✅ I don't understand GC
The output of this code is True, False. Why? Both instances of Class1 don't have any reference pointing to them. But only one of them is garbage collected. What am I missing?
2 Replies
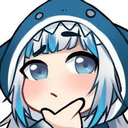
Was this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.
Closed!