PocketBase Auth with Context doesn't track store
I'm trying to create a PocketBase AuthContext that I may add to my app, so that in the future if I wish to add more routes I can access the data from all the routes. However this is getting on my nerves, because the store isn't tracking when the pb.authStore changes (creation / deletion / updated), that is except when HMR kicks in, then it refreshes. And the weirdest part is I can log it on the console...
Here is the code:
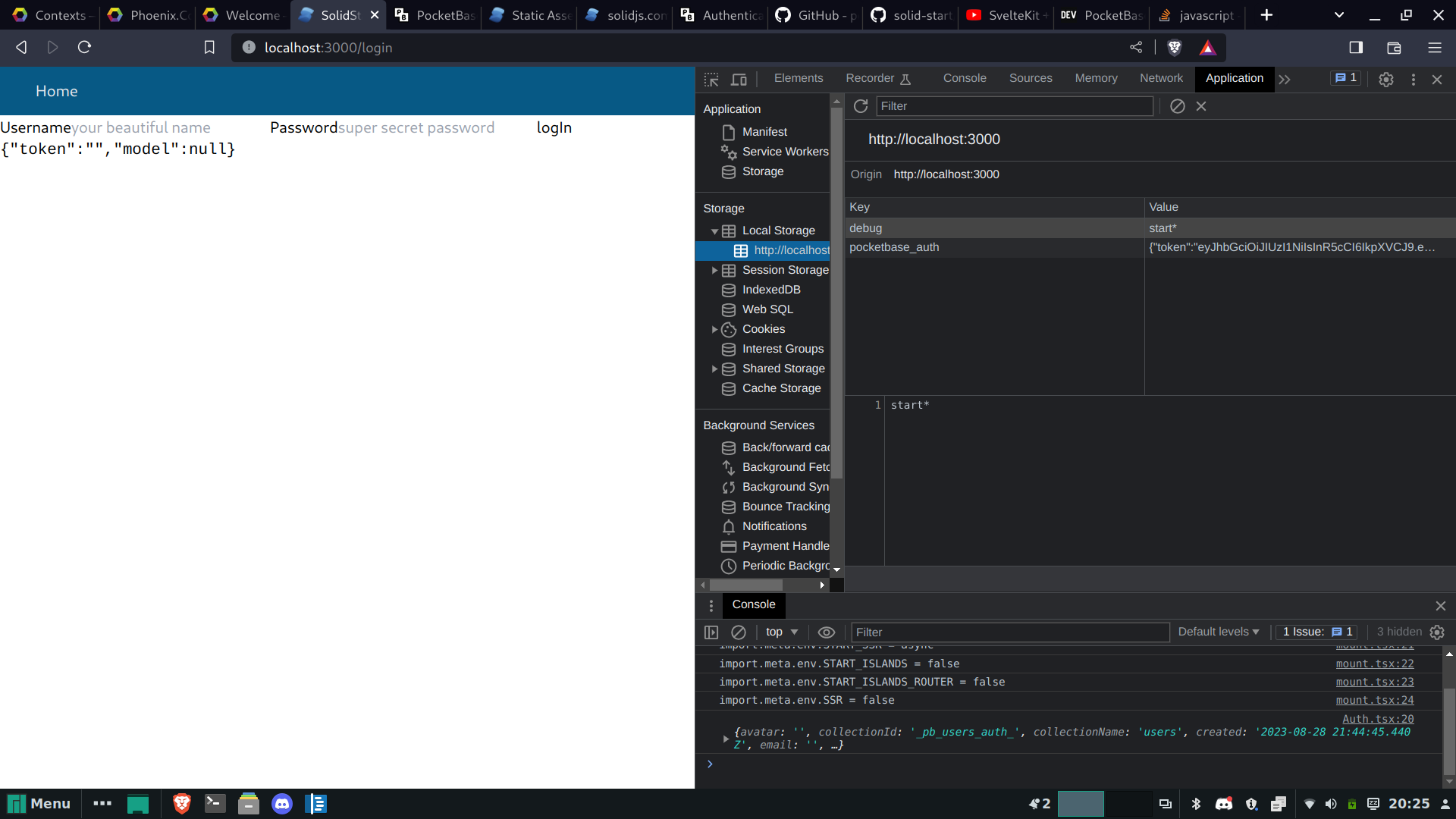
3 Replies
PS: I wrapped my Routes with the AuthProvider
1. you don't need
createMemo
, createEffect
here as pocketbase is external to solid and doesn't(just a hunch) use any solid primitives(signals, memo)
2. don't destructure store { token, model }
(also applies to useAuth
's return)
3. instead directly pass the state
(store) to context
4. also a good practice to unsub using onCleanup
5. reconcile
might be unnecessary if model
is a completely different object, doing setState({ model: mod, token: tok })
should replace the old one with the new one completelythanks for the help bruv it seems to working now !