SIA
Sapphire - Imagine a frameworkdisclosuure
UserErrors not being caught by listener - bot is crashing
messageCommandError.mjs
S
Spinel•398d ago
- Which version of
@sapphire/framework
are you using?
- What's your file/folder structure?
- Did you use the CLI to generate your bot?
- What's your "main" property in package.json
- Are you using TypeScript? And if so, how are you compiling and running your code? That is to say, what are your build and startup scripts?
- In case you are using version 3.0.0 or higher of @sapphire/framework
, and your problem related to message commands, did you add loadMessageCommandListeners
to your SapphireClient
options
Remember that if you are new to @sapphire/framework
it is important that you read the user guide.
When asking for help, make sure to provide as much detail as possible. What have you tried so far? Do you have stacktraces that you can show us? What are you trying to achieve? Try to answer these questions and others, so we do not have to ask for them afterwards.
❯ For a good guide on how to ask questions, see the instructions that StackOverflow gives. You should try to always follow these guidelines. ❯ For an excellent video that shows how not to ask technical questions is this, watch this YouTube video by LiveOverflow. ❯ Asking technical questions (Clarkson) ❯ How to ask questions the smart way (Raymond)
D
disclosuure•398d ago
- Which version of @sapphire/framework are you using? latest
- What's your file/folder structure? attached
- Did you use the CLI to generate your bot? no
- What's your "main" property in package.json? src/index.js
- Are you using TypeScript? And if so, how are you compiling and running your code? That is to say, what are your build and startup scripts? no
- In case you are using version 3.0.0 or higher of @sapphire/framework, and your problem related to message commands, did you add loadMessageCommandListeners to your SapphireClient options? yes
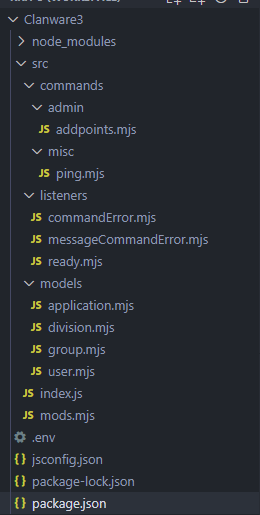
D
disclosuure•398d ago
F
Favna•398d ago
whats the code for
addpoints.mjs
D
disclosuure•398d ago
let me upload it one sec
F
Favna•398d ago
if you can put the full code on GH that would probably be faster
D
disclosuure•398d ago
F
Favna•398d ago
const amount = parseInt(args[1]);
should at best be const amount = Number(args[1]);
btw because messageRun
passes the arguments as number, number
, not as string, string
or better yet just make group-id
for chat input a number and dont parseInt or cast at allD
disclosuure•398d ago
group-id can be large though which is why i parse as a string
D
disclosuure•398d ago
GitHub
GitHub - cosigyn/Clanware-V3
Contribute to cosigyn/Clanware-V3 development by creating an account on GitHub.
F
Favna•398d ago
that doesnt matter for discord options...
D
disclosuure•398d ago
doesnt node.js have difficulty with large numbers
F
Favna•398d ago
no...
D
disclosuure•398d ago
i see
F
Favna•398d ago
well unless it exceeds 9007199254740991 I suppose
which is
Number.MAX_SAFE_INTEGER
but that would go for any language
and again, then you should also parse it as a string for messageRun
however messageRun
as await args.pick('number')
so you're not doing thatD
disclosuure•398d ago
yeah its possible, and you're right
but do you know if I'm doing something wrong with the user errors?
F
Favna•398d ago
should also note that
usage
isnt a valid command option and if you want detailed help for help you should put it in detailedDescription
so:
otherwise it'll just end up getting nuked at runtime without proper class extensions which you're not doing - and using detailedDescription
is better anywayD
disclosuure•398d ago
i plan to access it and the emoji later for a help command but ill look into detailed description
F
Favna•398d ago
command.detailedDescription.usage
vs command.usage
ezpzD
disclosuure•398d ago
oh i see i thought it only accepted a string
F
Favna•398d ago
except that the latter would mean you'd have to extend the sapphire comand class and add the properties for no real reason
string | DetailedDescriptionCommand | undefined
is the type
with DetailedDescriptionCommand
being an empty interface to allow TS users to module augment it
anywayD
disclosuure•398d ago
yes i havent extensively looked into the docs aside from the guide
F
Favna•398d ago
as for the error
The only thing you are missing is the identifier but I don't think that would prevent it from being caught by the listener
F
Favna•398d ago
here is the fixed up file
F
Favna•398d ago
or not, thjanks for the embed discord
https://hastebin.skyra.pw/ayobayoxeb.mjs
D
disclosuure•398d ago
thanks
F
Favna•398d ago
can you try making sure that
loadDefaultErrorListeners
is true
in your client options? see if it gets caught thenD
disclosuure•398d ago
yeah its true
F
Favna•398d ago
oh..
well then try with the fixed up file at least
seeing as you were missing various things
I fixed:
-
this.run
not being awaited
- the consistency of string vs number as we discussed above
- adding identifier
to all the throws
- (messageRun
) switching from pick(..).catch(() => null)
to pickResult
- (messageRun
) removing the NaN
check for amount
because args.pickResult('number')
already does that
- (chatInputRun
) marking group-id
and amount
as required
- (run
) properly checking if the channel is available before trying to send a message to it
- (run
) removing the number parsing for amount
D
disclosuure•398d ago
this worked
thank you
also, how do i prevent it from showing in the console, if the error is handled?
