you refresh it?
in the explorer?
No, call the Refresh() method
Nope
only directory
whats weird is that in the explorer interface it says its readonly
Is it still readonly if you run the app again?
What if you tried something like the following
whats the identity on the FileSystemAccessRule constructor?
System.Security.AccessControl

If it's a local user it's
.\username
A domain user would be part of an organization like a workplace
Is this computer part of a domain?
sry fell asleep 

found this im guessingf the workgroup is my domain?
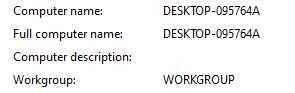
Also thought of a different approach
im creating and editing the files outside and then move them in
the problem is there seems to be already a desktop.ini file inside the folder
and with hidden items it cant be viewed
also got this enabled

with dir i can see there is a desktop.ini file

when i remove the file with cmd it works
✅ This post has been marked as answered!