interactionHandler parse showing TS '...cannot be named without reference to...' error
When attempting to make a very basic interaction handler I'm getting the pictured compiler error. The guide suggests that I shouldn't need to explicitly type the parse function so I'm not sure why I'm getting this error.
The code:

Solution:Jump to solution
Summary for Answer Overflow: The issues was caused by pnpm (the package manager I was using) using symlinks for @sapphire/result. Hoisting all sapphire packages did not help. Switching to yarn in place of pnpm has solved the build issue.
31 Replies
Heres the error in text if needed
Downgrade sapphire result
To 2.6.0
What why would a downgrade of result be needed lol
In fact you shouldn't need to manually install result at all
In fact 2.6.0 is latest @kaname-png smhhh
It looks like a pnpm issue Ben
maybe you can do me a solid here @Ben855. Add a tag to @Spinel
pnpm
with content:
and if you can add a page with about that content in the Additional Information category of the guide that'd be even more awesomeI’ll give that fix a shot and the open a few PRs in several hours
Pinning the version to 2.6.0 fixed the problem for me using pnpm
¯\_(ツ)_/¯
Unless I missed something here, adding the hoist pattern doesnt seem to have causes pnpm to do anything differently. Everything in the
@sapphire
dir in node_modules is still simlinked .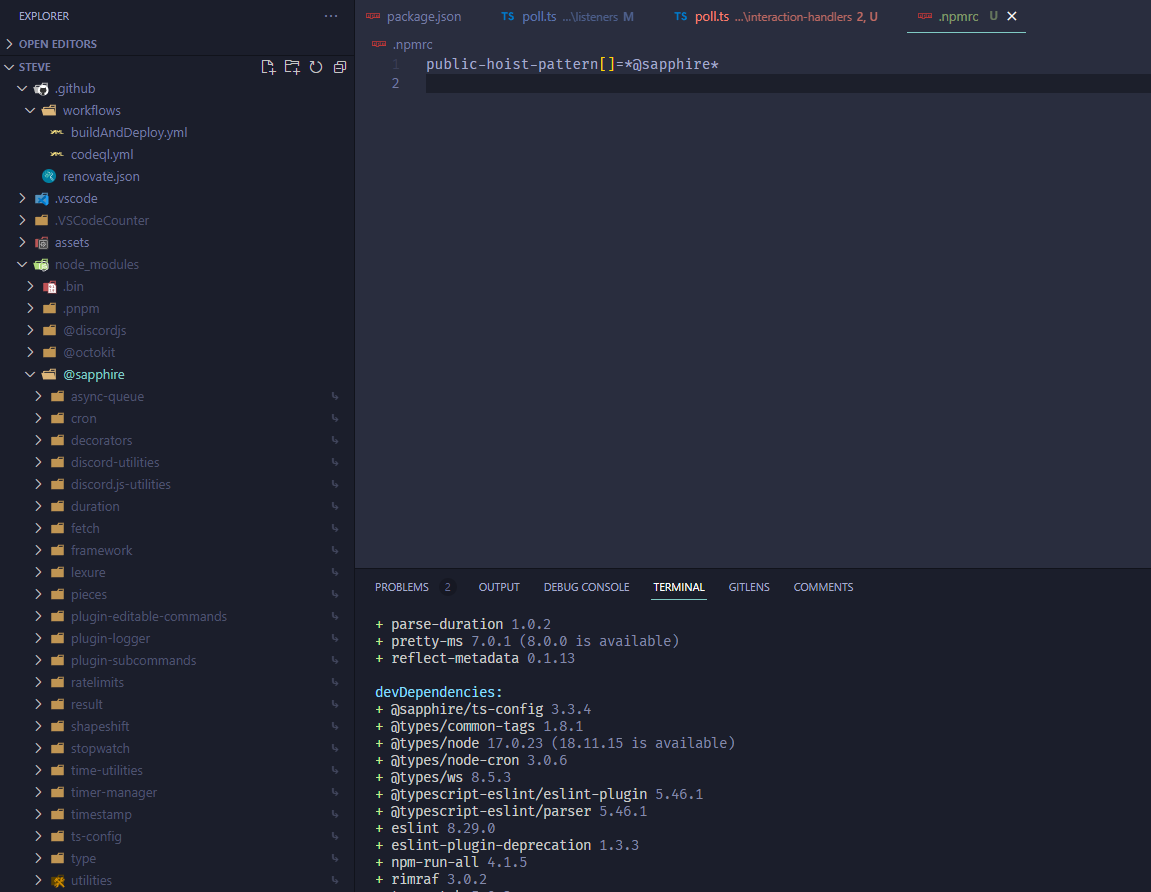
I did also yeet my node modules and lockfile then reinstalled
adding framework without hoist:
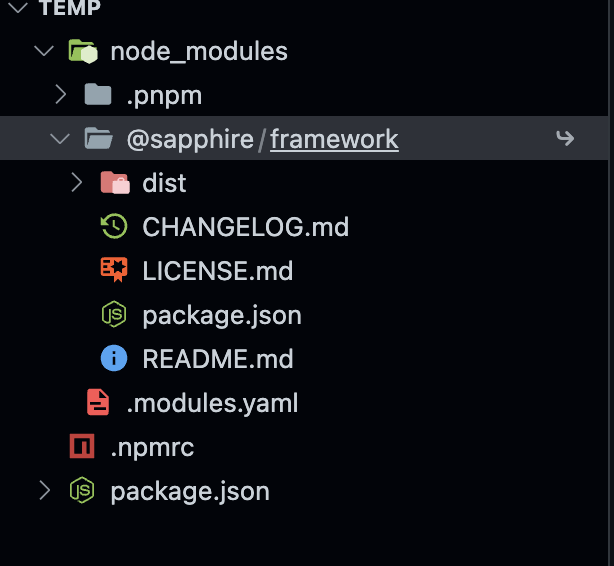
adding framework with hoist:
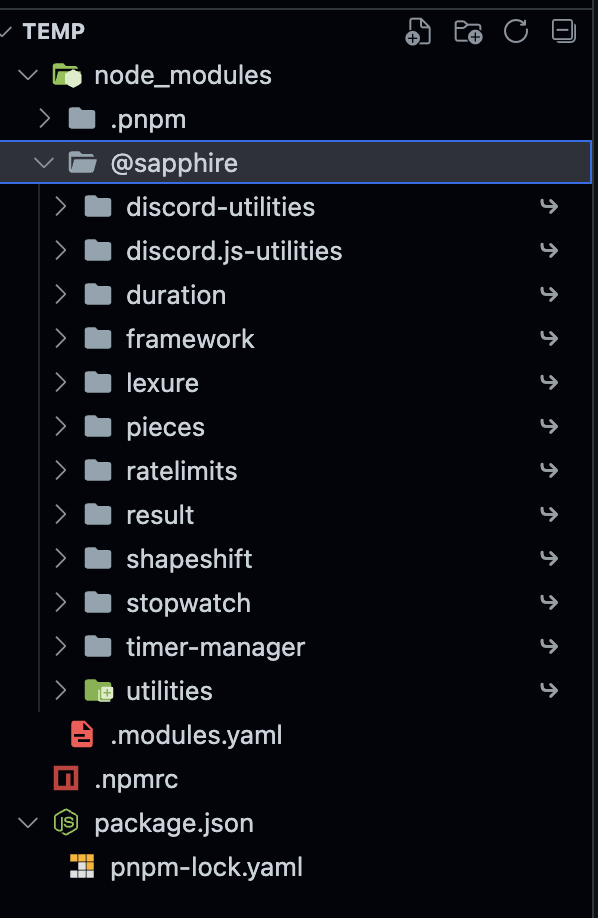
so yes it does change things
TypeScript doesn't read types that aren't in
node_modules/@types/
or node_modules/**/package.json:types/typings/export.types
Both those framework folders look simlinked
and when was this ever about framework

Right I know the problem is with result being simlinked. I'm just confused since both the two file tree screenshots you posted have everything in the @sapphire dir simlinked.
they all have the little simlink arrow to the right of the folder name
mate
the first screenshot only has framework
nothing else
Yeah I know that
all the other dependencies are not in node_modules at all
TypeScript doesn't read types that aren't inlike I said TS literally cannot read the shit that pnpm does with dependencies without being patched by pnpm on install which pnpm doesnt donode_modules/@types/
ornode_modules/**/package.json:types/typings/export.types
Right okay so then why doesnt this work?
compare that to Yarn which does do that
Again, pnpm DID do something different
