suspense + store
if I have a form tied to a store that gets populated async, how do i use this with <suspense so i don't need loading state management
22 Replies
resource + store storage
https://github.com/solidjs/solid/releases/tag/v1.5.0
the deep signal was added to solid-primitives too:
https://primitives.solidjs.community/package/resource#createdeepsignal
Solid Primitives
A library of high-quality primitives that extend SolidJS reactivity
any simple example for this?
is this correct?
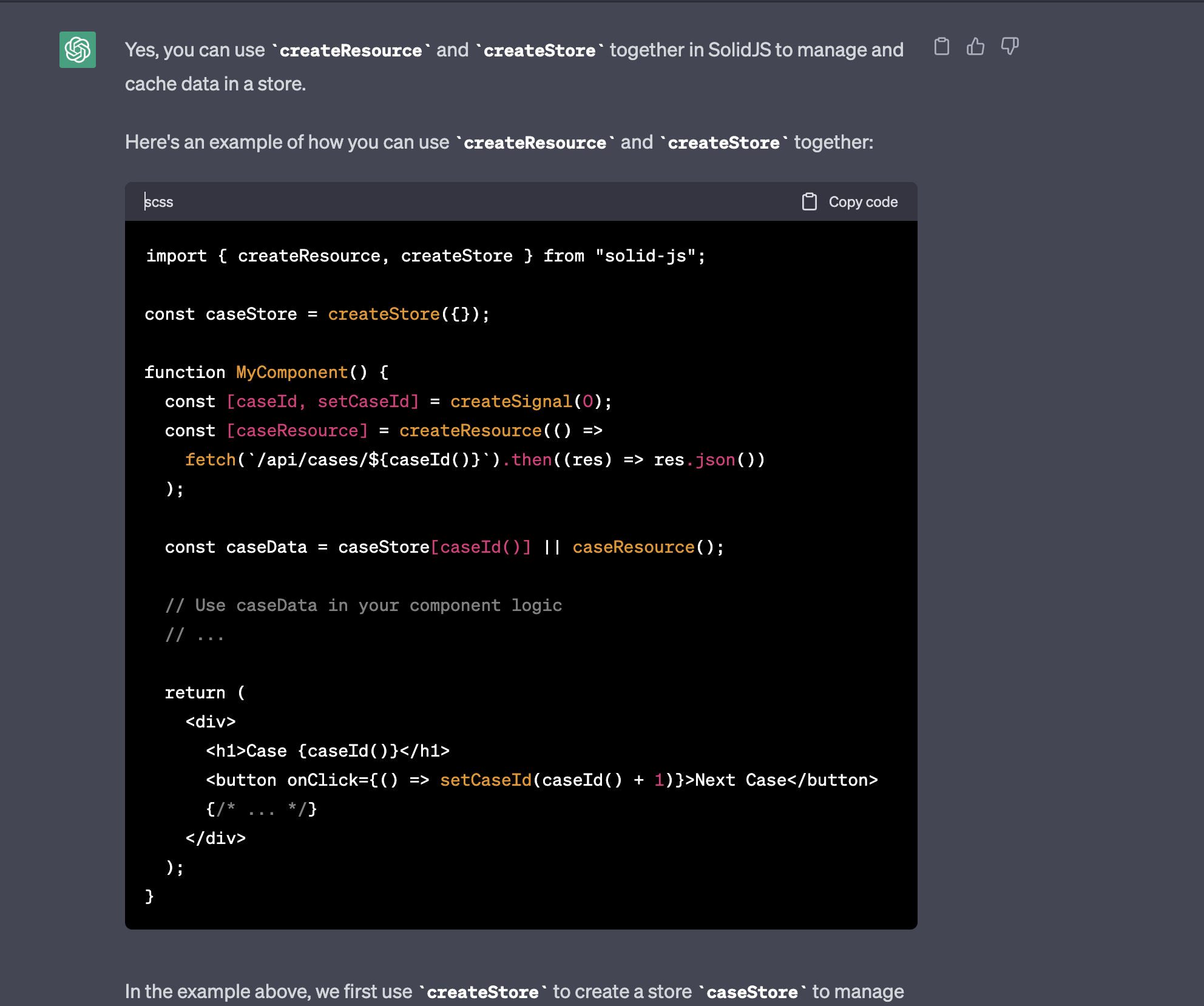
👆
i just want the simplest example possible, can't find exact what I want in the tutorial
this works, but its just a signal that returns I think, so i just need to update companyDetailsResource with the deep properties in the form
before I used resource and <suspend> i had loading management and
