❔ .NET split string that is in a list
I have this (possibly horrible) code that works
The output is a list of full path to a file. I would like to split/remove the
/README.md
from the results. is this possible?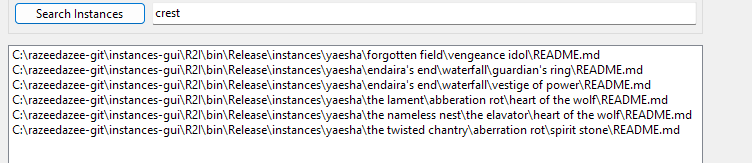
34 Replies
So... you want to remove the last item in a list?
Angius
REPL Result: Success
Result: string
Compile: 581.381ms | Execution: 77.383ms | React with ❌ to remove this embed.
that looks like the result, the
\README.md
so i just have path.?
You want just the path, without the file name?
Use
Path.DirectoryName()
then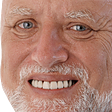
First thing I noticed lol
From an efficiency standpoint this also pains me, but I realize this question is probably not about optimizing performance.
i am a NET noob, i am pasting away till things work with a poor grasp
i need to the search the README.md files for a string and ideally list the parent dir.
Angius
Use
Path.DirectoryName()
thenQuoted by
<@85903769203642368> from #.NET split string that is in a list (click here)
React with ❌ to remove this embed.
Angius
REPL Result: Failure
Exception: CompilationErrorException
Compile: 336.801ms | Execution: 0.000ms | React with ❌ to remove this embed.
umm
Angius
REPL Result: Success
Result: string
Compile: 506.288ms | Execution: 34.826ms | React with ❌ to remove this embed.
There
If I actually had to do this I would probably shell out to ripgrep and parse the output (for performance reasons) but if you want to write it entirely in C# I would at least using a streaming reader instead of ReadAllText. Unless the set of files is always going to be so tiny it doesn't matter.
i am using windows forms with net 7 via Visual Studio.
the code optimisation thing is going to go over my head unless you ELI5 with examples.
Eh, don't worry about it then. Get it working first.
(For the record my approach would probably be something like this:
https://paste.mod.gg/rfgcrmjmvucq/0)
But again, don't optimize until you know you care about making it faster / use less memory.
BlazeBin - rfgcrmjmvucq
A tool for sharing your source code with the world!
this seems to have worked, in regards to function
cs
is the language marker for C#thanks

@mtreit thanks for the example. i will try understand it
Mainly it does two things for performance:
1. Enumerates the directories in parallel (which is why it uses a thread-safe collection to store the results)
2. Does not read the entire file into memory, but instead reads one line at a time.
Probably in your case neither is important but as a pattern it's useful to know if you ever need to process very large files, or very large numbers of files, or both.
it won't hurt to try and do it well. right now i'm just fumbling along with googles examples
Oh the lineNumber thing can be ignored, I was originally storing that in the result but changed it when I saw you just wanted the directory name. Originally I was storing the file path and the line number where the first match was found.
one thing i would like to know is how to make a function i reuse.
i know how to do it in bash scripting.
i have some repeated code i would function in bash but not sure how i do it in NET
i have a few buttons using same code except for different dirs
i should be able to make a function here?
Sure, making functions (methods) is a good practice.
If you are using Visual Studio there is an "extract method" thing you can use to highlight the repeated code and turn it into a method
thanks
Also, use
Path.Combine
to combine paths and filenames, instead of string concatenation.@mtreit when i look at your example, why does my visual studio shwo this error

i kind of understand the error, but i don't get what solution is
I wrote a stand-alone program that uses top-level statements - that code is inside a (compiler-generated) Main method that has a parameter named
args
, as most console programs do.
If you're writing a GUI program it won't have that.Was this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.