10 Replies
Is this only about the performance gain of not having to declare a new
List
, or is there something else im missing?The yield version doesn't actually allocate a list, it only yields its items when the caller actually requests it.
The list has to allocate itself and a backing array.
If you're actually concerned about performance here, benchmark it.
I'm just thinking pragmatically from a beginners point of view, as in "is this knowledge that's useful, or academic fluff"
yield is definitely useful
for instance, it can be used to construct infinite sequences
The usecase is flying well above my head sadly. Can you give some real world examples?
sure, one sec
For a simple example, this code generates an infinite sequence of factorial numbers.
This would be rather impractical to do with lists, if you just want a sequence of numbers
You can do almost everything can do with
yield
with lists, but the benefit is that yield
is lazy and only evaluates when the caller wants it. In the example above, the 10! isn't computed unless the caller actually wants that.
(and yes the example above isn't actually very practical unless you really want a sequence of factorial numbers for whatever reason)benchmark gone wrong lmao
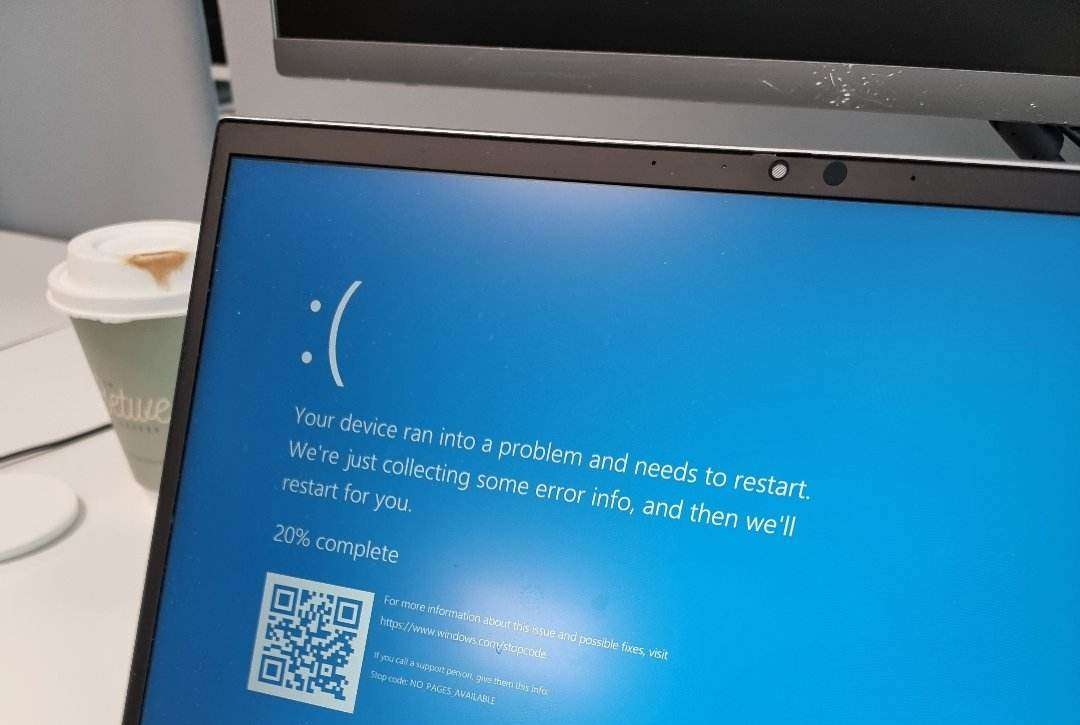
Another example would be if you have a tree structure This descends through a node's descendants, but only to the depth the caller wants. The tree might be recursive, having a node which links back to a previous node, which would for a list be impossible to handle, but for an enumerable using
yield
is perfectly fine.
Again the benefit is not having to compute the entire sequence unless it's actually needed.
Also here's some benchmarks
So you can see that the list allocates significantly more memory, because it has to allocate the entire list, while the enumerable only has to compute as much as it actually needs.
However the list is significantly faster (O(1)
) in terms of access, while the enumerable is linear (O(n)
).Yup I actually did some tests. Thoroughly shocked at how big the difference was!
If you allocate the list to fit 10K items from the start, you'll get a lot better memory numbers.. but it will still be much higher than the yield 🙂