dynamic relational queries column returns
Hi I'm not entirely sure if this is the intended result when trying to make a relational query.
keep in mind that the only thing that doesn't work is the return type definition, the query works as intended meaning it grabs and returns the data that I want to return from the specified columns identified as true.
//type is derived from a mysql table
type User = {
id: string;
username: string;
email: string;
}
type UniqueQuery = 'id' | 'email' | 'username'
type UserColumns = { [p in keyof User]?: boolean }
const findOne = async(query: UniqueQuery, value: string, columns?: UserColumns ) => {
//The object that will appear will be an empty object like => { }
const user = await this.db.query.users.findFirst({
columns: columns
? columns
: {
id: true,
username: true,
email: true,
},
//method defined elsewhere
where: await validateQuery(query, value),
});
if (!user) throw new Error("User not found");
return user;
}
// if method is defined like this though the user's return object works as expected
async findOne<Columns extends { [Columns in keyof User]?: boolean }>(
query: UniqueQuery,
value: string,
columns?: Columns,
) {
const user = await this.db.query.users.findFirst({
columns: columns
? columns
: {
id: true,
username: true,
email: true,
},
where: await validateQuery(query, value),
});
if (!user) throw new Error("User not found");
return user;
}
//example using the method using generics
const user = await findOne("id", "asdf", {
id: true,
username: true,
});
//return object will look like this { id: /* some string */, username: /* some username */ }
// using method without generics using same values { }
//type is derived from a mysql table
type User = {
id: string;
username: string;
email: string;
}
type UniqueQuery = 'id' | 'email' | 'username'
type UserColumns = { [p in keyof User]?: boolean }
const findOne = async(query: UniqueQuery, value: string, columns?: UserColumns ) => {
//The object that will appear will be an empty object like => { }
const user = await this.db.query.users.findFirst({
columns: columns
? columns
: {
