❔ Code only firing when using breakpoints in Visual Studio
I am having an issue with my code for a Twitch bot(Using TwitchLIB API)
Certain lines of code will work just fine, but others will only work when using a breakpoint inside of their methods, and i'm unsure why this is working this way. The point of breakpoints is so that I can see what lines of my code are being fired, and where issues are, but how am I supposed to troubleshoot if the code works when I use the breakpoints? I've used a logging method to verify, and the code is REACHED just fine without the breakpoints. Any ideas? I'll include some of my code in a reply to this thread.
20 Replies
I have a line of code that fired when the bot joins a twitch channel, that sends perfectly fine. Nothing is setup differently that I can see(code below sends the message just fine)
But then for my command and welcome message functionality, the client.SendMessage only actually sends a message when I am using breakpoints inside of the method that they are in. Below is my logic for welcome messages
form1.TwitchLog is my logging functionality into a textbox on my form, and client is a public TwitchClient variable that is initialized in the below method when I click a button
Generally, if some code works in debug but not otherwise, it's because something
async
doesn't get await
edHmm I only have two methods that are async, and they are both awaited(neither of them are the affected methods). I'm assuming something within the API could be asynced and need awaited?
Possibly
Since debugging slows down the code, some of the asynchronous code has the time to resolve properly, even without
await
. That's why it's my first suspicionBut that also confuses me as to why it would work in one(OnJoinedChannel) but not the other (OnChatCommandReceived or OnMessageReceived)
Often happens when
async void
methods existBoth of my asyncs are tasks, I do have an async void method lower in the list, but it only gets called when I press a different button.
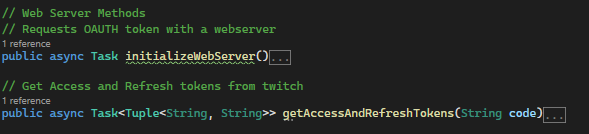
Huh, yeah,
async void
is unavoidable in GUI apps because event handlers cannot be asynchronous
Depending on the framework, there are various ways to deal with that, thoughYeah so I have async void in my form for all the button clicks and closing the form, and then I have an async void method for when my eventsub connects(it awaits a list of all users from a list I made)
But this isn't called until I press my second button so that shouldn't affect everything above.
The other possibility with debug mode, besides Z's points, is that maybe when you stop on a breakpoint, VS evaluates some property that causes a side effect, and your program is effectively depending on those side effects
In order to show the locals window, VS needs to evaluate properties and such to get their values
Hmm i'm not sure I follow
Your code has properties in it, yes?
Yeah ofc
Any that are not autoproperties?
That is, more than just
{ get; set; }
?I don't believe so no
I do have this class inside of a class, but I don't think that's what you mean
No, that's fine
Well, some questionable choices, but mostly stylistic, and nothing that'd cause the sort of issue you describe
Here are the variables I have set in this class, + 2 arrays and 2 dictionaries, but nothing else that looks to be out of the ordinary
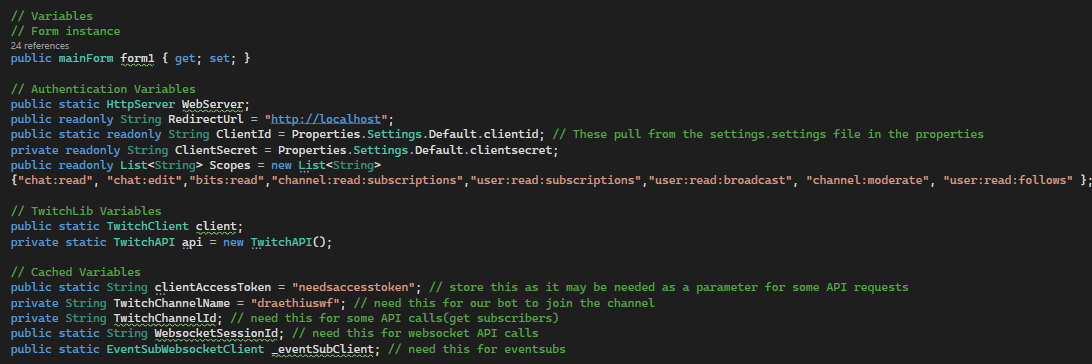
Was this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.This has not been resolved yet, unsure what is going on
Was this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.