Importing drizzle-zod Schemas on the Client
Hi,
Is it ok to import schemas generated with
drizzle-zod
via createInsertSchema
on the client? I have my schema in a separate schema.ts
file away from where I init my database if that matters at all.
Thanks!13 Replies
Maybe someone has a better solution... but here it goes.
Personally, I wouldn't do that because
- It essentially means that the client will have to import all the
- this includes drizzle and afaik your db schema. (Maybe that will be better once "use server" and "use client" are smarter?
drizzle-zod
dependencies- this includes drizzle and afaik your db schema. (Maybe that will be better once "use server" and "use client" are smarter?
)
- Bundle size increases for the client.
- TypeScript performance decreases significantly - since it needs to infer both, zod and the db schema.
After a small project with many entities, I found that drizzle-zod isn't that helpful to build client-side forms or API inputs with. Mainly, because of the reasons above and because I almost always had to refine every single field besides
id or a
createdAt anyway. (adding email, length or other constraints).
So what I'm doing:
Create an extra zod schema in e.g.
entities/user/schema.ts
```ts
const SOME_CONSTANT = "foo";
export const userSchema = z.object({...});
export type User = z.infer<typeof userSchema>;
```
Create a db schema in e.g.
entities/user/table.ts
```ts
// Can easily use SOME_CONSTANT
export const usersTable = pgTable(...);
// Add refinements/transformations for certain db fields. E.g. JSON fields.
export const usersTableInsertSchema = createInsertSchema(usersTable);
export type UsersTableInsert = z.infer<typeof usersTableInsertSchema>;
```
This way, client modules with e.g.
react-hook-form code can simply import
entities/user/schema.ts` without having the baggage of drizzle.
The backend API handlers (I'm using tRPC) can import both. The schemas for the form/client and the db insert schema.
This means you can still make transformations/parsing checks e.g. for JSON fields right before the insert - but without bothering the client with it.
