❔ EFC Is not loading collection properly
I have the following 2 models in my database, EFC does not seem to be loading it at all for some reason
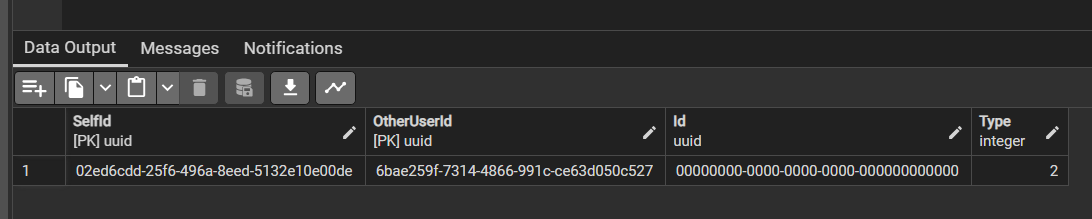
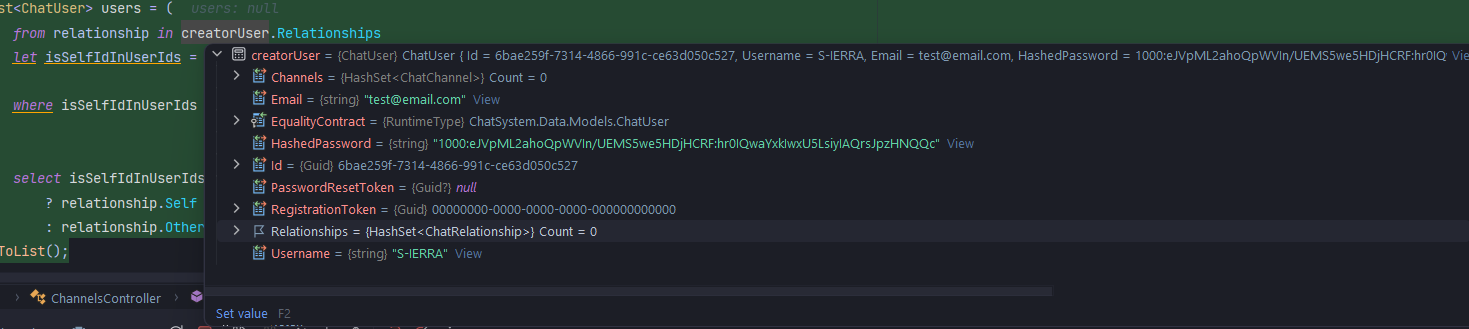
77 Replies
Possibly what I assume could be happening is its trying to search by "SelfId" when the key is saved to "OtherUserId" ? tho the behaviour I'm expecting is its fetching any table that contains the value, both are keys so it shouldn't be a problem unless thats not how it works and its only searching by the 1st key
EFC does not seem to be loading it at all for some reasondefine this
1. Don't use lazy loading
2.
.Include()
the related data you need
3. Or better yet, .Select()
what you needI was told to use lazy loading any idea why I shouldn’t do it? I ve tried doing with include but same outcome
Relationships shouldn’t be 0
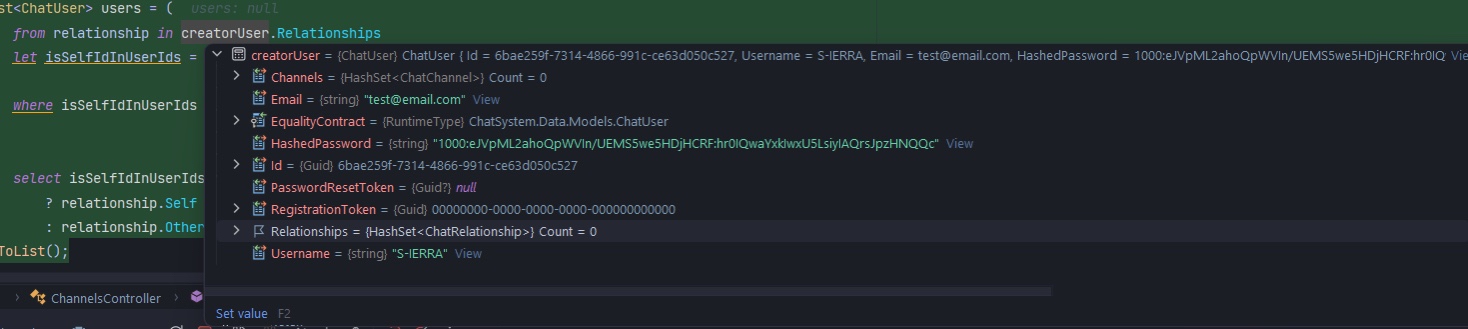
Because lazy loading will query the database multiple times
So why do that, if you can query it once for all the data you need
in a difficult to predict way
with thread blocking
I see, I can switching to load regularly that’s not an issue I guess, tho no idea why it still isn’t being loaded at all
Since it's lazy-loaded, the related data will only ever be queried for when you access that property
So, do you?
