❔ Random problem
When choosing a random number in c#, I don't want the same number to come across again, how can I do it?
40 Replies
Do you mean 1, 2, 3, 4 is okay;
But 1, 2, 3, 4, 1 is not okay?
Yes, define your exact requirements. How many random numbers do you expect to generate and does it matter what range of values they fall into?
There are probably several ways of solving this depending on your exact needs.
You can try something like this if I understand correctly what you want.
A HashSet would be a much better data structure than a List for keeping track of the values that have already been used
That's what I was thinking but I didn't want to type it out on my phone without being sure of reqs
Fisher-Yates shuffle
and call it a dayHow does shuffling help?
Doesn't that require a known set of values first?
1..int.maxvalue
yes i want this I'm sorry for the late reply
i will try
thank you all in advance
like @mtreit said, a HashSet will better handle the case of I've already got it
How many random numbers do you need to generate?
Because by the pigeon hole principle you will eventually have to repeat.
estimated 45-50
Do they need to be within a certain range?
OK, let it be repeated, but for example, coming back to 5 after 5 is a problem for me.
yes
What's the range?
1-2
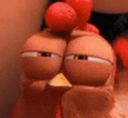
There's an infinite set of numbers between 1 and 2
3.14 to 3.15 is interesting too
I don't understand much from c#. I'm working on Unity and I want to turn off the random number doubles in my game. It doesn't feel right for me to share because there are Unity codes
rndm = Random.Range(1, 45);
So you want to hand out 50 random numbers in the range of 1 to 45 without duplicates?
I can explain like this.
34,23,43,43
I don't want the same number to appear side by side again. I want the incoming number to be less likely to come back
Im really sorry if I cant explain but my english is not that clear
or if a number comes up once, number must wait for the other 50 numbers to finish before it comes back
I think you can achieve this by simply generating a pool of numbers, shuffling them, and then keeping a pointer into that shuffled set and using that to hand out the next value.
Do you want to hand out more numbers than is in the range (like the example I gave?) Because that's obviously a problem since duplicates are guaranteed 🙂
Yes it makes sense. I will do it. Thanks for your help
No, I know I can't explain my problem fully, but I think I'd better do what you just said.
I'm not sure it's the best approach, you could still end up with duplicates next to each other in the sequence.
@!Scofield here is a completely brute force way to do this that will definitely work. It has theoretical performance problems if the same number that has already been sent keeps getting generated, but in your example it's probably fine.
like, 34, 23, 43, 20, 43 is ok?
Sample output:
No number will ever repeat until all possible values have been sent
Performance of this seems totally fine after testing, I think it's a much better solution than my original suggestion.
Fisher-Yates shuffle wins!
Fyaseh-Resti Shuffle
No, it was rejected
Im really grateful, sorry if I stole your time
Not at all, it was a fun problem to think about.
Actually I guess if you re-shuffled after the entire sequence has been produced you would avoid repeating the same exact number sequence over and over again.
Yep. It's deterministic.
Here's the same program but using Fisher-Yates
Was this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.