id: true,
username: true,
email: true,
},
//method defined elsewhere
where: await validateQuery(query, value),
});
if (!user) throw new Error("User not found");
return user;
}
// if method is defined like this though the user's return object works as expected
async findOne<Columns extends { [Columns in keyof User]?: boolean }>(
query: UniqueQuery,
value: string,
columns?: Columns,
) {
const user = await this.db.query.users.findFirst({
columns: columns
? columns
: {
id: true,
username: true,
email: true,
},
where: await validateQuery(query, value),
});
if (!user) throw new Error("User not found");
return user;
}
//example using the method using generics
const user = await findOne("id", "asdf", {
id: true,
username: true,
});
//return object will look like this { id: /* some string */, username: /* some username */ }
// using method without generics using same values { }
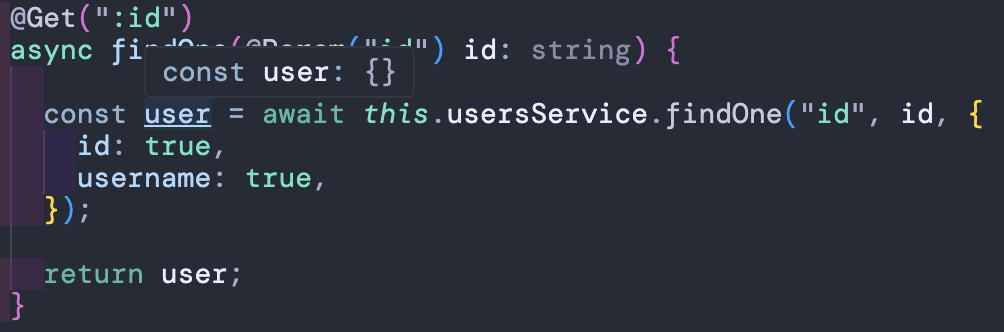
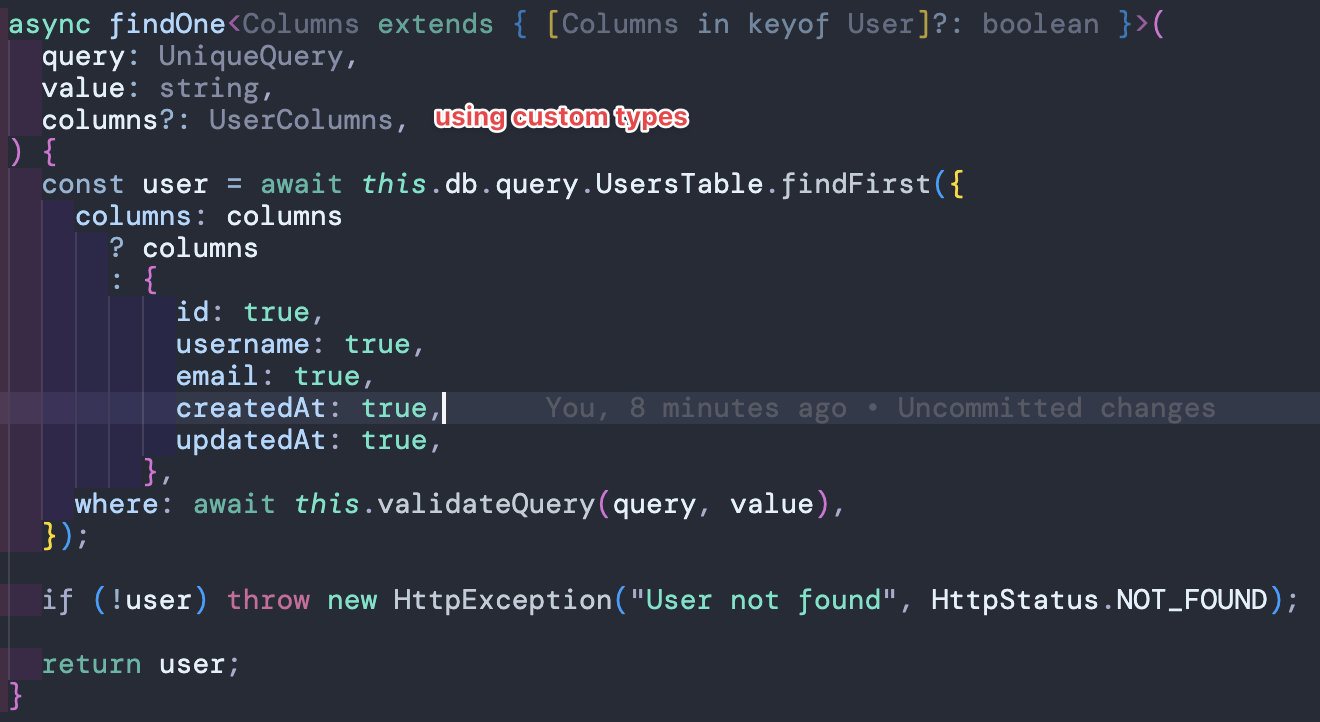
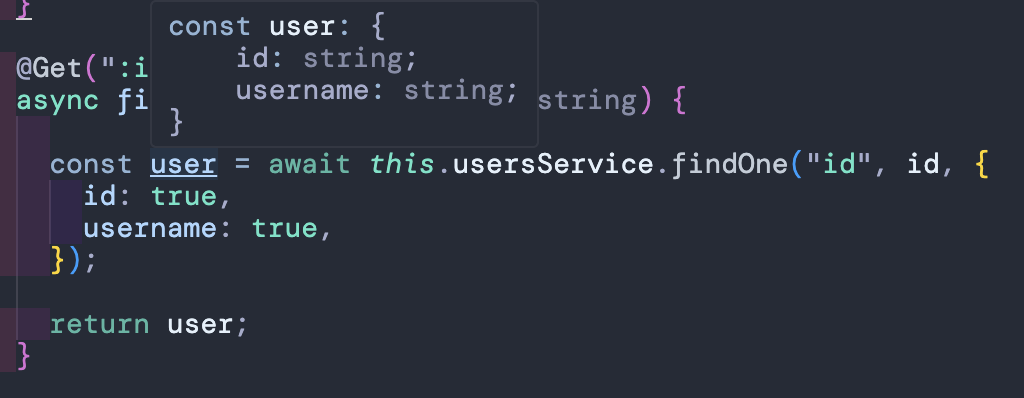
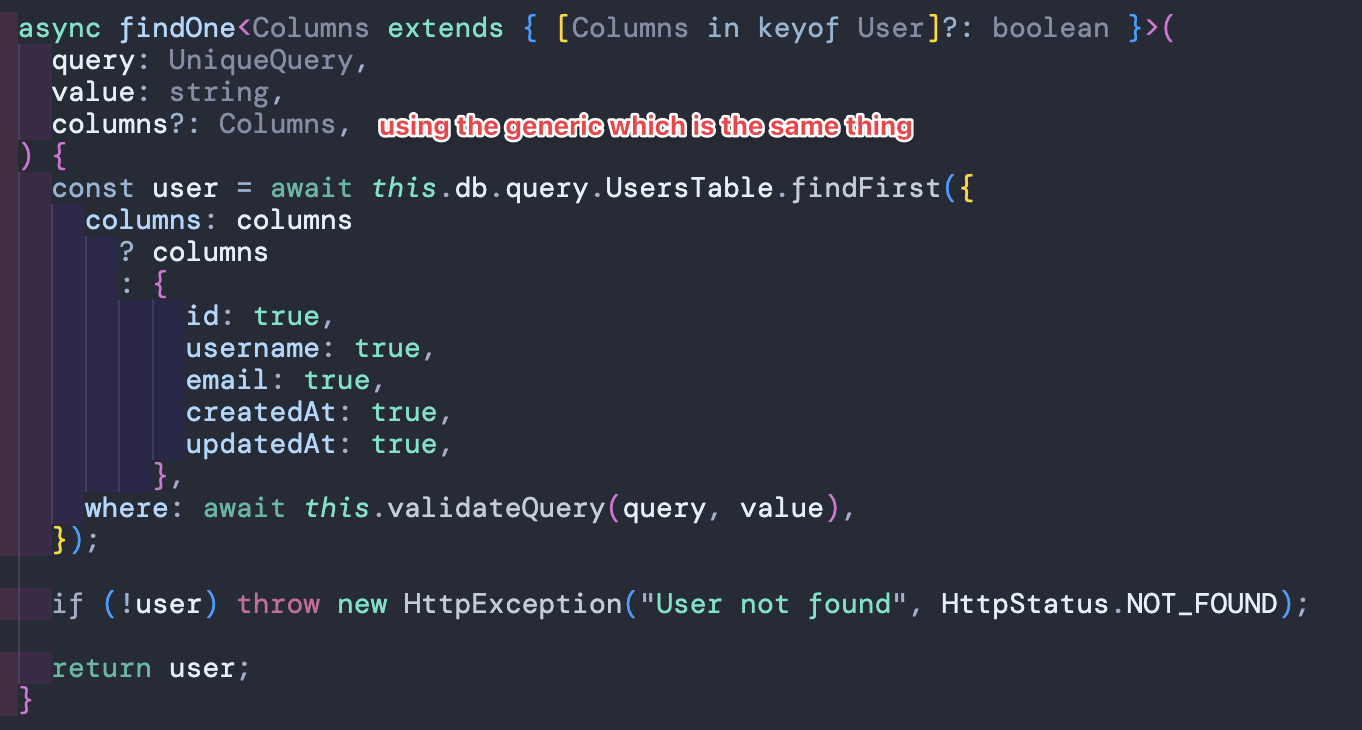
0 Replies