onChange={(e) => setCompanyDetailsStore('country', e.currentTarget.value)}>
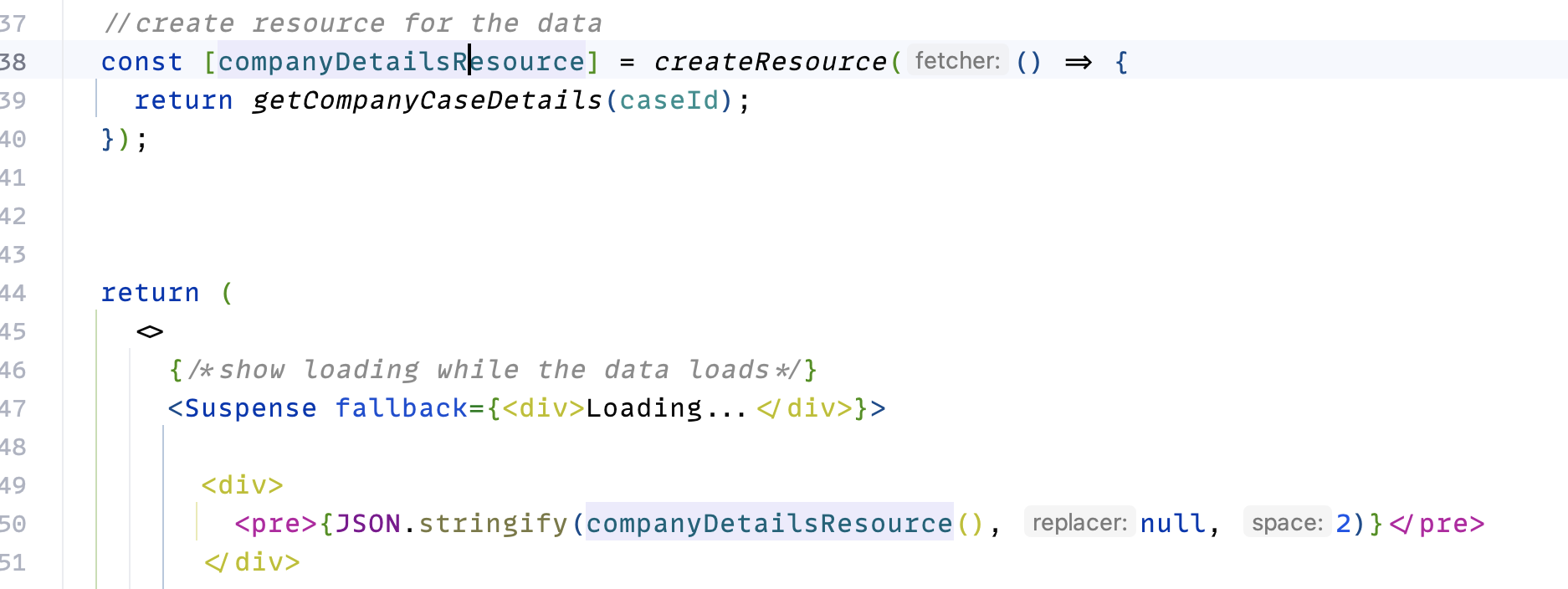
this release introduced
storage
option in createResource
, also has an example integrating createStore
=> createDeepSignal
+ storage
which makes res()
=> store
, { mutate }
=> setStore
(with reconcile
)
and thetarnav added that createDeepSignal
(i guess) to solid primitives so you can just grab it from there, which also has a simple example integrating the two
but yeah, it could be improved with more usage example i guessthx, so the primative is sugar?
createDeepSignal is from solid.js?
a helper for your convenience crafted by a magician 🪄
nah, the release link includes the implementation
.
my devs gonna be confused no end lol
which is probably the same as the solid primitive one
cool
How do I update a store in this way? is there a setter?
