❔ JWT Authentication: Name goes to claims not identity.Name
Following Patrick God tutorial for JWT Authentication and Authorization here:
Here's the class in question with dummy token:
Here's the razor HTML from the shared image:
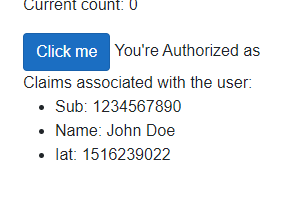
33 Replies
Everything is exactly the same as the video aside me checking claims in html, but his claim overwrites the
@context.User.Identity.Name
Thanks for any help given!uhhh...whose claim?
can we clarify the issue here?
context.User.Identity
is being overwritten? By what?the
context.User.Identity
is overwritten by var state = new AuthenticationState(user);
. Here user
holds a claim type Name
so I'm wondering why it's not overwriting the User.Identity.Name
as shown in the linked tutorial and instead making a new claim under context.User.Claims
.wait, this is all client-side auth?
yeah
mm-kay
I have basically no experience with the client-side auth framework
Oh well neither do I 🤣
I'm not really concerned with any particulars at this moment other than why the JWT isn't overriding the default claims or whatever you call them and instead adding claims
me, I'd probably start looking at the source, if there's really no official docs for it
idk what you mean
source code, I mean
oh the example code?
no
the source code
like the code I'm writing? I'm writing this. lol.
no
the source code
for the thing you're trying to figure out
the identity docs? oh okay lmao
if there are docs, sure
sorry I'm dense. Yeah there are.
otherwise, the source
gotcha sorry for being dense
no big
what library is this?
ClaimsPrincipal(IEnumerable<ClaimsIdentity>) Initializes a new instance of the ClaimsPrincipal class using the specified claims identities.this is aspnet identity
specifically
oh one sec
like I said, I'm not familiar with the client-side libs
for the identity claims creation it's
System.Security.Claims
and
Microsoft.AspNetCore.Components.Authorization
tooallllright
so, what is
context
here?
also, have you read this?ASP.NET Core Blazor authentication and authorization
Learn about Blazor authentication and authorization scenarios.
also this
Secure ASP.NET Core Blazor Server apps
Learn how to secure Blazor Server apps as ASP.NET Core applications.
Was this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.I'm actually not entirely sure. I'm guessing httpcontext which has information from authorization middleware. I have not read those in total but have referenced them when trying to figure this out. They don't show this particular process in detail, that us unpacking a JWT and applying to a claimsidentity.
I'm guessing httpcontextwell, don't guess, look and see the second link seems to explicitly demonstrate iplementing a custom state provider, where the claims come from is irrelevant although, at a glance, I don't see anything different than what you've shown me
Was this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.