Let me know if that works for you.I'm using Wundergraph which introspects my DB (using prisma under the hood i think). it produces a ton of types for the graphQL API it uses
So do you think i'll just have to manually write up the zod schemas manually based on the generated types? the generate file is huge so wondering if there might be a better aproach. Each of those types has its own nested input type which has nested properties, so not sure if i should go very detaild and also create zod schemas on the nested input types..
Seems like i dont have to make zod schemas on the drizzle schema however.
Also using
satisfies z.ZodType<...>
seems to capture any errors i am missing required properties, but doesnt include optional fields or the nested types. so i am a little concerned with bugs where an optional field is used and errors out from being missed in the zod schema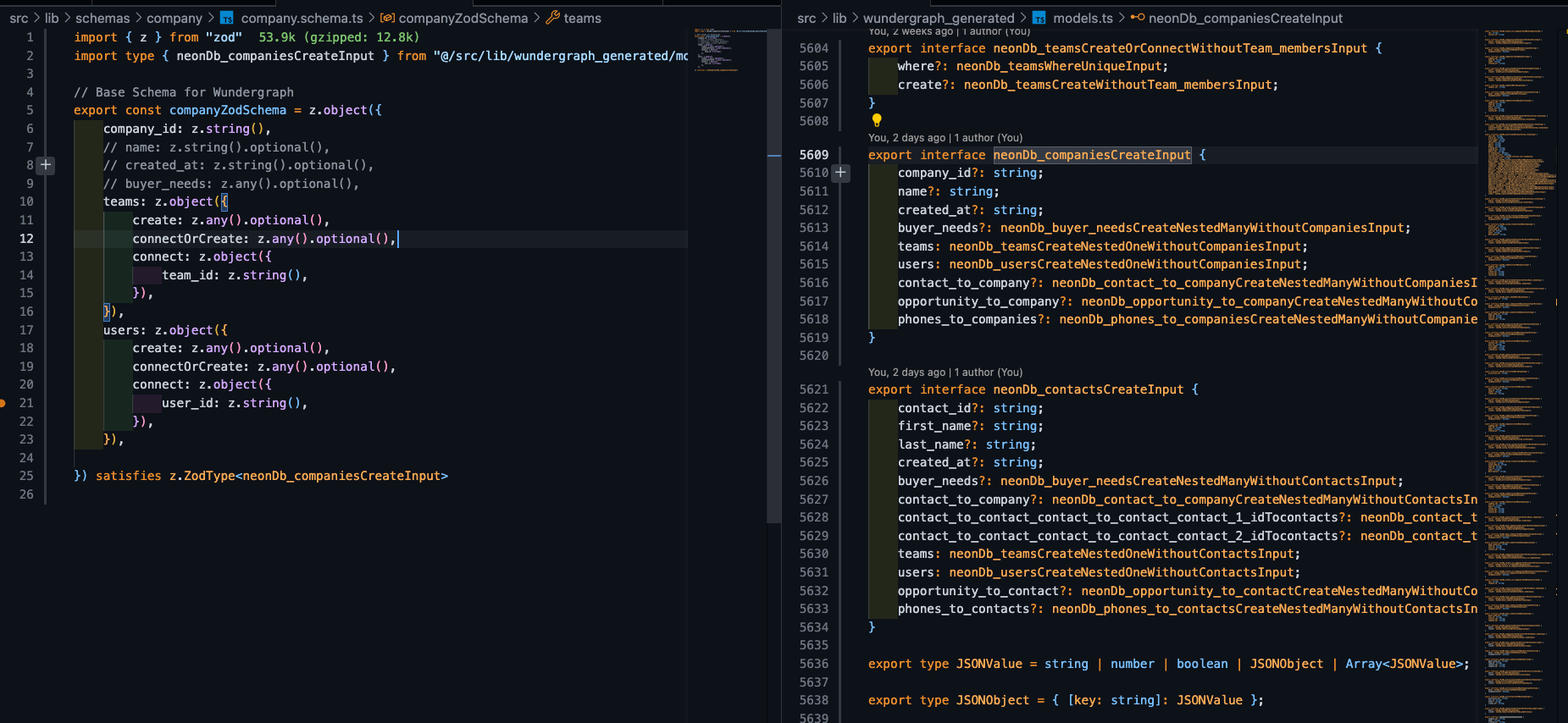
Okay. So you want zod schemas for validation of graphql api calls?
In that case, I think you are better off generating the zod schemas with a library from here https://zod.dev/?id=x-to-zod
Based on your use case, I think
ts-to-zod
will fit. But there's also a GraphQL codegen one.
Oh, just noticed you're not OP. This reply is for you @diamond.dragonyeah i been playing around with the graphQl codegen but am getting issues getting it to run with graphql dependency issues. and ts-to-zod wasnt able to parse the generated ts file >.>
Have you seen this? https://github.com/fabien0102/ts-to-zod#limitation
Maybe you can adjust Wundergraph's output to match the limitations. Also, I suggest trying it on a small part of the file first and see if it works.
https://wundergraph.com/blog/a_comprehensive_guide_to_wundergraph#usequery
it looks like Wundergraph already has some validation. Maybe zod is not even needed...?
yeah the issue i have found is the enum's generated produce
Error: Unknown IndexedAccessTypeNode.objectType type
which i saw there is an issue outstanding about this in ts-to-zod.
