❔ JSON serialization nullable warnings (best approach?)
Kind of have a "best approach" question for the following:
Would you make it nullable using
?
or ??
in the appropriate spots, or (in this particular case) since I know the value will never be null, would you do as I have and use !
to suppress the warning?7 Replies
It would only ever return 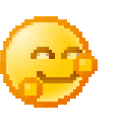
null
if the contents of the quacken.json
file were literally just
If you know that's never the case, I'd suppress it with !
A more proper way would likely be ?? new()
But that crosses the territory of inappropriately handling null inputs and hiding said handling away from whoever uses it
The most proper way would obviously be to make the return type nullable
But now you have some options and some 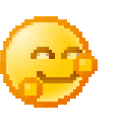
So the two good options of these three would be either what I did above or
I think this is what you meant by making the return type nullable, right?
Mhm
Awesome. Thank you for the insight. I was not aware of the
?? new()
hiding the handling away, so I'll just try to keep in mind to either suppress or make it into a nullable type(preferably the latter) ⭐if you really don't like
Deserialize<>()!
you can always do Deserialize<>() ?? throw new InvalidOperationException()
one could argue that's the most "proper", even if it's an exception that should never occur
as it fails earlyI would perhaps choose FormatException
Was this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.