F
Favna•398d ago
false here
D
disclosuure•398d ago
i see
F
Favna•398d ago
Just saying, these are all runtime fixes that were all raised by simply dumping the code in a TypeScript file (
.mts
, to match the .mjs
). I understand if TypeScript may be daunting to learn but I can very strongly recommend you do.
note: see #Announcements -> stick to TS 4.9.5 for now until we have time to bring out new releases.F
Favna•398d ago
correction: exception goes to the top point which wasn't so much typescript itself as the ESLint plugin for typescript code. That said, they kinda go hand-in-hand.

D
disclosuure•398d ago
I haven't really used ESM at all before, but I will start to look into types and things - I don't really understand many of the core concepts yet
F
Favna•398d ago
kudos that you're starting with ESM right away though
many people would have stuck to CJS
and you already figured out the hashes for importing rather than using relative paths, that's also something a lot of people struggle with
D
disclosuure•398d ago
i got sick of packages not supporting cjs
F
Favna•398d ago
ironically there are also packages that don't support ESM 

D
disclosuure•398d ago
ah 💀
F
Favna•398d ago
but ESM is the future
I don't expect it to happen any time soon but Node will deprecate then remove CJS eventually
D
disclosuure•398d ago
I haven't used JS outside of node.js so ig that's why I've been using CJS
Also would you recommend setting up an event emitter for success responses/logging user actions
F
Favna•398d ago
For @Dragonite I have it but I only load it when logging level is set to debug and that's only the case when NODE_ENV is 'development'
Dragonite and other bots are open source btw:
S
Spinel•398d ago
Discord bots that use @sapphire/framework v4
- Official Bot Examples ᴱ ᴰ ᴶˢ
- Archangel ᴱ ᴰ
- Dragonite ᴱ ᴰ
- Radon ᴱ ᴬ
Discord bots that use @sapphire/framework v3
- Arima ᴱ
- Nino ᴱ ᴰ
- Operator ᴱ ᴬ ᴰ
- Sapphire Application Commands Examples ᴱ
- Spectera ᴬ
Discord bots that use @sapphire/framework v2
- Materia ᴱ
- RTByte ᴱ ᴬ
- Skyra ᴬ ᴰ
- YliasDiscordBot ᴬ
ᴱ: Uses ESM (if not specified then uses CJS)
ᴬ: Advanced bot (if not specified it is a simple bot, or not graded)
ᴰ: Uses Docker in production
ᴶˢ: Written in JavaScript. If not specified then the bot is written in TypeScript.
D
disclosuure•398d ago
thanks
Welcome to the Sapphire Discord server! The next-gen object-oriented Discord.js bot framework can be found here.
2.2KMembers
View on DiscordWant results from more Discord servers?
More PostsSubcommand isn't runningWhen the subcommand is run in Discord, it times out. I've added a log to the beginning of the methodHow to properly handle UserErrors?I'm using the official sapphire template and want to throw a UserError inside a context menu commandCan I set different requiredUserPermissions for each subcommand?Just wondering if this is possible, and how. ThanksHandling BulkOverwrite Registry ErrorsWhen I encounter this error
```
[ERROR] ApplicationCommandRegistries(BulkOverwrite) Failed to overwrEvents for VoiceHow can I do something like the following but for voice?
```
import { Listener } from '@sapphire/frHow do I use autocompleteRun on subcommands?^ As the title says. I can't seem to figure out how subcommands can have autocompleteRun implementedMessage editing fails even though message can be fetchedWhenever I want to edit an ephemeral message I get the following error:
```js
[ERROR] Encountered erProblem with ephemeral messagesI currently have a command which sends a button, problem is, once the button is pressed, I can't remIs recommend have split autocomplete handlersI want know is recommend have split handler such as characterAutocomplete & itemsAutocomplete.Error serverHow can I solve this error ?
```
Error [ERR_SERVER_ALREADY_LISTEN]: Listen method has been called mQuick question regarding application descriptionsHow does one link an application command in the description of an app? Looks like a mention syntax, What typescript version to use?Since the most recent announcement in #Announcements recommends that I should not use typescript verHow to get subcommand name from interaction object?I ~~am~~ will be having multiple commands which have their own sub commands (3 sub command per parenHow do I listen for reactions on all messages in a channel?doing discord bot dev after a long time. i wanted to listen for message reactions on every message iMessageCreate Event isn't workingThis is the first listener Im creating I've read the documentation 50 times imo and checked out the How big is the difference between xyz.cache.get() and await xzy.fetch()?fetch returns the cached thing aswell if it exists, so except the Promise difference i see not reallNOT WORK SAPPHIREwhy not work bot commands?Throwing on unhandled interactionHey, I just wanted to quickly check this idea - If I assign a listener to interaction create and theReply During CooldownCan I make the bot reply with the remaining time of cooldown delay ?messageUpdate event not Triggering```ts
import { Listener } from '@sapphire/framework';
import type { Message } from 'discord.js';
exp