I beleive you still have to define the zod schema in order to pass the data to the API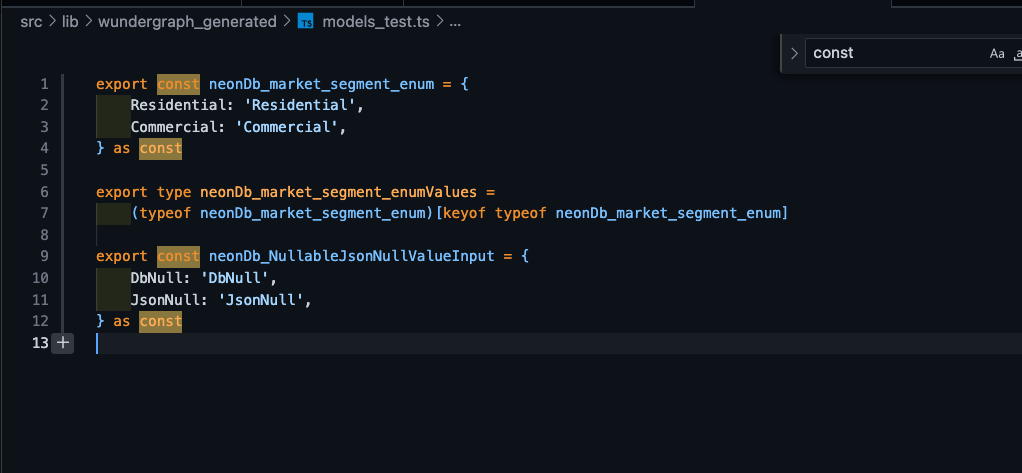
Yeah, seems not to be an easy fix.
Having said all that, I don't think it makes sense to create a schema only from the drizzle schema.
createInsertSchema
creates a schema to be used to validate DB inserts. This does not mean it's compatible to your API. Especially if you can create rather complex filters, etc. with GraphQL.Ah @michaelschufi its actually here where the ts-to-zod issue is
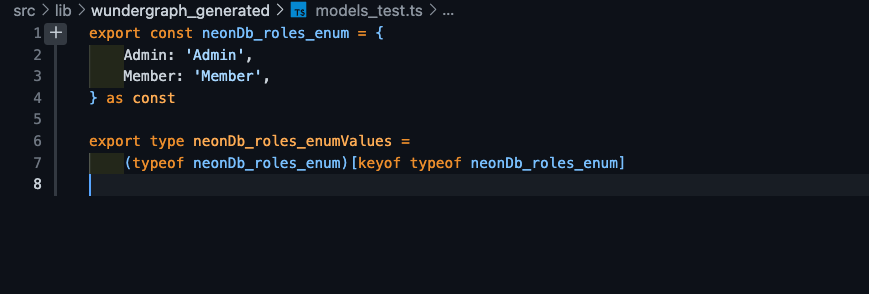
@lermatroid Sorry for hijacking this thread. It's a bummer, Discord doesn't allow threads in the Q&A chats.
i think what i may be able to do is use a utilty function to create the create/createMany/connectOrCreate objects and then assign the drizze utility that wraps all the "base fields" of a table.
I think then I can also add additional schema fields for all the related Input objects and merge them into the drizzle wrapped schema
i am going to have to rename all my drizzle field names to snake case tho to match whats generated from GQL schema
