ReactiveMap
I am having an issue with reactive map - i think i know why it's happening but i have no idea how to fix it.
I have the below code
Setting up the Store (static map just for testing)I am trying to render it as such: Now, it renders two components - however it renders two of the first mapped object. Which i don't understand at all. What am i doing wrong?
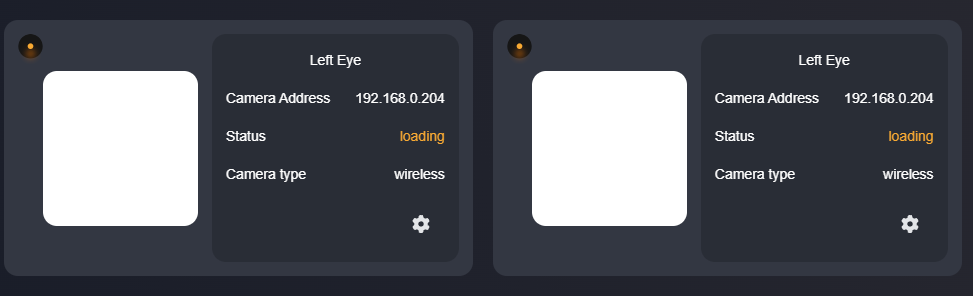
16 Replies
if your keys are strings, why not use a store?
not sure i understand
i thought i was creating the store correctly 😅
I mean instead of ReactiveMap
easier to manage 1 api then 2
also this would probably work better
i thought using the map was nice because i can lookup a camera based on the device name later on. Just a
get()
and set()
api to retrieve or add camera'syou can have the same with store if keys are strings
i am totally new - i am not really sure how i would do that. I am from c++ - maps are in my blood xD
forgive my ignorance xD
isn't that just an array of objects?
or object of objects
yeah no problem
they are common use in normal js too
but stores natively support only plain objects and arrays
you can import 3rd party lib for reactive maps, but you may just be adding complexity needlessly
and thats coming from a person that created the solid-primitives/map package 🤣 😅
i understand 🙂 thanks for the heads up
I am going to switch
this doesn't work
This get's close, but throws an undefined error
it comes off of the iterable that
keys()
returnshow about this
no,
Property keys does not exist on type Accessor<ReactiveMap>
ah, its key
nope
i was wrongcameras is probably meant to be _cameras -> value not a signal
liquid is just gone xD
lol
I mean it's in his name
yeah, this works perfect
but i agree - i should just use an object of objects
thank you both for the kick in the right direction
this might be useful for you then
https://github.com/solidjs/solid/issues/1300
if you want to have similar interface
but not necessary
GitHub
Creating a store from another breaks read-write segregation · Issue...
Describe the bug You can easily create a store from a "read-only" proxy returned by another, gaining write access to the state and causing reactive updates to the original store. ...
small update - migrated the camera component away from map - purrs like a kitten now 🙂 thanks ya'll