Calling a function from a string.
Hey, everyone! I have a timer that will run every 100ms, but I have a list of functions named "f1", all the way up to "f255". I know it sounds impractical to even have all of those functions, but I need them. Anyway, is it possible to have an int++ to call the next function on the list? I want it to do this every time the timer runs:
1: add 1 to the
framei
int.
2: add the 'f' at the start of the function name, then attach framei
after it
3: call the function
Expected behavior:
100ms elapsed,
f1();
another 100ms elapsed
f2();
and so on.
Here is an example of the code that doesn't work: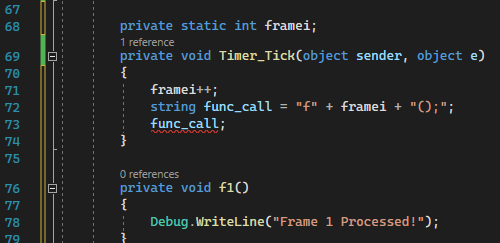
97 Replies
why would you possibly need all those functions?
I'm doing a challenge. Run bad apple by manually updating a pixel grid that was created in XAML
Each frame is a function, setting pixels that need to be set
I told you it's impractical, but I want to do it
So is it possible?
i feel like this would be better of in one single method honestly
What do these methods do?
print a bunch of full size blocks i guess
If they just print the same thing but with a different number then use one method with a parameter
Manually updates each pixel on a pixel grid
I haven't actually implemented that yet...since I need this to work first
It is possible to call a function knowing its string, but I am not sure that's the solution here
"bad apple" is a song with a music video. people have started playing said music video on weird stuff
just like "running DOOM on a calculator" or whatever
https://youtu.be/2QiDb_khg44
Yup. Precisely. I have the frontend working, just not the backend
How would I do that?
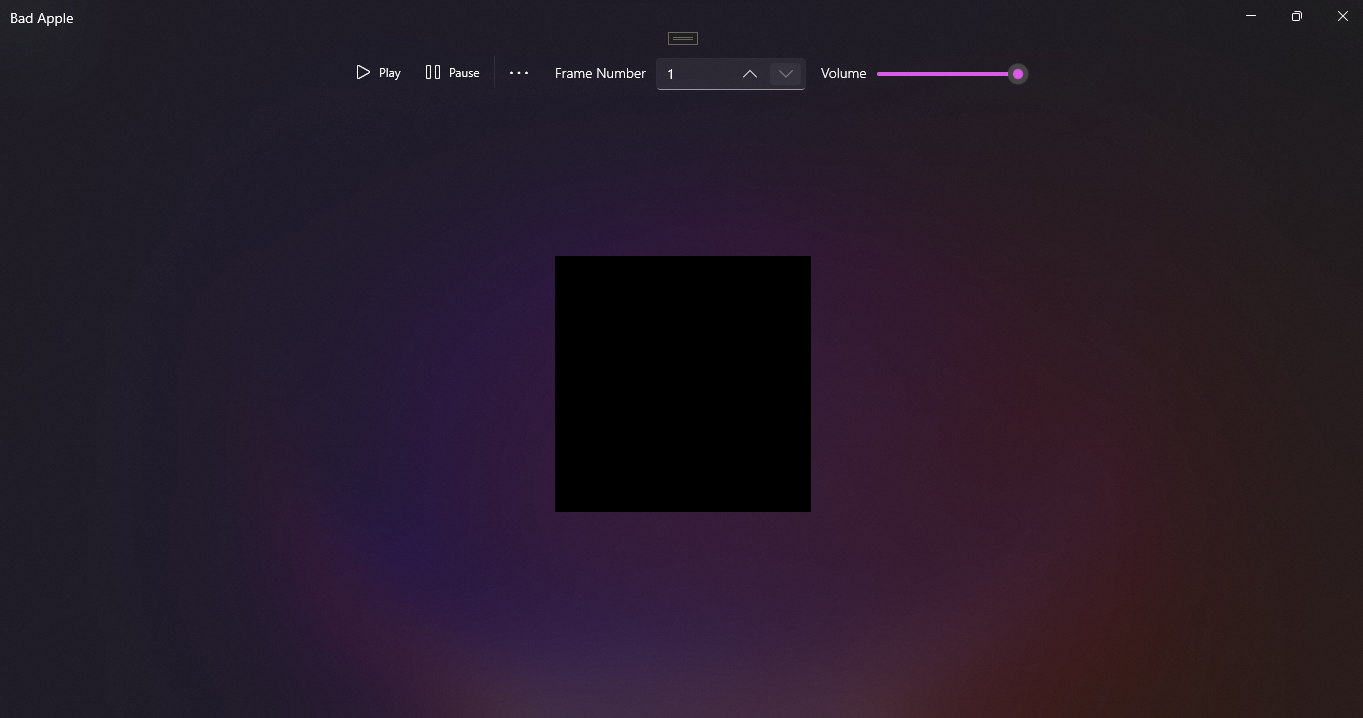
Don't in this case
This is the app btw. That black square is actually made up of many small squares
Calling a function by it's name is only necessary when you have no other option
It's slow and kind of "bad code"
Would it still hit that 100ms mark?
