❔ Adding to Arrays Via Methods
I am having trouble getting arrays to be changed by methods. I tried lists as well. Please send help.
82 Replies
You can't append items to arrays
They're fixed-size
Use a
List<T>
@Angius thanks but look at this
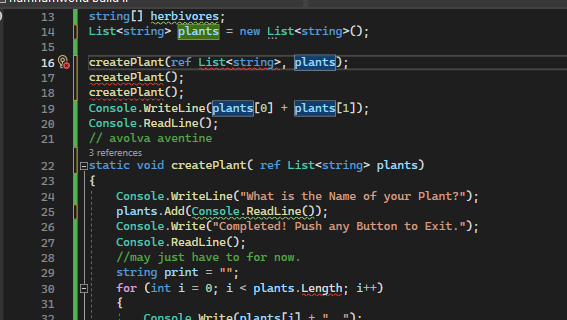
its error after error
The parameter is declared as a
string[]
, so obviously it won't take a List<string>
Also, in your CreatePlant()
method you crate a brand new array
You don't add anything, you replacecan you explain that
What's to explain there?
Square peg doesn't fit into a round hole
int
and string
are different types
So are boolean
and UngaBunga
where is the square peg
So are
string[]
and List<string>
List<string>
is the square peg
The method has a round hole, string[]
type parameter
List<T>
is not T[]
oh ignore the writeline
Nothing to do with any writeline
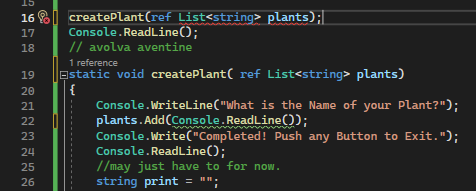
right here where is the []
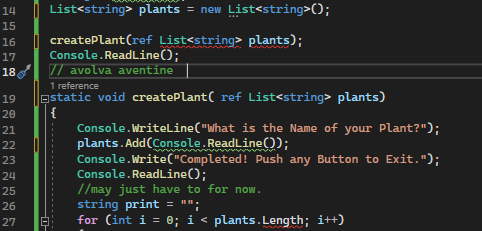
and ignore line 27 for now
im just trying to get the errors off the first part until it functions
Aight, so, why do you try to pass
ref List<string> plants
as a parameter?
Do you also use Console.WriteLine(string "message")
?apparently to change a variable in a function you have to use ref
I tried just "plants" but it said it has to be written like a list
Yeah, in the parameter declaration
was there a better way of doing that
When you call the method, you don't supply a type
You just pass the param
wym
CreatePlant(plants)
oh

like this?
Not here
Jesus
$helloworld
Written interactive course https://learn.microsoft.com/en-us/users/dotnet/collections/yz26f8y64n7k07
Videos https://dotnet.microsoft.com/learn/videos
im used to javascript
You really should brush up the basics
its much easier when I could do something like:
appendItem("plants", "");
which I dont even know if thats a real thing
And you can
because I learned from code.org
List
has the .Add()
methodyeah see I get that
but I called plants in main
and im trying to add in a method
and its causing tons of errors to get it through the parameters
like in js, everything is global pretty much
I just say:
and var becomes 2 if I call the function
in c# i'm just lost with methods and why they are so isolated
you have to use ref for some reason for it to change anything, and even still its like a maze to get the errors to be broken
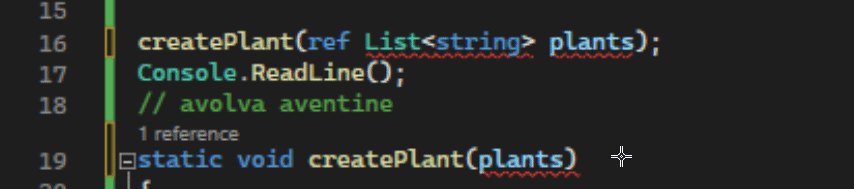
For reference types, you don't need to use
ref
oh let me read this rq
And here's the fix to your issue
You declare types when declaring the method
There and only ever there
When calling that method, you don't declare any types
Just pass the params
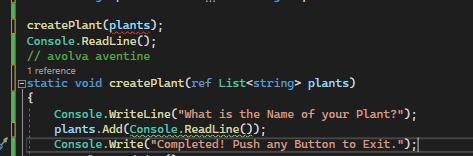
Cool, what does the error say
argument 1 must be passed with the 'ref' keyword
Well, try that
oh wow
ok brb
ok yeah its cleared up
next issue though
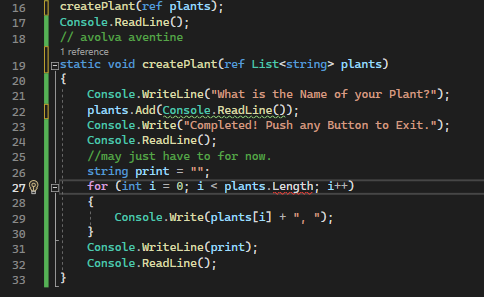
Again, what does the error say?
probably says that
.Length
property doesn't exist on List<T>

That's because those are the properties
List<T>
has: https://learn.microsoft.com/en-us/dotnet/api/system.collections.generic.list-1?view=net-7.0#properties
.Length
is not among them
.Count
, however, isare there any substitutes
ah
thats weird
i'll try it
oh wow thats wonderful
it works
ok now I need a few clarifications so I really grasp this
this is whats confusing me
its the whole parameters and redeclarations thing
The method declares what it wants
like this one, that wants a
string
and an int
When calling that method, you don't need to say that you're passing a string
hold up
No need to do
because the method will not let you pass anything else. You just do
wow
so ref only needs to be sent in the declaration of the method
Admittedly, I haven't really worked with
ref
it seems so obvious, swapping syntaxes really gets me a headache
To begin with,
List<T>
already is a reference typea what?
You're already passing a reference to it, not a copy
$refvsvalue
In C# there are value types — mostly the basic ones like
int
, bool
, etc
And reference types — custom classes and stuff
Value types, when passed, create a copy
Reference types pass a referenceoh
so I only use ref when I need to make the copy change something outside
Angius#1586
REPL Result: Success
Result: List<int>
Compile: 606.838ms | Execution: 36.419ms | React with ❌ to remove this embed.
ref
coerces value types to be passed as referencewait
so saying a variable prints it?
I see you wrote list
That
list
without a semicolon is just something the repl of the bot doesah
I had trouble earlier with printing arrays
Angius#1586
REPL Result: Success
Console Output
Compile: 681.702ms | Execution: 78.655ms | React with ❌ to remove this embed.
Here's how
ref
works for value types
Without ref
, the change happened inside of the method, the copy of the number was changed
With ref
, the change happened inside and outside of the methodSorry my network is acting up. So I get it now! reference = ref. if I did val to a ref then would it act like a value?
No
You can pass a value type as a reference, but you can't turn a reference type into a value type
hm
and I know this is off topic
but what do you do for the " cannot implicitly convert type 'string' to 'int' " error
int.Parse()
for example
Or better yet, int.TryParse()
But this one's a little more complexwhat
what do those mean
Angius#1586
REPL Result: Success
Result: int
Compile: 475.533ms | Execution: 31.322ms | React with ❌ to remove this embed.
what does the word parse mean
It's the name of the method...?
Like
Console.WriteLine()
I'm guessing over all it converts
It parses a string into a number, yeah
You could say converts, sure
and one last question
how do you close the program through code
Once it completes, it'll just close
IIRC you can just
return
from it as wellah ty
thats everything, thank you for your help!
Anytime
Was this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.