Integration testing a web API [Answered]
Just for the sake of the learning experience, I wanna write some integration tests for the endpoints in my web API. What's the typical way of doing this? Do you have to use some kind of
HttpClient
setup to call the API as if using HTTP or is there some better way of just testing the endpoints individually? For context, all of my endpoints return Task<IResult>
, so I (probably) can't just test the direct return value of each endpoint method.56 Replies
Do you want to test the Http calls? Or do you just want to test the logic of the endpoint?
We do both, we start up a testhost and do http calls at it. We also allow you to replace instances with mocks if you want to not have any external calls etc. You can also just create a Controller with all the dependencies and use the functions directly
Test the logic of the endpoint
I don't think I care about testing the HTTP calls...?
I suppose I can do something like
Seems like otherwise you need to test the entire pipeline with
WebApplicationFactory
Yeah
That's... annoying
Is there at least some way to not have to write the endpoint route and instead have it be inferred from the method?
We generate a client from the openapi spec that has models and routes etc, might be very overkill
I've seen ppl make consts and helper methods for setting and calling the routes
... you can't access 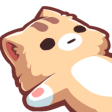
Microsoft.AspNetCore.Http.Result.OkObjectResult
???
why tf
Why do you need to do so much stuff to write some tests 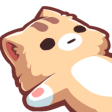
Most stuff you need to setup once, then the actual tests are small
Are you doing the httpclient route?
i don't know what you're actually trying to achieving by testing this
all you seem to be doing is testing asp.net core
I wanna test my endpoint methods?
what's the goal of the test
Test the endpoint methods
that's not a goal
that's an activity
... testing that they do the correct thing
to see if they work as expected?
people writing pointless tests lead to maintaining absolutely worthless code, if you're doing this for experience learn what makes a good test
wow, thanks 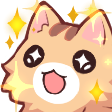
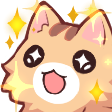
not sure if sarcasm, the point being most people end up preaching 100% code coverage and end up spamming tests which have little worth in the bigger picture of actually maintaining a project
Well what should I be doing then? I wanna learn how to write tests for stuff, because that's what I've seen other people do.
Agreed, doing tests for testing sake is bad
I can absolutely see that
that depends on the project - imitating unit tests just because you've seen them defeats the purpose of unit tests
But we tend to unit test logic heavy code and do some integration tests for the "code clue"
This is literally my first web API project and it's ridiculously small, really I don't need tests but I thought it'd be a good opportunity to learn anyway
ok but you've built the API to do something, yes?
Yes
and you'd have an idea of who would consume the API?
Yes
the integration tests should be modelled around that - "as a user of this API, when I do X I should get Y and be able to do Z"
Okay so I shouldn't be testing the individual endpoint methods but rather the HTTP routes?
ive come in with a little less context to be honest, but if you're going for an integration test that sounds more like what you should be doing
Right
I usually do both tbh
My primary motivations for web application factory tests is to test I get 401s back
This can still be done with controller tests in a manner
Okay so I'm getting an exception 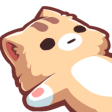
System.InvalidOperationException : The server has not been started or no web application was configured.
when calling WebApplicationFactory<TEntryPoint>.CreateClient()
why is this so damn hard 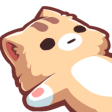
Integration tests in ASP.NET Core
Learn how integration tests ensure that an app's components function correctly at the infrastructure level, including the database, file system, and network.
That's the article I'm reading
Okay now it works when just using the first example of
var application = new WebApplicationFactory<Program>()
ingame currently so cant look 😄
This is my thing currently
It works and I guess it's fairly compact
We use something like this to move the whole host stuff outside the test
https://docs.microsoft.com/en-us/aspnet/core/test/integration-tests?view=aspnetcore-6.0#customize-webapplicationfactory
Integration tests in ASP.NET Core
Learn how integration tests ensure that an app's components function correctly at the infrastructure level, including the database, file system, and network.
How would you add a mock service to that?
We use some logic so you can replace services at startup of the host
Also when you say "we", do you mean your company?
Yeah
Swedish digital healthcare
Also do you need to mock the todoSerivce? Might be worth doing a
wait we're both swedes
again more of a case of just wanting to try what I've seen others do because I wanna learn
Plus it's kind of useful since some endpoints only need to call specific methods in the service.
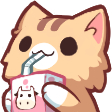
Are you using xunit?
yep
If you want we could perhaps jump in to a call and look at it, maybe not tonight tho
You could also do something simplier
Mind showing an example?
You could follow this and do a CustomWebApplicationFactory https://docs.microsoft.com/en-us/aspnet/core/test/integration-tests?view=aspnetcore-6.0#customize-webapplicationfactory
Integration tests in ASP.NET Core
Learn how integration tests ensure that an app's components function correctly at the infrastructure level, including the database, file system, and network.
also completely unrelated, is your pfp literally the ica logo?
Yeah
love it
Maybe something like this
Im sure there are better ways
You could also just move some logic to the ctor a function to keep it simple
Do this and save the application and mock in a readonly field
I gtg
This got messy but 😄
I need to go to sleep anyway
Thanks for the assistance 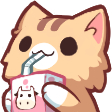
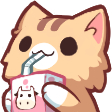
Net6?
If you’re using top level statements there’s some things you have to do for web application factory
yes
✅ This post has been marked as answered!