Any way to create another instance of a List<Tuple> with the same setter?
I have this within an abstract class:
Is there any way to simplify it?
I am basically writing the exact same logic for 2 tuples, just with different names.
Thanks!
26 Replies
Firstly, use
(string, string)
(ValueTuple<string, string>
) instead of Tuple<string, string>
I'm fine with Tuple<string, string> honestly
The frontend reads it fine and its not that ambiguous
Anyone can deduct what header.Item1 & header.Item2 are
It's not about ambiguity or anything like that
It's more efficient, less allocations, not a reference type, can be equated
What does equated mean? @Ero
I don't see my Tuple being inneficient for my use case though
A major problem with the tuple class is that it is a reference-type with memory allocation on the heap. Due to allocations and garbage collection pressure, using it can lead to CPU-intensive operations and major performance issues in our system. The ValueTuple on the other hand is a lightweight value type object and has its memory stored on the stack. This means that with ValueTuples we can build better-optimized systems.- https://code-maze.com/csharp-valuetuple-vs-tuple/
Ok
I'll change to ValueTuple
How could I fix the problem I'm facing in the title of this thread though?
You could select on it
now 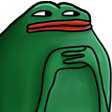
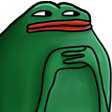
Don't use literally
ValueTuple
Thinker showed you how to do it(string, string)
List<(string, string)>
private List<(string, string)> _HeaderVT;
public List<(string, string)> HeaderVT
ok
but how do i create like a "reusable type" out of that like the title asks
with the same setterWhat do you mean?
"reusable type"?
that was probably the wrong way to describe it
but
ill show you
imagine this used a valuetuple
ignore the tuple<string>
how would I prevent boilerplate here
im technically writing the same setter for the two instances
surely theres a way to make this shorter and cleaner?
is there not a way to create like a "custom" ValueTuple that contains the getters and the setters already?
seems like im still repeating some code here no? @Ero
to be fair, its pretty short now, but just for learning purposes is there any way to do this?
make a record struct for that
and how would I override the .Add method? I just realised that i can't use getters and setters because its a collection and im adding individual elements
you're overcomplicating it
the problem is this doesn't work @AntonC
also setters should be idempotent
because setting is not the same as adding
do AddRange then
but adding elements in a setter is just wrong
it should be idempotent
idempotent
i simplified it
as you said, i am complicating it
this works

make a method
AddCookies
that takes an IEnumerable
of those tuples
it means applying the same operation does nothing after each consecutive application
make a named record struct instead of that tuple
use the implicit new()
for the field initialization toook