Modal Validation Error
const { InteractionHandler, InteractionHandlerTypes } = require('@sapphire/framework')
const { ModalBuilder, TextInputStyle, TextInputBuilder, ActionRowBuilder } = require('discord.js')
module.exports = class extends InteractionHandler {
constructor (ctx) {
super(ctx,
{
interactionHandlerType: InteractionHandlerTypes.SelectMenu
}
)
}
async parse (interaction) {
if (interaction.customId !== 'department' && interaction.customId !== 'staff') return this.none()
return this.some()
}
async run (interaction, result) {
try {
const selected = interaction.values[0]
const modal = new ModalBuilder()
modal.setCustomId(`ticketSubmit_${selected}`)
if (selected === 'inquiry') {
const textInput = new TextInputBuilder()
textInput.setCustomId('inputOne')
textInput.setLabel('What is your question?')
textInput.setStyle(TextInputStyle.Paragraph)
const actionRow = new ActionRowBuilder().addComponents(textInput)
modal.addComponents(actionRow)
} else if (selected === 'report') {
const reportWho = new TextInputBuilder()
reportWho.setCustomId('inputOne')
reportWho.setLabel('Who are you reporting')
reportWho.setStyle(TextInputStyle.Short)
const textInput = new TextInputBuilder()
textInput.setCustomId('inputTwo')
textInput.setLabel('Why are you reporting this individual?')
textInput.setStyle(TextInputStyle.Paragraph)
const actionRow = new ActionRowBuilder().addComponents(reportWho)
const secondActionRow = new ActionRowBuilder().addComponents(textInput)
modal.addComponents(actionRow, secondActionRow)
}
await interaction.showModal(modal)
} catch (err) {
console.error(err)
}
}
}
const { InteractionHandler, InteractionHandlerTypes } = require('@sapphire/framework')
const { ModalBuilder, TextInputStyle, TextInputBuilder, ActionRowBuilder } = require('discord.js')
module.exports = class extends InteractionHandler {
constructor (ctx) {
super(ctx,
{
interactionHandlerType: InteractionHandlerTypes.SelectMenu
}
)
}
async parse (interaction) {
if (interaction.customId !== 'department' && interaction.customId !== 'staff') return this.none()
return this.some()
}
async run (interaction, result) {
try {
const selected = interaction.values[0]
const modal = new ModalBuilder()
modal.setCustomId(`ticketSubmit_${selected}`)
if (selected === 'inquiry') {
const textInput = new TextInputBuilder()
textInput.setCustomId('inputOne')
textInput.setLabel('What is your question?')
textInput.setStyle(TextInputStyle.Paragraph)
const actionRow = new ActionRowBuilder().addComponents(textInput)
modal.addComponents(actionRow)
} else if (selected === 'report') {
const reportWho = new TextInputBuilder()
reportWho.setCustomId('inputOne')
reportWho.setLabel('Who are you reporting')
reportWho.setStyle(TextInputStyle.Short)
const textInput = new TextInputBuilder()
textInput.setCustomId('inputTwo')
textInput.setLabel('Why are you reporting this individual?')
textInput.setStyle(TextInputStyle.Paragraph)
const actionRow = new ActionRowBuilder().addComponents(reportWho)
const secondActionRow = new ActionRowBuilder().addComponents(textInput)
modal.addComponents(actionRow, secondActionRow)
}
await interaction.showModal(modal)
} catch (err) {
console.error(err)
}
}
}
Solution:Jump to solution
FYI, you can chain builder functions. For example, you can turn:
```ts
const textInput = new TextInputBuilder()
textInput.setCustomId('inputOne')...
5 Replies
@.theedeer Your modal needs a title. You can set it via
modal.setTitle('Ticket Submission Form')
.Solution
FYI, you can chain builder functions. For example, you can turn:
into
const textInput = new TextInputBuilder()
textInput.setCustomId('inputOne')
textInput.setLabel('What is your question?')
textInput.setStyle(TextInputStyle.Paragraph)
const textInput = new TextInputBuilder()
textInput.setCustomId('inputOne')
textInput.setLabel('What is your question?')
textInput.setStyle(TextInputStyle.Paragraph)
const textInput = new TextInputBuilder()
.setCustomId('inputOne')
.setLabel('What is your question?')
.setStyle(TextInputStyle.Paragraph)
const textInput = new TextInputBuilder()
.setCustomId('inputOne')
.setLabel('What is your question?')
.setStyle(TextInputStyle.Paragraph)
Nice try favvy wavvy but that wasn't the solution message 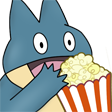
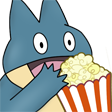
I thought it was one and the same message. Oh well.
How dare you