Cannot use tRPC infinitequery in SolidStart app
We (me + @pupbrained) have a solidstart app bootstrapped with create-jd-app, using trpc, prisma, zod, and solidquery. We have a component that hits our api:
However, it doesn't work... at all. The query errors with
No QueryClient set, use QueryClientProvider to set one
. We tried putting the provider in both root.tsx and this component. First image attached shows the trpc procedure for getInfinitePosts, second image shows our root.tsx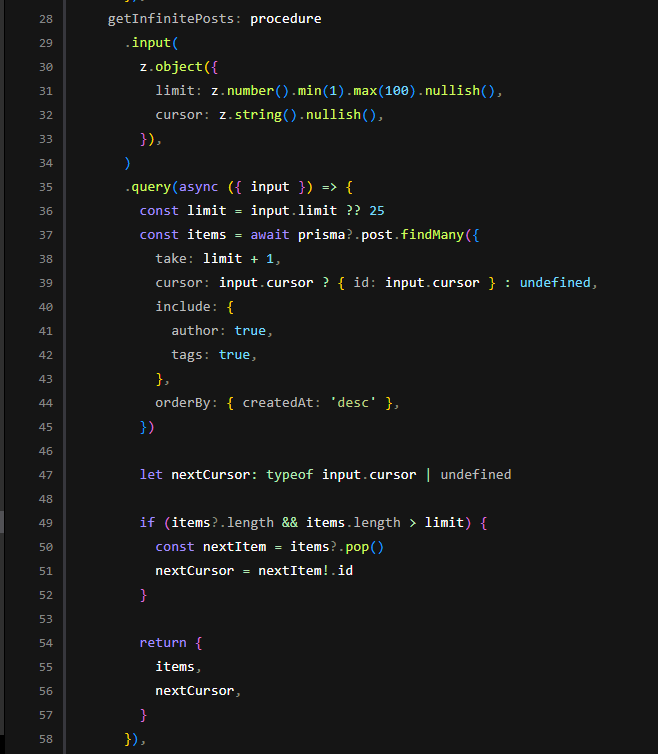
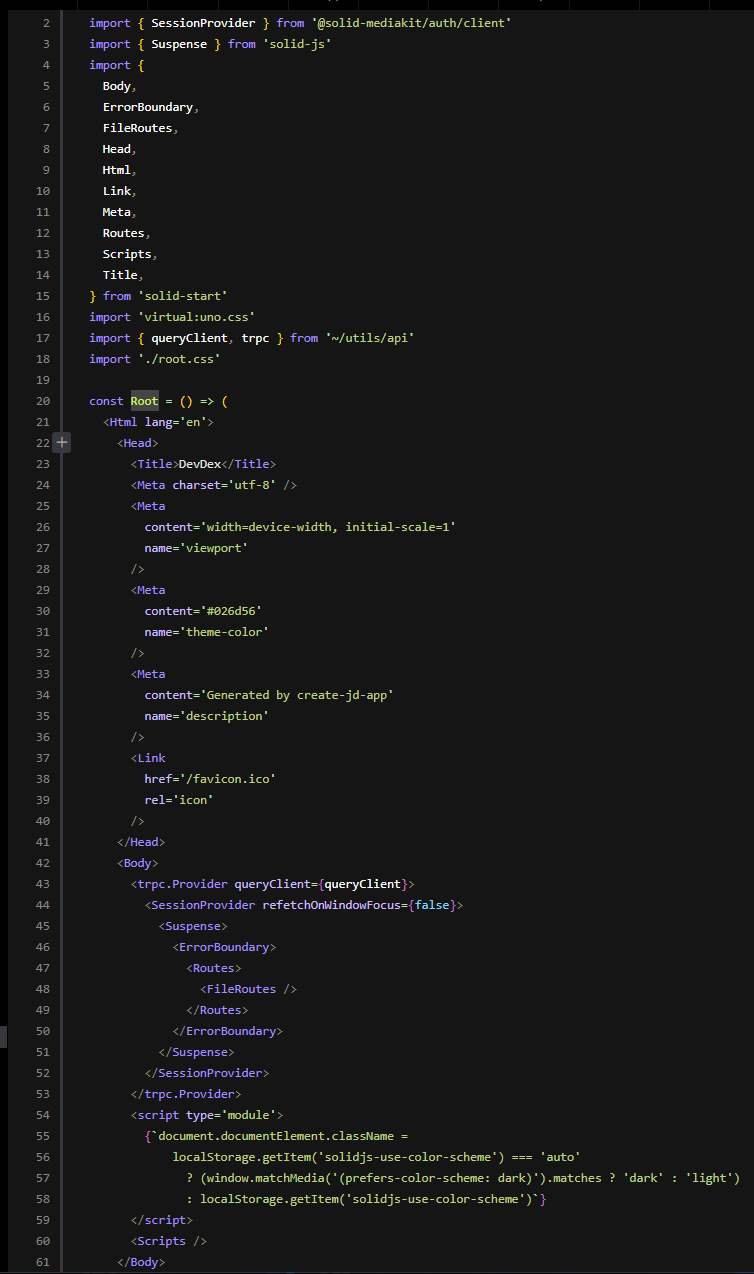
1 Reply
...overall just unsure where to go from here. i'm not sure if our entire app is setup wrong or something. i also tried making the Timeline component client-side only using ClientSide(), but that didn't change anything