I would believe so
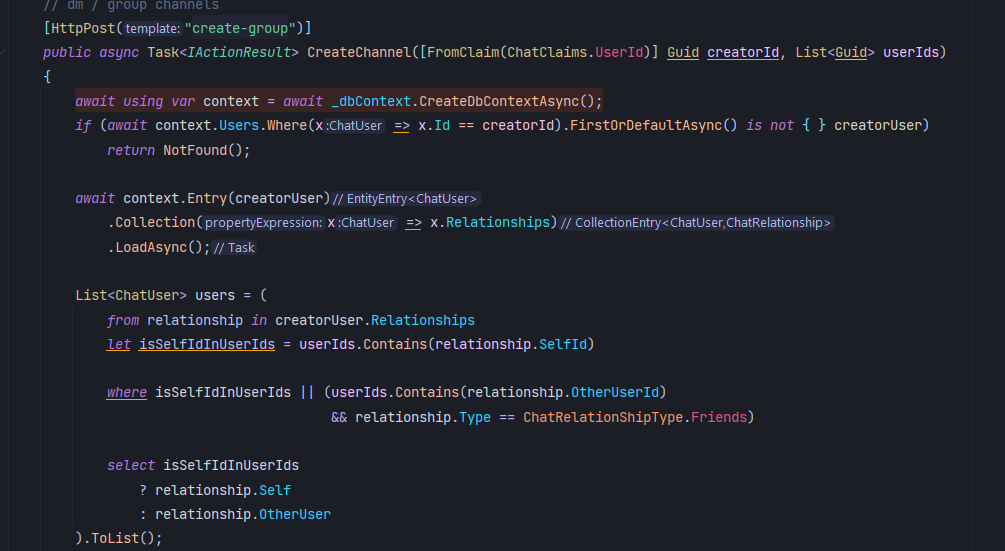
Do you have
UseLazyLoadingProxies()
in your EF config?No
https://learn.microsoft.com/en-us/ef/core/querying/related-data/lazy
I feel dirty posting this link
Haha why
Because I'm actively helping someone make lazy loading work
Instead of discouraging them from using this shit
I should of probably informed my self a bit more on this, just this is the way I've been taught the entire time
And telling them to
.Select()
dw I ll switch it
tho in some cases I have to use lazy loading
i.e loading server members
Why
Why
well youre not gonna load 100k entites at once are you
ig paging does the job
No, you paginate it
fucking hell gonnaa be funny swapping the entire logic from lazy loading
what would the difference between select and include be ? if at all
.Include()
means you're loading the entire entity and the entirety of related data
Which is not always a good idea
For example, if you want to display the user profile and their posts, if you do
it will load everything
Including the user's password hash, their email, and all of their posts with their whole bodies and everything
But you just need the user name, avatar, and the posts' IDs and titles
So you can do
And it will get only that dataoh
yeah... thats alot better
The difference between
and
well depends on the scenario bu tyeah
if i remeber correctly virtual on icollections marks the entity as being loaded lazily

yep
On any related data
switched to this
removing that virtual requires db update or no need
No need
It doesn't change anything about the database
Just about how EF queries for items
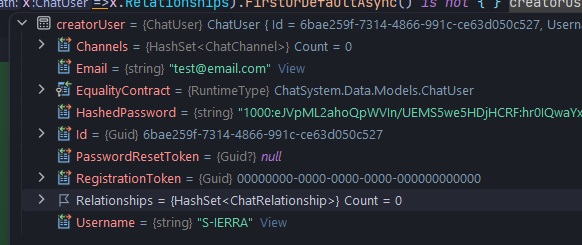
ong im gonna shoot my self
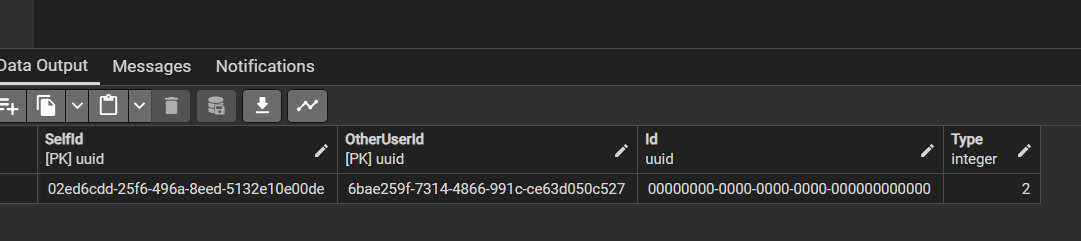
its there
The properties have to be virtual, because EF basically overrides
into a
i think it could be bcause its querying by "SelfId" in this case its in OtherUserId
huh
fails to load them
it could be my garbage config
Well,
OtherUser
is not said to be related to Relationships
, technically... So I wonder
It shouldn't matter, I don't thinkis it not?
eeeeeeeeeeee
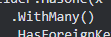
.WithMany()
is emptythought thats more of a way to reuse the existing relationship
