C# help with detecting key press
Hello, I'm a beginner to c# and I'm currently doing this practice exercise.
The exercise requires me to code out a character falling from the top of the compiler window to the bottom. This task will run until the user press the Escape key to then quit the task.
So I know how to make the character fall from the top of the screen to the bottom, but I'm not sure how I can make it do that asynchronously while waiting for a key press to quit.
I have searched online for a way to detect a keypress while executing a loop and found this KeyAvailable property that I can use. However, as to my understand, the KeyAvailable property only returns true when a key is pressed (that is if I press a key during the inner while loop is executed, it wouldn't actually stop the loop and exit it, to do that I must actually hold down the Esc key until the inner while loop finishes (or press the Esc exactly at the very end of the loop) to check the condition !KeyAvailable)
Could anyone recommend me an alternative to this?
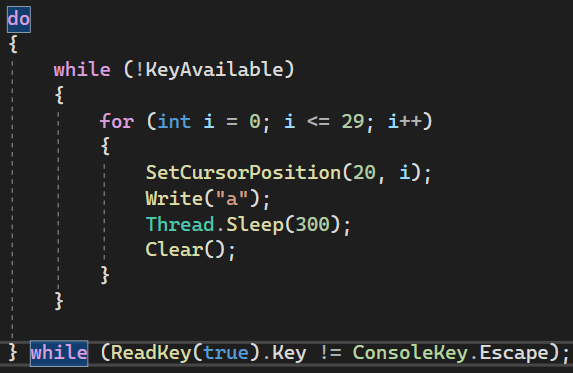
7 Replies
are you searching for something like this:
Console.WriteLine("Press a key");
var end = false;
while (!end)
{
var keyPressed = Console.ReadKey().Key;
Console.WriteLine($"You pressed {keyPressed.ToString()}");
if (keyPressed == ConsoleKey.Escape)
end = true;
}
Console.WriteLine();
well kind of, but the main thing is that I want the whole block of
SetCursorPosition(20,1);...
to be executed if the esc key is not pressed
kind of like having a background task running?
I figure that if i had a ReadKey(), it would mean that I'll have to press a key everytime the letter "a" finishes falling from the top to the bottomDepending what you exactly want it can be more complicated.
But start with replacing:
var keyPressed = Console.ReadKey().Key;
Console.WriteLine($"You pressed {keyPressed.ToString()}");
with you're for loop.
But Maybe a better sollition is to put youre loop in a different method. I will make it in a minute my cat has priority
Alright, thank you very much
Console.WriteLine("Press a key");
var end = false;
while (!end)
{
end = downloop();
}
Console.WriteLine();
bool downloop()
{
// you'e loop
for (int i = 0; i < 40; i++)
{
if (Console.ReadKey().Key == ConsoleKey.Escape)
return true;
// the other stuf
Thread.Sleep(300);
}
return false;
}
add you're loop within the method downloop. PS. I cant copy from a picture so you have to do it you're self
Thank you very much. I ran it succesfully
youre welcome