How to do nested <For
I have a signal that is array of arrays
I want to render it out on screen and for solid to update it. However I read https://www.solidjs.com/tutorial/flow_for and still not too sure how to do nested <For.
I tried this:
But it doesn't work.
In react it's quite simple, I just do
Thanks for any help.
SolidJS
Solid is a purely reactive library. It was designed from the ground up with a reactive core. It's influenced by reactive principles developed by previous libraries.
38 Replies
ok this works I think
š¤
I am not sure why the grid is not updating
GitHub
test/snake.tsx at main Ā· nikitavoloboev/test
Trying new things. Contribute to nikitavoloboev/test development by creating an account on GitHub.
GitHub
test/snake.tsx at main Ā· nikitavoloboev/test
Trying new things. Contribute to nikitavoloboev/test development by creating an account on GitHub.
I console logged, the values do change
I can't console log inside the component function itself
as it only runs once
but I thought that if I render it out with jsx it would update
imo you should use <Index> for that as the index is what's identifying the input element - not reference because changing 0 to 1 is changing the reference
something like this?
get some type errors
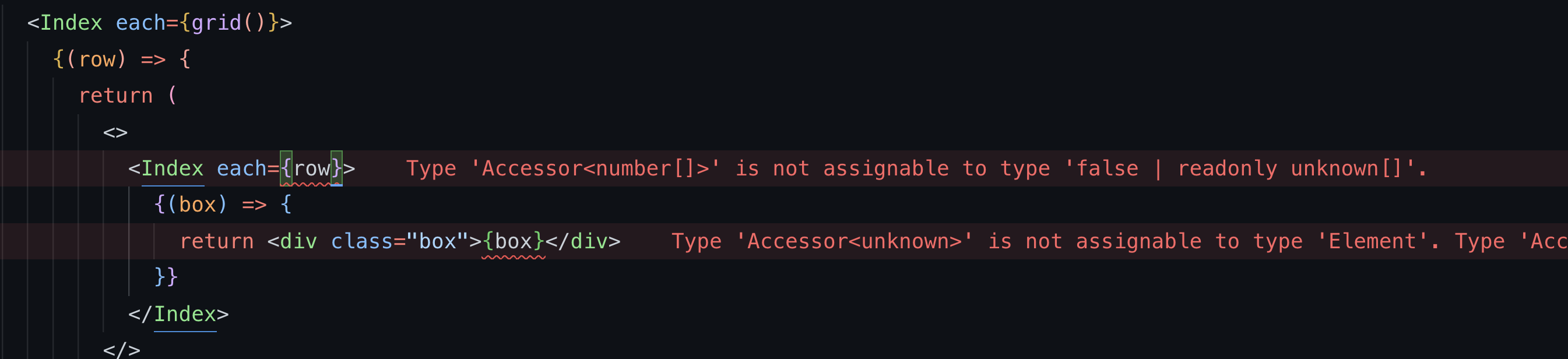
SolidJS
Solid is a purely reactive library. It was designed from the ground up with a reactive core. It's influenced by reactive principles developed by previous libraries.
Also, since the two components are to be derived from a single signal - both of them need to be subscribing to it
So the nested <Index> should actually reference the signal as well in the
each
functionreading its docs it seems like its similar to For
And thirdly, using a store would probably be the best
I have one component though
so I put both snake and grid inside one store
why doesn't it work with <For though
trying it with store
When you call
newSnake
, line 155, you supply the same array (although some elements are mutated). The signal doesn't change if it's assigned a value that is equal to the old value (eg. same array)
You can opt out of that with the createSignal optionsSolidJS
Solid is a purely reactive library. It was designed from the ground up with a reactive core. It's influenced by reactive principles developed by previous libraries.
then
moveSnake(state, setState, direction)
I get this I guessIn my oppinion this has nothing to do with For vs Index
I have Q about this actually
Using a store would solve the issue
reason I creates those mutable variables
is that I can't do
setStates
there
and expect changes to be instant
they are not
thats quite a big change
if I move it to store
hope it will solve it
I
not sure how to type store wellCheck if `setSnake([...newSnake]) solves the issue. By cloning the array it should always notify the subscribers
so here
do this?
that console log there won't be updated value
and I need new value of snake
later in function
or here
you mean
should I continue with migration to store or that won't help
Solid Playground
Quickly discover what the solid compiler will generate from your JSX template
You can either create a new top level array with a spread, or alternatively use
{ equals: false }
option on the signal to ensure it the signal fires each time the setter is called, even if it's the same array.
The example of @thetarnav creates a copy with slice instead of spread, but it's similarexample of @thetarnav is blowing my mind a bit
trying to make sense of it
The secret is that I'm always calling
grid()
in each place it has to track it.
Since this is a single signal, and you only reference some property of it without calling it, it won't track the change.
Using createStore gives you that each property access is trackableGitHub
test/box.tsx at main Ā· nikitavoloboev/test
Trying new things. Contribute to nikitavoloboev/test development by creating an account on GitHub.
I am now trying to
So no component gets recreated, BUT all the places that call
grid()
will have to reexecute on each update to check if they don't have to update. So it's diffing, just smart diffing.
Using createStore will eliminate that issue as well - you subscribe to a single value directlymove @thetarnav code to use my functions
to update
without store
for now if I can
as that would be a big diff
so if I get it right
it seems I can't pass setters to my function?
like my
moveSnake
in the end it should just update the signal value
then DOM should change
as signal value is updated
im confused why it doesn't š
I checked that logic is valid if I just console log updatedGrid
and newSnake
at end
like the grid has right values and all
I will try to add things on top of @thetarnav code instead of combining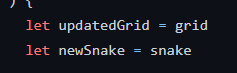
those will be the same references
ohh
ok but if I don't do it
and say do set things instantly
how do I get current updated values inside same function
?
But I would just destructure the top-level signal values and apply the change
ok will try thank you
like Big Thief too btw š
this is the first time I saw a function that takes both value and the setter š

I would never pass the setter around
yea it did look ugly
I just thought thats how you had to do it
like im coming from react
that would work in react
or well you can actually have the function inside component
so you don't need to pass it too
I mean I like to have a single place where the state is updated, then it gets confusing where the change actually happens, is it batched or not, idk
oh I missed that
ā¤ļø
ngl I have yours "Inspired" playlist often on repeat