Again, I don't think it matters that you explicitly do that
But it might
because
See what SQL your query generates
Maybe EF doesn't know it has to join on both
SelfId
and OtherUserId
this is the wrong thing
there
Yeah, it only joins on
SelfId
Any clue why that is
I assume its because of the SelectMany()
Possibly
tho if i were to write a second " .WithMany(x => x.Relationships)" it would error and say that relationship already exists
Right, I was afraid this might be the case
No clue why I wrote "afraid" as "fartaid" lmao
dw haha, and hm weird I ll have to look around how I could make a "friendship" model so to say
cuz it seems to be a pain in the ass
The easiest way would be to have two collections
MyRelationships
and RelationshipsWithMe
But that's more akin the followers system, not friendship where it has to be mutualStack Overflow
How to Model Friendships Between IdentityUsers More Directly in Ent...
I'm trying to make a simple Social Network (MicroBlog) with ASP.NET Core 5+ with Entity Framework Core. I ran into a problem when modeling the friendships between the users and fetching friends of ...
yeah found this but like
exact same issue 1:1 just there is no replies
I wonder if you can go around it with a manual join
Possibly, how would I do that?
.Join()
programming is my passion
manual join doesnt seem to be doing much, also its not really a good fix, i ll look around because i'm sure there is some weird ass way of doing this
Try asking in #database
@ZZZZZZZZZZZZZZZZZZZZZZZZZI'm thinking a hacky ass method I could write is if I made their relation ship many to many instead
I mean, it already is many-to-many
users-to-users
yeah just written weirdly
Except the join table has some added data
Yeah
yeah im a dumb ass the way i wrote this
the fuck
Nah, N-M with added data is fine
mm i see
It's just that EF seems to have issues with N-M where both sides of the relationship are the same
Yeah
And stored in the same collection
I will make it M-M
I've actually gotten in to work quite well
PreviousVersionId and NextVersionId
just had to configure the relationships manually, but the nav props worked fine, IIRC.
Was this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.