❔ Return IEnumerable backwards
Hey! I got a method which returns a specific collection depending of the parameter. Right now, I needed to add a method to return similar result, but
Left.Lane
collection should be returned in backward order.
How can I do it in the more compact way (and without errors)?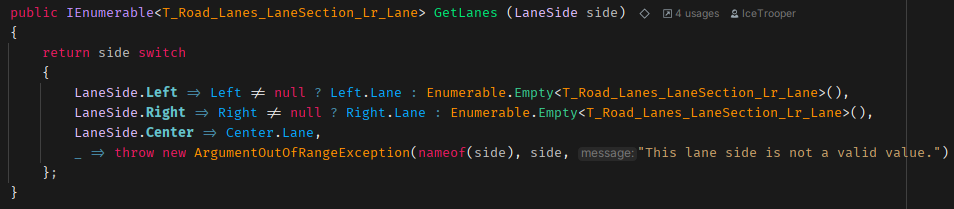
30 Replies
Old, working method:
New, not working method (that method should return Left colleciton in reversed order:
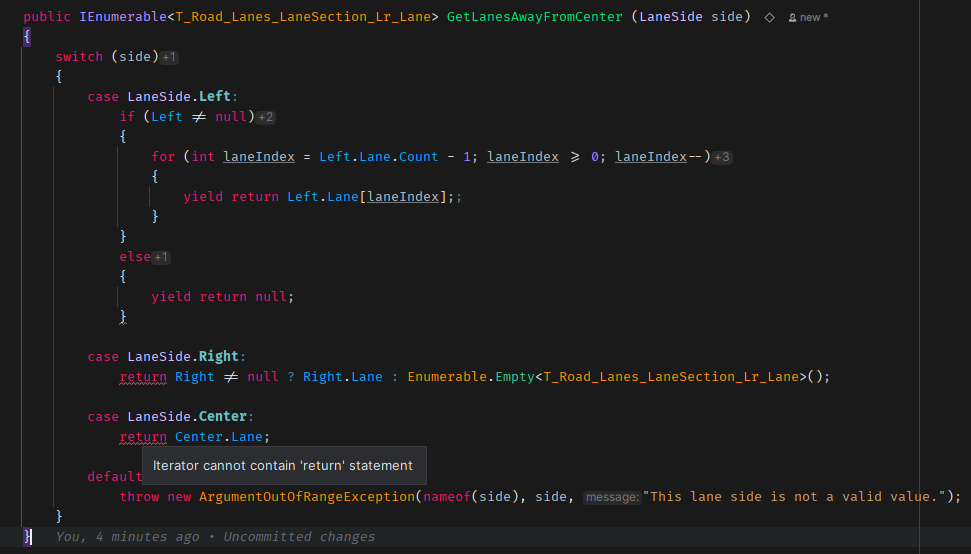
you could use .Reverse() on the collection i suppose?
Reverse will call another IEnumerable, it wouldn't be performent 😅
unrelated but wtf is that type name
T_Road_Lanes_LaneSection_Lr_Lane
it being an IEnumerable is already bad for perf
make a custom iterator if you care about that
External library type
I ended with something like this. I don't know how I can simplify this
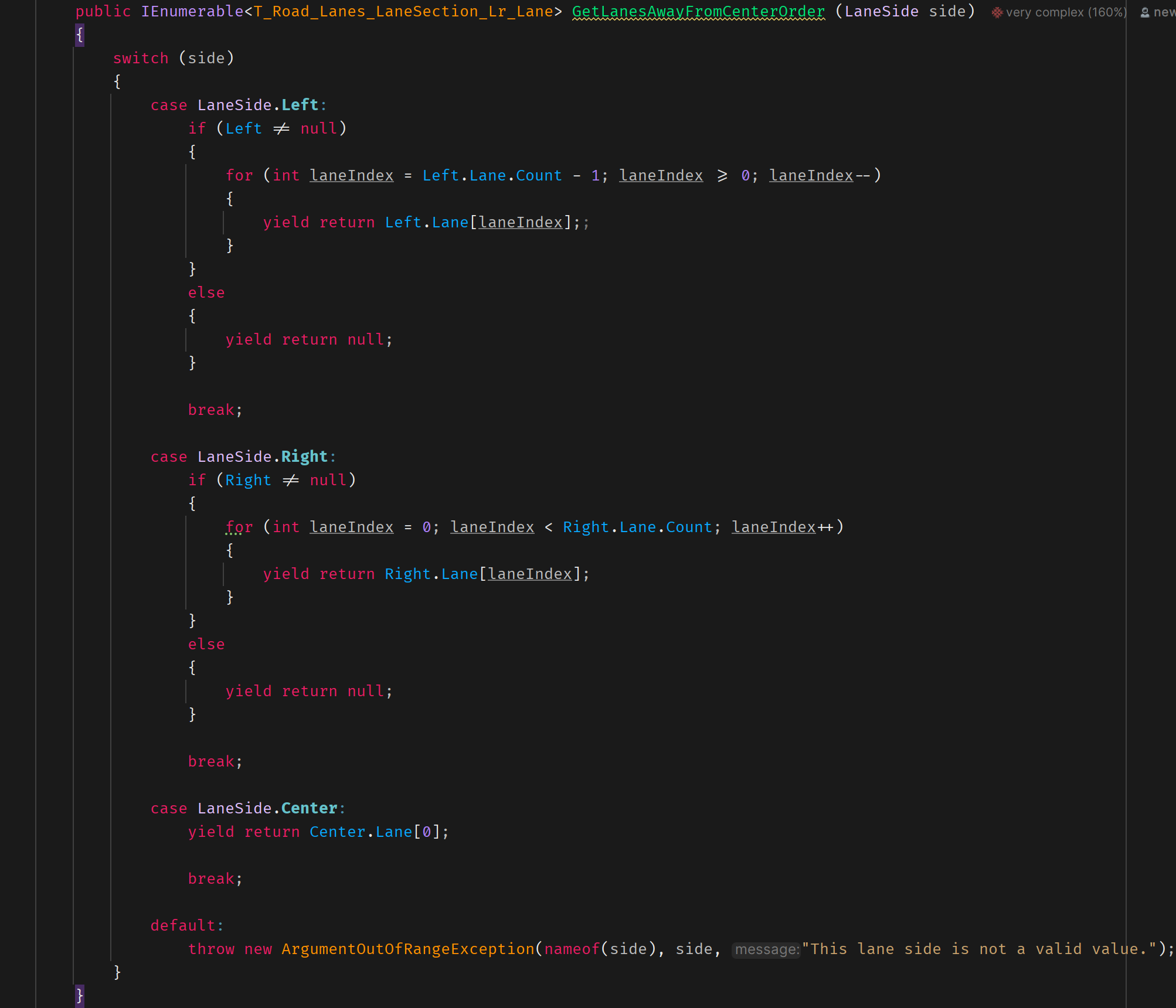
but Reverse built-in method would call another unnecessary IEnumerable 😅
if you make a custom iterator type it won't have to
you can make the iterator reversed and also be a value type
to avoid allocations
if you're just going to foreach over it, it will be better
You're using enumerables in the first place. You can't possibly be concerned about perf
yeah the functional features in c# are implemented in a way that's quite bad for both perf and memory
it's intentionally made more dynamic
And what you have there sure can be simplified a good bit
dude, com on...
I'm working in game dev, so yeah I'm not joking about performance ;p
If I have a list, that is, an indexed collection then I don't want to unnecessarily call an additional IEnumerable to Reverse if I can do it in a more efficient way. I just want to write this in the more compact way.
BTW I changed the above code to
yield break
instead of yield return null
because of the error.yeah again, if you actually did care about perf, you wouldn't use enumerables at all
foreaching over an enumerable is incredibly costly
something as simple as
becomes