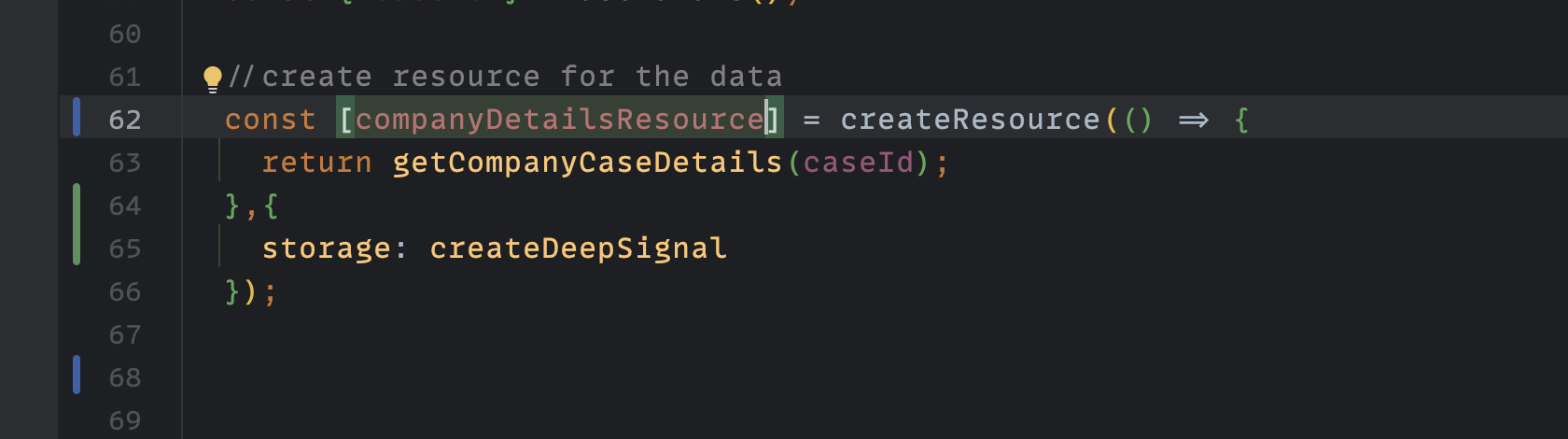
[data, { mutate }]
mutate is pretty much a signal setter
but since it's a "deep signal" it will reconcile on every set
there is probably a way to expose the path syntax and produce
too
but the typescript part is tricky
haven't looked into that yet
getting this error
will I guess I can set the data in a new clone of the resource, since the gql data response should not change
why can't we type the resource
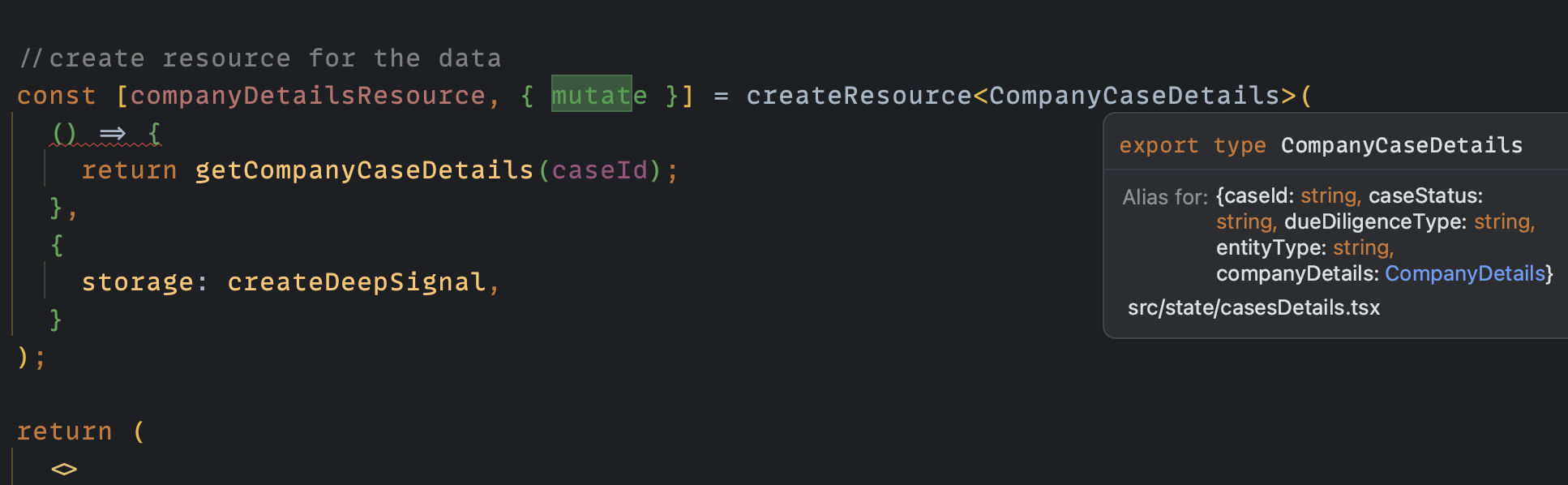
as I said
it's more like signal setter then store setter
if you see the source code for the deep signal youll see what's getting returned as a setter
ok
ah the type comes from the fetcher
what ill do is clone the resource result and tie that to the form
and mutate that
hmmm, donno
then i lose the suspense feature
i guess i will make the intital value for the form inputs equal to there resource and the cloned store used in the mutations
nah that aint gonna work, cause the value=
value={companyDetailsResource()?.companyDetails.registeredAddress.country}
im back to where i started lol
two simple stores without <suspense just seems easier to manage for me and my devs
at least i know how to use a read only resource tho, thx
i dont see
function createDeepSignal<T>(value: T): Signal<T> {
anywhere in source tho
in either repoyou could play with this too if you don't need to reconcile, but just use the resource as an initial state 🤷♂️
it's just createStore + reconcile
https://github.com/solidjs-community/solid-primitives/blob/main/packages/resource/src/index.ts#L256
i dont want to scrare off the react devs
too many layers of complexity here vs two stores
ill wrap the whole form in a <show with initial store>
and just have the 2nd store as the original comparison
I've decided to do this:
but calling
companyDetailsStore
throws error caught ReferenceError: companyDetailsStore is not defined
what should that <show
statement be like?