✅ Some web things
Soooo... i have this app
problem - On home page i have link which goes to /users, i wanted it to work like, first time when go to users, send users html page, and on this users html page wanted to make <ul> with event listener "onload" to request data. any advice?
28 Replies
Not sure why you need the middleware
But yes, you certainly can do that
me too, some old preoblem when i didn't get how to send html and db data together
Well you can't do that
problem is -

Sending the static file is one thing, one endpoint
Sending the data encoded as JSON is another thing, another endpoint
so, how do i do tihs? should i send html page as static file?
forgot to send static files and was confused why tf js not working lmao
so, i got
this tabs....
and got
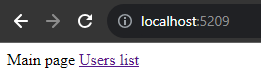
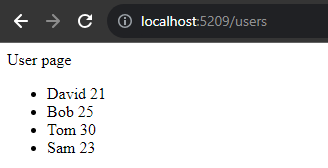
lgtm
thx a lot again, now i even realize what is this "api" ting in route is 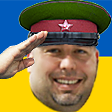
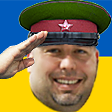
Anytime
btw, today i had resuls api day at my corse and i decided to refactor it like this -
is this better?
also wanted top make something like this,
That string interpolation isn't necessary there
And I personally prefer using
var
butt im forget some c# basics and got funny thing

But besides that, sure, seems fine to me
And yeah, this works
But you need that lambda
yeah
i did it
async () => await SendHtml("page")
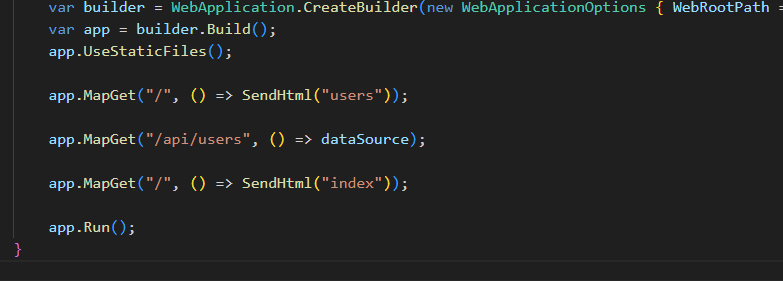
but have some error
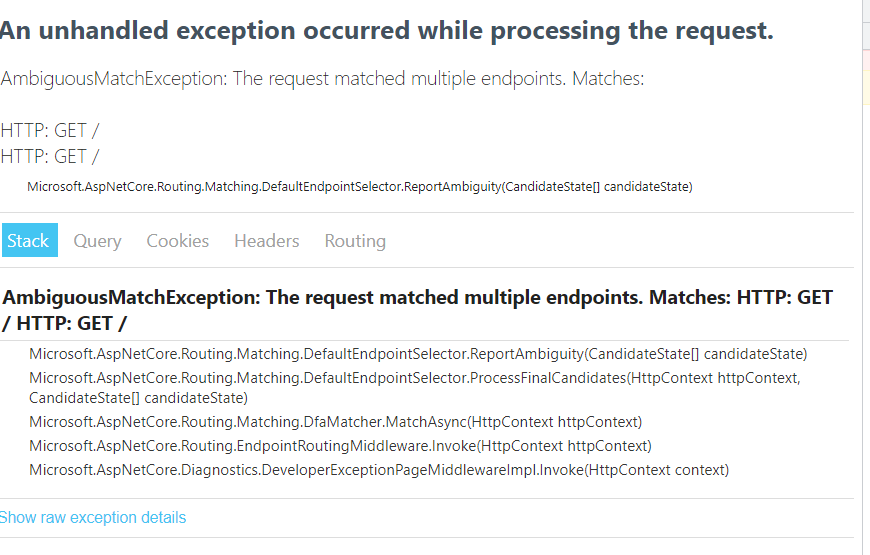
was reading rn
oh
nvm
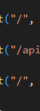
i copied
yea
looks better now
but... any advice about placeing of things?
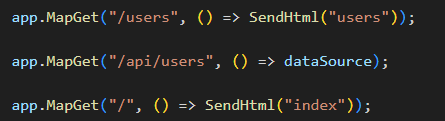
i mean, looks like they are in totally random order, and its not rly matter when tey are to work
Personally, I like ordering routes from most shallow
So
And grouping by area
You can also use route groups for that: https://learn.microsoft.com/en-us/aspnet/core/fundamentals/minimal-apis/route-handlers?view=aspnetcore-7.0#route-groups
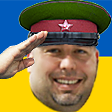