Like execute within 1ms
you probably want like
a list
or if you must, a dictionary
This is a 3532 frame video or something like that
So fun
and just access the frame via the index/key
Oh, so like
Kinda
i would just do this?
and then do
Wouldn't going through a list every time the timer hits be slower though?
what do you mean?
Than just directly generating the call every time
no?
it will be so much faster
you don't create the list anew every time
Ohh, okay. That makes sense
I'll try this out
Thank you
just for education's sake, the way that you're suggesting(the very bad way) might look something like
classObject.GetType().GetMethod("namehere").Invoke(classObject, null); or something
it's called reflection, if you still care to look it up
you probably don't need the GetType call
oh cool, that works too
but yeah, reflection is incredibly slow, and incredibly bad code
This seems to want C# 9.0

and why are you on such an old version?
This is a UWP app
That's what the blank app generates
hm
just don't use target typed new then
just put List<string> after it right?
it's really not a big deal yeah
Ugh
My visual studio install broke again
Atleast I can still do this
@Ero Would I just add each method as a string?
what do you mean?
It doesn't like _frames.Add("f1);
i'm not sure what you're referring to
you'd put whatever you want to print on that frame
Wait, so this doesn't call another method? This frame can require from 1-256 changes at the same time
The methods need to be separate from the list because it would become a jumbled mess
you don't even have any of the methods
f1() and f2() for testing
this is literally your entire code
Yeah, but the pixels are different for each frame, so it can't be in one single set of code
what do you mean? you'd obviously have the pixels be different for each list entry
Ok. So what I mean then is it won't let me create an entry. I've never used a list before, only arrays and ObservableCollections
You just...put that?
yes
Does each comma start the next frame?
it doesn't "start" anything
it's just a different list entry
accessible by the list's index
you could even use an array, might even be better
By the way, each pixel is set by a color value
So example:
P12 = Colors.White;
P24 = Colors.Black;
That would change the color of each pixel
Oh okay. Also, it won't let me access the pixel grid from the list
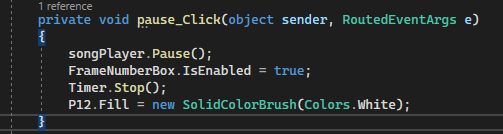
If I put it into an event handler, it's there
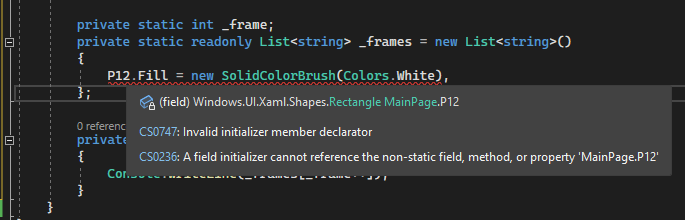
In the list, it has no idea what it is
@Ero Is there a fix for this? I think it has to do with routing the event(?)
the issue lies with that the list is expecting a string inside of the initializer however you are trying to assign a brush to a control property inside of that initializer
Yeah, but even trying to get the P(number)s to come up doesn’t work either
I don't follow?
Basically, each pixel has a name: (example), P1, P2, P3, and so on
Each "pixel" is created in xaml
But I can't access the pixels from the list
Okay, I understand what you're talking about now. Is there a way to fix this?
Can I make it expect a control property?
what exactly are you trying to do
So...I have a grid of "pixels" (rectangles) and I need them to be updated every frame.
