❔ Use `this` inside method decorator
Tried to follow https://stackoverflow.com/a/2966758
Can't figure out how to access
this
using method decoratorStack Overflow
Can I use the decorator pattern to wrap a method body?
I have a bunch of methods with varying signatures. These methods interact with a fragile data connection, so we often use a helper class to perform retries/reconnects, etc. Like so:
MyHelper.
23 Replies
side note I was like "how hard can it be I will just factor out the simple side effect code into a decorator and have it up front"
oh how naive
@nekodjin ^u^
lol
oh damn you too huh
yeah I actually thought decorators of all thing would be a small cost abstraction to make my code a little prettier
I'm f*cking stupid
sigh
but alas, I still want my pretty
[Effect]
boxes so, here we areC# doesn't have decorators
Attributes are just metadata
For some other code to find and do something with
They're not like C++ macros or anything of the sort
but you can sandwich the original method in extra stuffs right?
no..
if so it'd be a decorator :p
Only in a very roundabout way. The attribute can contain a
Pre
and Post
methods, then you can get a method with this attribute using reflections, and execute Pre()
then ActualMethod()
then Post()
Attributes themselves don't ever execute
Something has to execute themYou can, of course, do something like
and call it with
mmm
I did an inefficient but it makes my brain happy
the long and short of it, vi, is give up the decorator idea
if i know anything about what you might be trying to use it for lol
>.<
yeah, sigh no actually interesting decorators
https://github.com/Fody/MethodDecorator
shenanigans with Fody are an option if you really want decorators
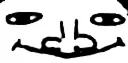
oh no..
don't encourage her 😭
she'll end up trying to make monads with a do notation decorator or smth
o.o
OwO
algebraic effects in C#?
>.>
aaaaaaaaaaaaa
listen
I autismo
sigh
FP go brr
Was this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.