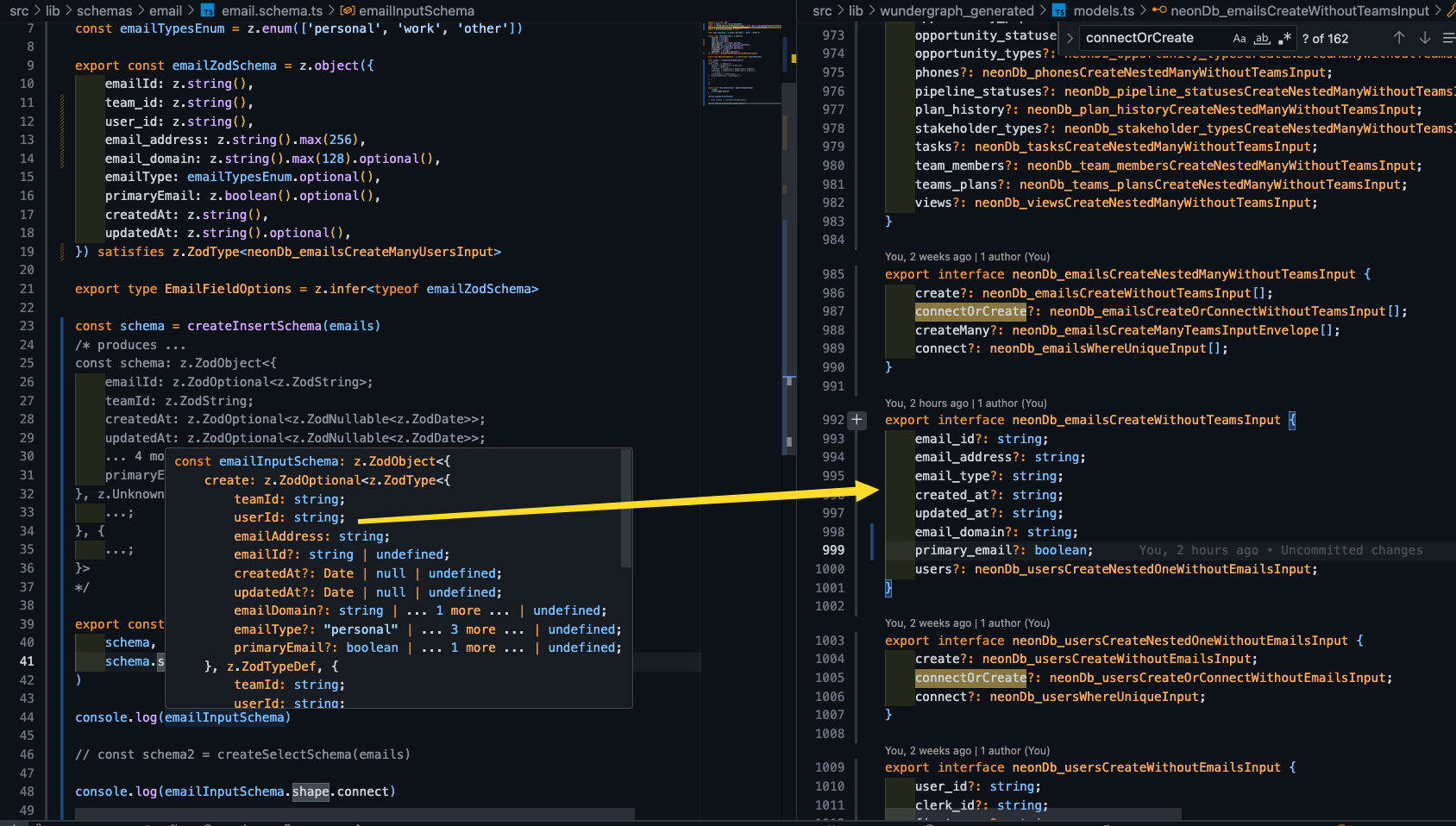
Hmm, I think we should be able to to this though... granted I'm not sure.
If you have a separate package for your db and then create a schema like this:
And then import it in the client the only thing that will end up there is a zod schema.
When you build it should output
userTableInsertSchema
as a zod schema and if you import it in the client it should be fine right?
This works if your database and schema are in a separate package and you first build that package and then it's used in other places like your backend or frontend.
I'm not really sure if this work so I'm going to try it this weekend.It seems like it unfortunately is in the client bundle. I've tried it with
export const usersTableInsertSchema = createInsertSchema(usersTable);
in a separate module. But since we are importing the table there, we also get e.g. drizzle-orm
in our bundle (see screenshot). But your table definitions are not in the client bundle afaik (searched .next
in vscode for a field name).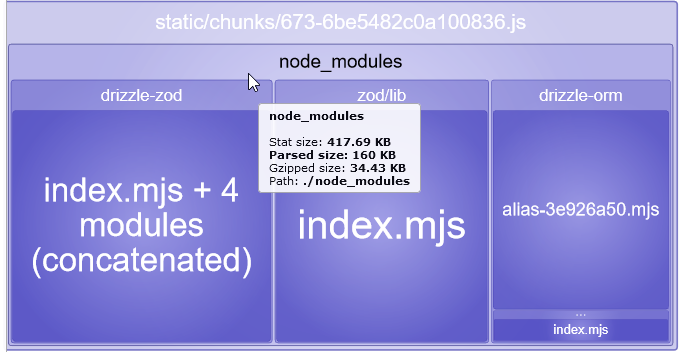
(zod/lib is 12 KB - so about 22 KB is drizzle-zod + -orm)