I already explained it like thrice now
So neither of the file trees you posted would work then right
I would assume the latter one does
with the hoisting
heck if I know, I don't have your source code to try
GitHub
GitHub - BenSegal855/Steve-V3 at tmp-for-Favna
Contribute to BenSegal855/Steve-V3 development by creating an account on GitHub.
What I was saying is that my filetree screenshot matches your second file tree screenshot unless I'm not seeing something correctly. So if your second one works, whats wrong with mine
nothing is wrong but as far as I am aware you haven't checked whether the issue persists yet
I have
That was the first thing I did after reinstalling with the updated .npmrc
well idk then
I never use pnpm
yarn god
pnpm for doing stupid shit that TypeScript doesn't support and then proceeding not to provide TypeScript patches
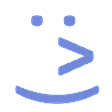
It pnpm is gonna cause this issue then without a good fix, I'll switch to yarn.
Any particular version of yarn to use?
I dont know to much about it since I havent used it that much
3
1 and 2 are deprecated
Yarn v3 is new version of Yarn that we recommend switching to as Yarn v1 has been deprecated.
"But I don't see any update on [source]?"That is correct. Yarn v3 is installed through Yarn itself. Your global toolchain is and will always remain to be Yarn v1, however, you configure Yarn v3 on a per-project basis. How? Simply write: This will download the new Yarn v3 binary and put in
.yarn/releases
, you should push this to your Git repository. It will also create a .yarnrc.yml
file which configures the path which you should also commit.
Next you probably also want to run the following 2 commands:
This will add to your .yarnrc
file:
Which ensures that you just have a Yarn v1-like experience with node_modules
and a global cache.
Next step is to nuke your node_modules
and yarn.lock
and run yarn install
Then some final adjustments. Put this in you .gitignore
:
And anywhere in your scripts in package.json where you use *
you should wrap it in extra "
For example:
Mind you this last thing is good add regardless of script runner / package bundler because it ensures the glob is performed by the library and not by your shell, which may differ when people develop on different operating systems.
In short the command to set everything up you can run:
Thanks
Solution
Summary for Answer Overflow: The issues was caused by pnpm (the package manager I was using) using symlinks for @sapphire/result. Hoisting all sapphire packages did not help. Switching to yarn in place of pnpm has solved the build issue.