How can I access a store from the parent context from inside of a nested context?
I am trying to access the WebsocketContext from inside of the DataStreamContext, in order to send out a websocket message when a DataStreamContext function is called. I can't seem to figure out the right way to do this.
Provider structure:
22 Replies
Seems like you need to compose this two contexts into single one
I can't seem to figure out the right way to do this.You can access context in the same way you do in the routes. If it is below the provider it will be available, you just need a reference to WebsocketCtx
Seems he is asking how to access DataStream Context from Websocket Context, that is reverse order
I am trying to access the WebsocketContext from inside of the DataStreamContextπ€ if itβs the other way around then just reverse them π€·ββοΈ
It's circular dependencies probably
I am trying to access the websocket from inside the datastream
it builds on top of it
Got it, so I just need to add a useContext inside of that context?
so instead of my incorrect
props.state.socket.emit()
here, I could access it via useContext()
right?Yes
Ok, let me give that a shot.
Hmmmm
websocket
is undefined here inside of calling addStationToMonitor()
and it doesn't seem to make sense to put the context into the state for this other context.you probably want
no i return two items
since your websocket state is in the first index
yeah
yes, but if you want to access
socket.emit
, that's in your state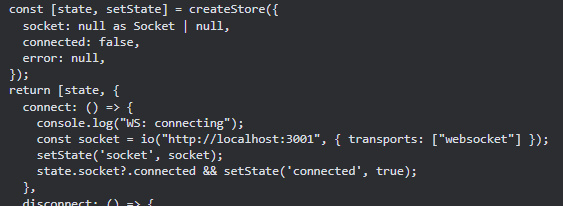
i have it in websocket (second item)
ohh
right, the second item is the functions
Fantastic, that worked
nice
One more issue.
Trying to call
socket.on()
from inside of the DataStreamContext, but of course at time of initialization the socket does not yet exist. Is there some way to do this so that it can register those once the socket exists?you could use a createEffect
if you only want it to run once the socket is available you can have the initialized variable like I used there
oooooooh
elegant
let me give it a shot
It works! Incredible.
nice!
This has really extracted out some functionality nicely. I'm impressed.
Looking sharp. Thanks for the help.
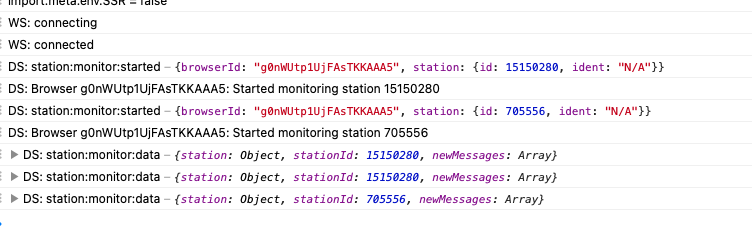