Recurring Task
Is there anyway i can get my bot to run a task every 10 seconds or so to check if a giveaway is over by querying my mongodb
15 Replies
i know how to check if a giveaway has ended or not and how to handle that
but i have no idea how to implement a recurring task in my bot cleanly
Install, register, use @sapphire/plugin-scheduled-tasks
We don't have guide pages yet but various bots use if such as @Dragonite
Discord bots that use @sapphire/framework v4
- Official Bot Examples ᴱ ᴰ ᴶˢ
- Gemboard ᴱ ᴰ
- Dragonite ᴱ ᴰ
- Radon ᴱ ᴬ
- Sapphire Application Commands Examples ᴱ
- Archangel ᴱ ᴰ
- Zeyr ᴰ ᴬ
Discord bots that use @sapphire/framework v3
- Arima ᴱ
- Nino ᴱ ᴰ
- Operator ᴱ ᴬ ᴰ
- Spectera ᴬ
Discord bots that use @sapphire/framework v2
- Materia ᴱ
- RTByte ᴱ ᴬ
- Skyra ᴬ ᴰ
- YliasDiscordBot ᴬ
ᴱ: Uses ESM (if not specified then uses CJS)
ᴬ: Advanced bot (if not specified it is a simple bot, or not graded)
ᴰ: Uses Docker in production
ᴶˢ: Written in JavaScript. If not specified then the bot is written in TypeScript.
i wouldve used it myself
but i never got them to fire
Works just fine for dragonite and I know others use it as well
it could’ve been my db
or smth else
but I just use listeners
is there anyway i can use it without using a redis db
and maybe a mongo db
Not with this plugin but you can come up with your own system.
Mongo however unlike Redis doesn't have key expiry systems which Bullmq relies on
Also regarding having no idea, we live in 2023 yaknow:
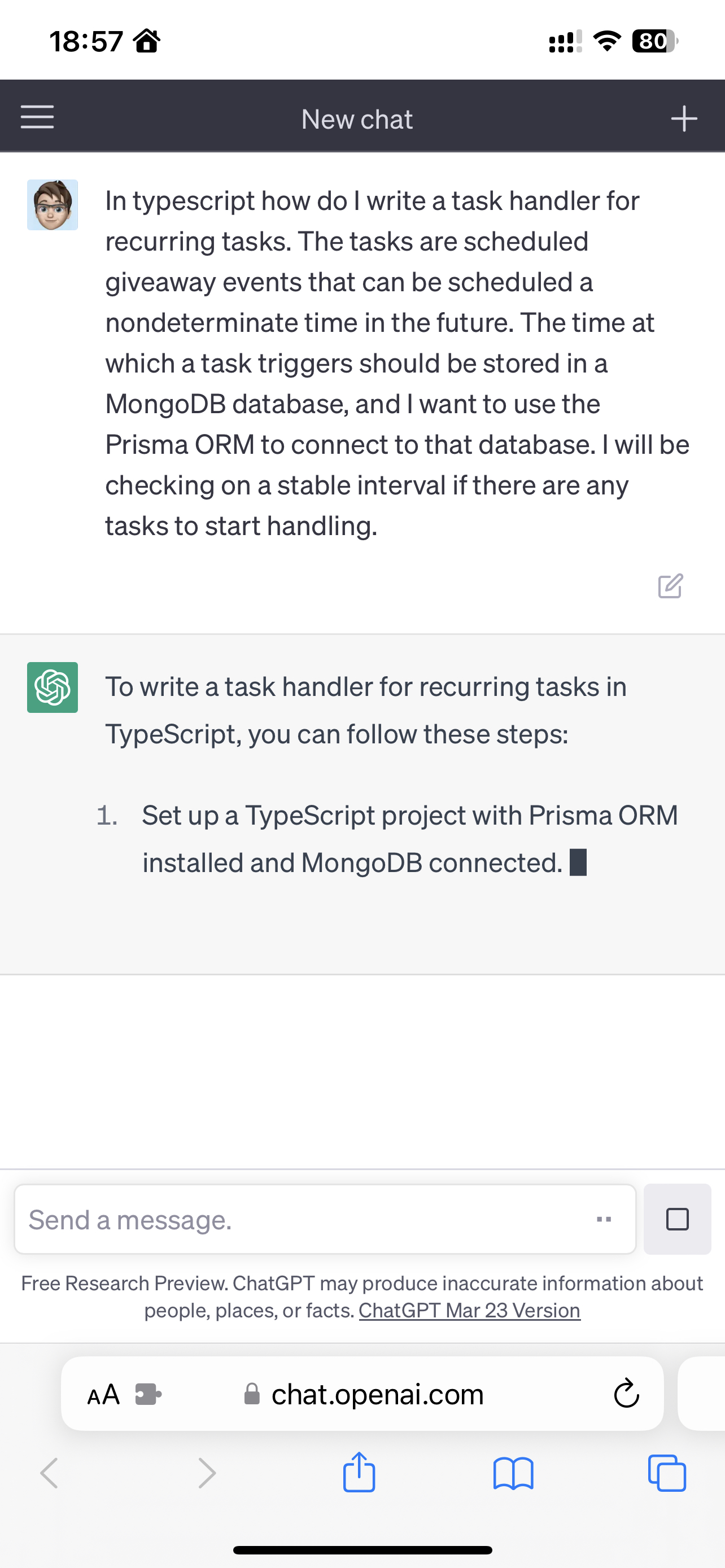
To write a task handler for recurring tasks in TypeScript, you can follow these steps:
1. Install the necessary dependencies:
-
You can install these dependencies using npm or yarn:
2. Create a
node-schedule
: to schedule recurring tasks.
- @prisma/client
: to connect to your MongoDB database using the Prisma ORM.
You can install these dependencies using npm or yarn:
2. Create a
Task
model using the Prisma schema language. This model will represent your scheduled giveaway events. You can define this model in a file called schema.prisma
:
3. Generate the Prisma client by running npx prisma generate
or yarn prisma generate
. This will create the PrismaClient
class that you can use to interact with your MongoDB database.
4. Write the task handler function that will handle your scheduled events. This function will use the PrismaClient
to retrieve the scheduled events from the database and perform the necessary actions. You can define this function in a file called task-handler.ts
:
In this function, we first retrieve all tasks from the database using prisma.task.findMany()
. We then loop through each task, check if its date is before or equal to the current time, and perform the necessary actions for the task. Finally, we delete the task from the database using prisma.task.delete()
.
5. Run the task handler using node task-handler.js
. This will start the task handler and schedule it to run every minute.
That's it! You now have a task handler for recurring tasks that uses MongoDB as a database and the Prisma ORM to interact with that database.
That's the response it generated
At a glance it covers all the major thingsAlright thanks
ill try and make my own system based on this information
ew mongo
do you need to use specifically mongo
Not my choice
whats wrong with mongo, what database do you recommend
psql is better
atleast imo