you're even throwing exceptions...
how many times would this code be called per second?
also, have you tested performance at least?
You're right that foreach is more costly than for, but I wouldn't say that so much. Anyway, I've used them here to use the deferred execution functionality 😅
This exeception... should be exception. I've made it there only to throw something if someone would add new LaneSide (which should not happen) and it wouldn't be supported here.
This method could be called at least once per frame
seems reasonable
and collection size?
Reverse would make new allocation for a stack, then loop once over collection, and then again to use it. That's why I'm so reluctant to use the Reverse method
When I code it myself it loops over collection only once and without any allocations. In that case the code is just quite long which I prefer to simplify :f
Quite small (in the worst case max 16)
So yeah, IEnumerable for small collection is not great. Still want to use deferred execution.
to me spending time optimizing this seems overkill
as of now the only way i personally could think of simplifying it would be going unsafe
it's hard to make any suggestions without more context
You're probably right. And I don't want to use unsafe hah.
Last question, could this code above be more readable for other programmers?
Should I rewrite something?
what was the issue with this?
too slow
but i don't see how
Ice_trooper#2729
Reverse would make new allocation for a stack, then loop once over collection, and then again to use it. That's why I'm so reluctant to use the Reverse method
When I code it myself it loops over collection only once and without any allocations. In that case the code is just quite long which I prefer to simplify :f
Quoted by
<@!654411275094327296> from #Return IEnumerable backwards (click here)
React with ❌ to remove this embed.
i don't understand
i mean either way, you could
yield return forces an allocation anyways
Was this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.