Default values in props with Merge Giving me a weird interface
In the situation where I want to possibly pass in prop, say
arrOfStrings
:
I'm getting a resulting type for merged
as :
How can I resolve this in Typescript?6 Replies
hm it seems that typescript doesn't know that in this case, the merged props will 100% have a arrayOfStrings property
I don't know if that could be fixed by solid.js. This would be a workaround for you:
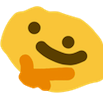
The problem is this is a simplified version, so I could have final props but I'm trying to avoid duplicating a large interface :/ Thank you for this answer though
oh it's because of the empty array. This also solves the problem:
very confusing why typescript thinks the property can be undefined if you provide a default empty array
ah yes, that did it! thank you
glad that I could help!
I asked in the #typescript channel about it (https://discord.com/channels/722131463138705510/783844647528955931/1143634436240461824)
This looks like it could be a mistake on solid's side. I will open an issue if this would be the case.
awesome I'll track it there!