❔ How to convert line endings from my not encypted string to my encypted string
I tried already
But that does not work
28 Replies
i Encrypt my text
But
it does not copy the lines
because i wanna write that in a file
but it goes all in 1 long line
and not like before in more lines...
i hope anyone can help me 🙂
Has no one a idea?
:/
idk what you're doing but I can point out two things about the code you should improve
1. use early returns
2. using has a second form that applies to the current scope
use that form
using var stream = new blah();
like that?
did you even change anything
i try to give me Encrypted string the same line brakes
yes...
they mean like this
oh
yea well thats cleaner
thxx
But do you know
how i can give the EncryptString the same linke brakes
no clue, sorry
:/ ok
That is how it should look after a line
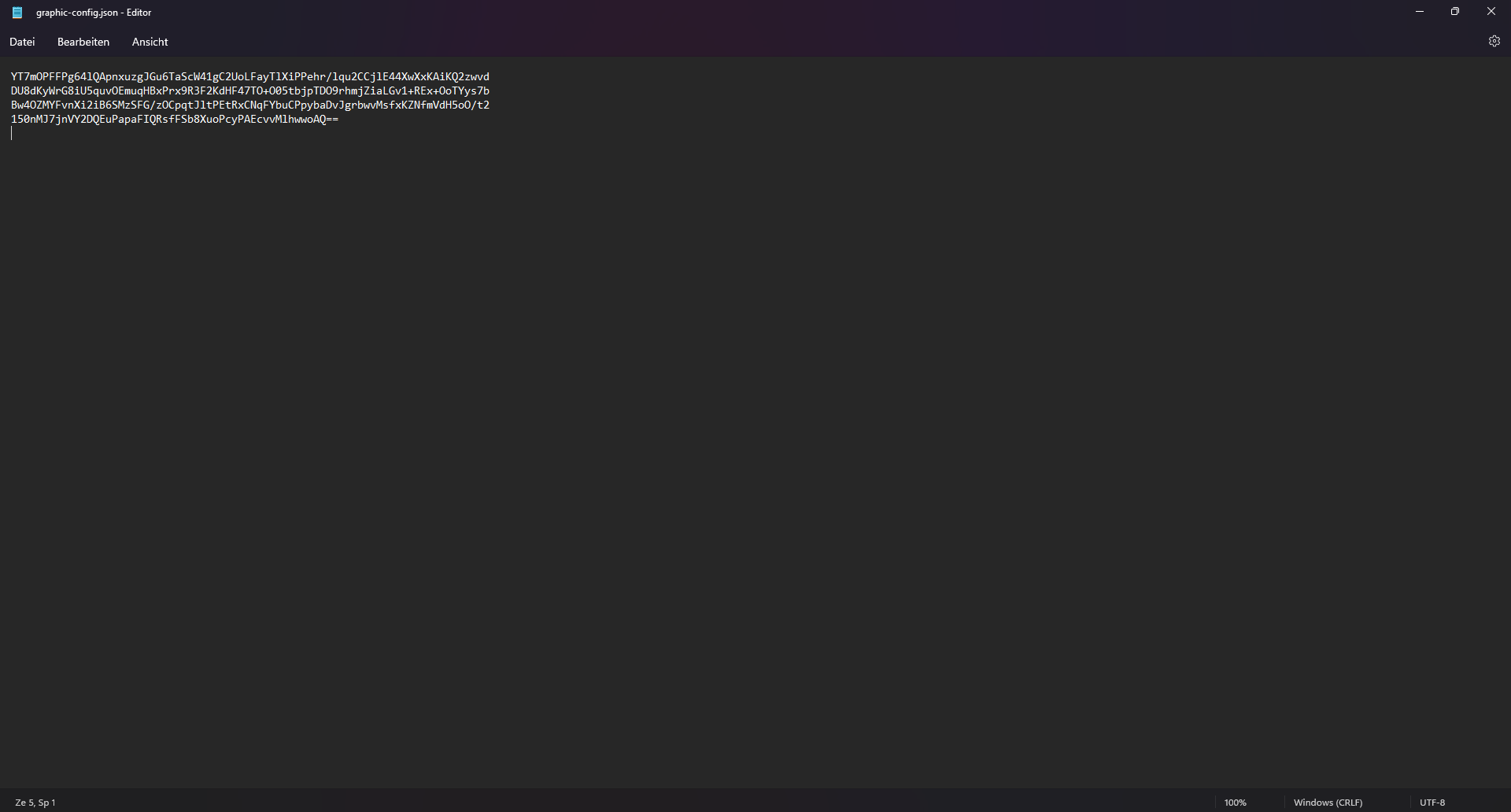
but it does just 1 very long line
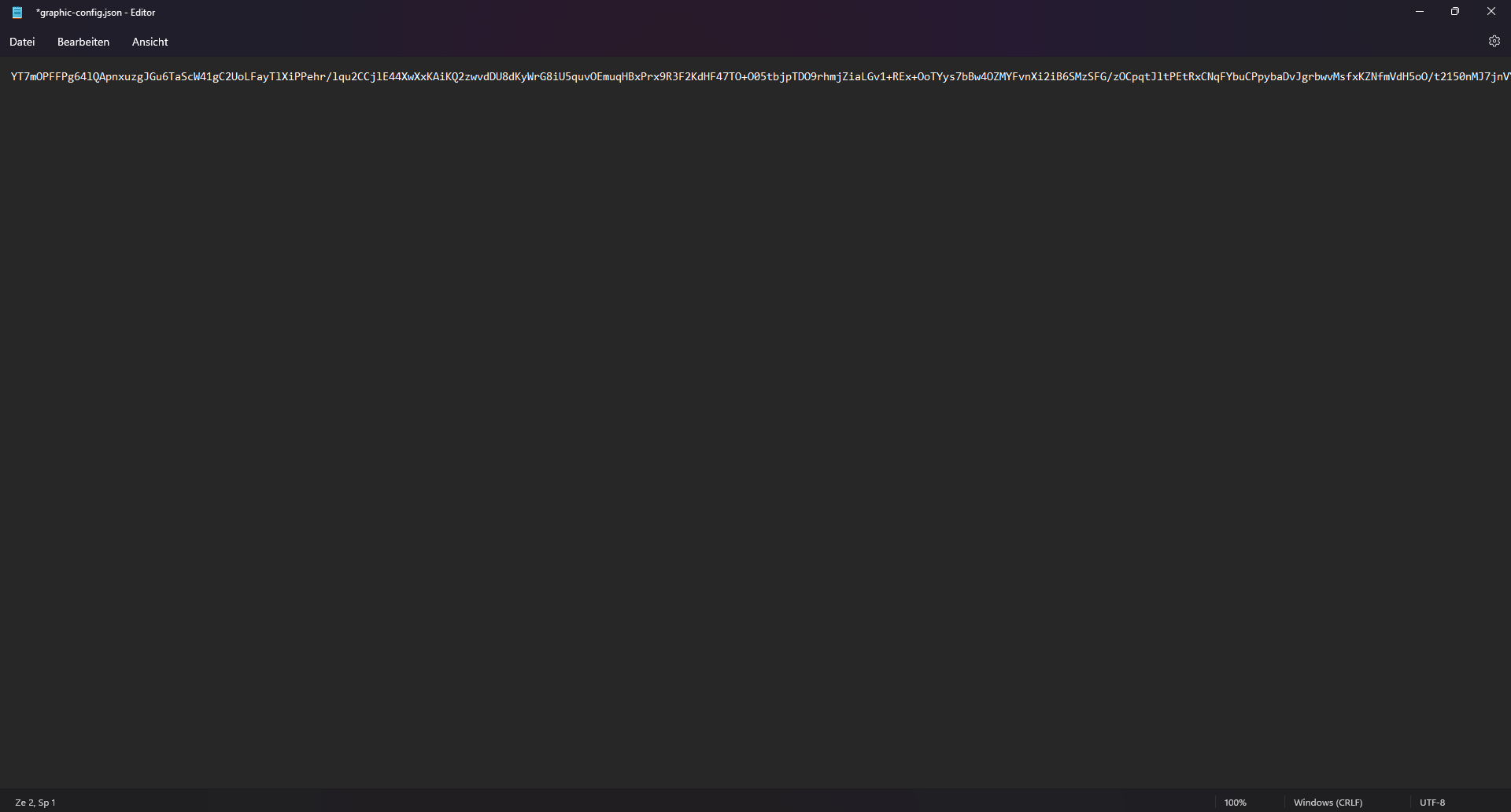
i try to do the old line brakes before it get encrypted to the encrypted one
any one a idea?
so just to clarify, you want to keep the line breaks from the original string? and somehow take those over to the encrypted one?
or do you just want to add line breaks after a certain amount of characters
cause i mean,
Base64FormattingOptions.InsertLineBreaks
existsyes
but i don't know if that's what you want
alright well, that can't work
that can never work
thats not possbile?
the only way i see this happening is if you first
.Split()
the string on the line breaks
and then encrypt each line individuallyouf
is it possible to get the count of characters of a line?
then i remove the spaces in it
and then i split it with the encpyted one every time when a normal line should end
would that not work?
btw this code does not work... (EDIT: i fixed it)
and return then a array?
and then
.Join
again
with Environment.NewLine
as the delimitorhey question
is it allowed to have just 1 character in a line?
because {
sure
you sure?
of course
because my console print
don't really know, sorry
thats my Decrypter
ouf
Was this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.