Confirm with password
I want to perform deletion upon password confirmation. Any suggestions on how i can do that?
62 Replies
What do you want to delete?
Like you want to support the user deleting their account after verifying their password?
like i want to delete a member from team_joined_user table upon password confirmation
I am thinking of fetching the password through a form and then verify it using
current_user.hash_password
and the password entered by user. for verification, I am thinking to use Bcrypt.verify_pass(password, current_user.hash_password)
Purpose of asking here was that i might be able to customise the action of destroy for team_joined_user
to verify the password and delete it or like to create a code_interface for the password verification π
Well, I'd probably suggest that you could add a change in the action that checks the password of the current user before completing the action.
Might want to make that a special action called
:destroy_with_password
, or make the password allow_nil? true
and ignore it if there is no current user, that way you have some way of destroying them without setting a current user if you don't want toShould i be defining this in my junction table resource?
In the above example, yes
But there are lots of different ways you could do it, that is just an example of one of them
Anyway, then when creating the form in your UI, you'd say
Form.for_destroy(team_joined_user, :destroy, actor: <current user>, api: YourApi)
I will be creating this as a seprate module and then call this in my juction table resource?
Well, kind of
a "change" is a way to plug some logic into an action
you don't "call it" per-se
for suppose i call it
destroy_with_password
then? and i will define it in require_password_confirmation.ex
. if yes then shouldn't it be in
Yes, it should be
I was showing both parts in one example
but the action goes in your actions, and the change goes in a different file
um, let me just clarify.
so, i will be calling this in my actions of team_joined_user resource:
and this part will be in new file maybe called
require_password_confirmation.ex
containing:
right?Yes
and then call this for my form part
Form.for_destroy(team_joined_user, :destroy, actor: <current user>, api: YourApi)
Yep!
is this a built in function or do i need to define it ?
valid_password?(actor.hashed_password, current_password)
Depending on what your UI looks like you may need to change it, but that is a basic version that would allow you to select a join record, and make a join form for it that requires a correct password for the signed in user
You need to define it
I am getting a hang of how things work in ASH eco system. I am very keen to explore more of it.
is this right?
looks right to me π
gives me an error
π
need a bit more information than that lol
sending
oh, sorry
I can send you the stack trace in private if you want
you need to provide the actual record as the first argument
not
TeamJoinedUser
but the_team_joined_user_you_want_to_destroy
let me check
now it says it needs current_password as argument but i just want the form to be created for now
Try removing the
%{actor: actor}
and see what is in the third argument, context
on it
I found the mistake
i thought i can replace the
actor
key with current_user
but it was expecting actor
yeah, its always called
actor
inside ash resourcesso i will be doing the same i usually do for saving the form, right?
in save handle event
Yep
something is wrong as i entered the wrong password and it still deleted the member π
you'll have to debug the change and see if its getting there and whats going on
noted
my valid_pass function is giving false but it still destroyed that entry
And youβre adding an error to the changeset if itβs not valid?
yes
the way you said
Can i see the whole change as you have it now?
The module I mean
Can you inspect the changeset after adding the password?
And make sure that there is an error there?
Sorry
After adding the error
yes i am on it
I have this in the inspect of
it said
valid?: false
and still deleted it.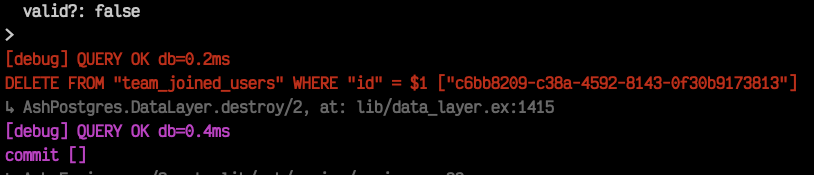
this must be a bug with adding errors in
before_action
hooks
have a fix, will push up after I run checksyou found the issue?
yes
Please let me know once you push the changes so i can fetch the latest version
Hey @talha-azeem can you please check the behavior when using with the main branch of Ash?
{:ash, github: "ash-project/ash"}
sorry for the late reply. Let me try and update you
still same issue
π€ were you already on a github release before?
Or did you just switch
i just did
let me try to put the whole link
i deleted the deps and now fetching it again now it is pulling from the github
let me update you in a min
I am getting this error if i fetch it directly from github while trying to add a record in my junction table which was working fine before
yeah, please update ash_postgres to the latest
in addition to this
same behavior
valid_password/2
returns false. It goes to the else part adds the error and then deletes it π
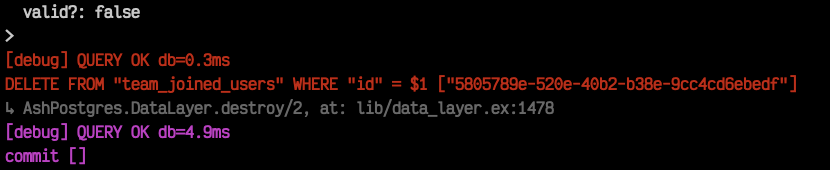
errors part from the changeset βπ»
ah, yeah sorry missed one thing
No Problem. I appreciate the fact that you are providing me fix on the run so that my work can continue β€οΈ
okay try main now please π
use
mix deps.update ash
to fetch the lateston it
kudos @Zach Daniel , It works now
π₯³
β€οΈ
Nice work team.
Great Work π«Άπ»
@Zach Daniel , A quick question. Can i utilise your ash-hq user settings page?
Its MIT license, you can do whatever you want with that code π
you could copy it entirely if you want
okay can you tell one thing?
π
please make a new thread for it, but yes π