CSS Transition does not get animated properly when applied in style={} directly
I have a navbar which I have animated (attached), however to achieve this effect I needed to go in a roundabout way.
Basically, the transition needs to switch directions based on which path the user is navigating to, and the issue is that if I compute the transition in the
style
prop of the div, then the transition updates only after the transition has occurred, rather than influencing the transition. To solve this, I have an effect that sets the style programmatically before the signals are flushed, causing the transition to animate properly.
Having a style applied as such:
does not work.
I'm wondering whether there is a better solution to this problem, and whether I am missing a proper method to deal with this sort of issue.
You can view a running copy here: https://stackblitz.com/edit/solid-start-latest-g8yyym?file=src%2Fcomponents%2FNavbar.tsx
Thanks in advance!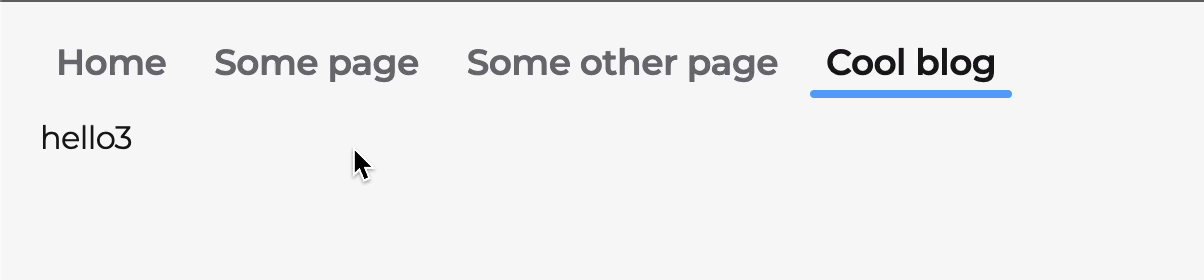
4 Replies
Unknown User•16mo ago
Message Not Public
Sign In & Join Server To View
No, those are fine. Those just transition the animation faster.
Unknown User•16mo ago
Message Not Public
Sign In & Join Server To View
Yeah I agree-that's why applying the transition styles in the effect works-it's applied before the dom is mutated by solid