Bad Apple is two colors, black and white
So every frame, some pixels will have a
P1.Fill = new SolidColorBrush(Colors.White);
or a
P1.Fill = new SolidColorBrush(Colors.Black);
And I have P1-P256
Each rectangle is one pixel, in a 16x16 gridright, so im assuming you will be reading this pixel data in from somewhere
Nope. This is a challenge, I will be manually creating each frame in a function
So P1-P256 can be changed each time the timer is called, to whatever value they need to change to
I tried earlier, the List thing didn't want to work
just create a class which defines a frame, then create an ordered list of frames
then you can loop through your list of frames and render them based on your frame definition
creating 256 functions for each frame is really stupid
Oh I was gonna create 3288 functions to update each pixel at once
Lol
Though that would be like 800k lines of code (not even joking, I did the math)
i don't really see the point in doing that
So a loop that counts up to 3288
That makes sense, but the issue lies in the list itself
The List does not want to cooperate with me...at all
@Anchy
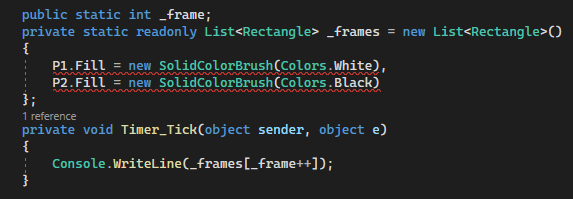
Here is the issue
It doesn't want to let me create a list of pixels for each frame
This code is what the person who previously tried to solve this gave me
you could do something like this I suppose, however even this would be really slow
As long as it hits around the +-5ms mark, it'll be okay
I have no idea what the performance would be like
You can thread diff parts of the image youre recoloring
but ye idk the performance either
This code seems to be for an actual pixel grid. The "pixels" don't have x and y values. Instead, they are all assigned names
wat
I know, it's confusing, but the challenge was to create a grid of "pixels" in XAML, and then change their color each frame

Look at this xaml
oh you mean the Rectangles
This is literally how each pixel out of the 256 are made
well just replace X and Y with one Rectangle then
Up to 256 of them will need to be changed at once
Don't think that'll do
again, trying to do this by hand would just be a lot of work for no reason
i'd sooner try and read the video file directly and fetch the pixel data before trying to hardcode each frame
This gives the same issue as before
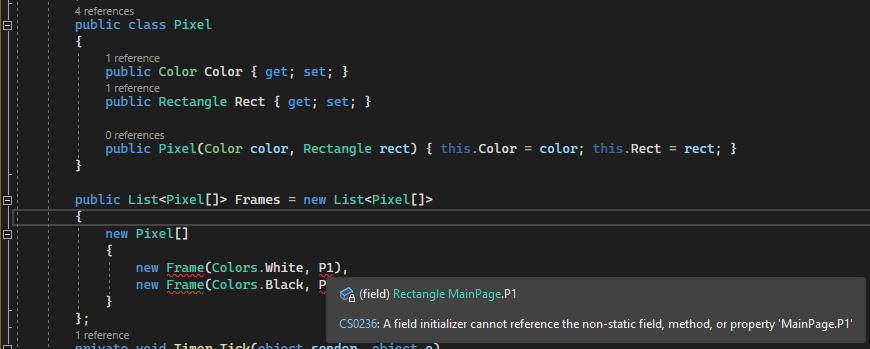
The original video is 320x320
The content area of xaml pixels is 16x16
oh rename Frame to Pixel
originally it was called Frame

I'm talking about these
It doesn't like the fact that it's pointing to a rectangle
So unless I put 102,400 small rectangles on a page, that's not gonna work
no it just doesn't like using that reference inside the initializer
so instead I would just create the list inside the constructor
Actually, I could do that
I have a 16x16 dump of all the frames

Why doesn't discord support bitmaps
Anyway, these are pretty good. I could try and "decode" these for each frame
I think the System.Drawing namespace supports opening bitmaps and you can even loop through all of the pixels
Awesome! Will try it out tomorrow. Thanks!
(sorry this is not related to your question but is this WPF? Because it looking quite good)
No, it’s UWP with winui 2.8 lol
It would be a lot of work to make a WPF app look this good