Adding components that use context to the DOM after mount
I have a component (ShelfScene) that returns a mapped list of items (Shelf). The ShelfScene component appends a few Shelf components onMount and those render and use context fine with no issues. However when I try to append a new Shelf component after mount the new component is not able to read the context. Any Ideas?
10 Replies
Could you share some code? is hard to guess rn
You got it
here is the component that wraps context:
Here is ShelfScene:
<TestComponent /> and <Shelf /> are components that use context passed in the context wrap component. When I run newShelf() onclick with a generic div it mounts just fine but when I try to add one of the context using components. (shown above ) they do not mount and I get these errors:
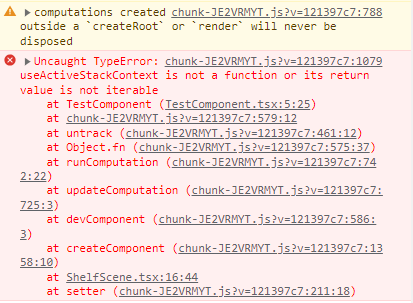
If its relevanant this is a multi page application and the I am using astro to handle the routing
newShelf
fm gets called in an event listener cb which, where context is not available
if you wrap component calls with a thunk, so it gets called lazily in jsx it should workcould you enlighten me on what a thunk is? sorry, relatively new to programming in general and solid. thanks!
() =>
so:
OK adding a return to the thunk does the trick:
Thank you much!
you should probably look into rendering them with For instead though
you’re at risk of rerendering components a lot of you just map over an array and render that
yes I was using for initially I switched to the map during the debugging process. Thanks for the advice!