❔ What is problem?
using System;
using System.Net.Http;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
namespace GenesisAPIFormApplication
{
public partial class Form1 : Form
{
private string apiUrl = "https://cluster-2.pudochu.repl.co/api/genesis";
private HttpClient httpClient = new HttpClient();
public Form1()
{
InitializeComponent();
}
private async void button1_Click(object sender, EventArgs e)
{
// Sabit bir metin kullanarak API'ye metin gönderme işlemi
string prompt = "Senin adın Jarvis. Her şeyi bilen üstün bir yapay zekasın.\n\nBen: " + "selam" + "\nJarvis:";
try
{
var content = new StringContent($"{"prompt": "{prompt}", "model": "text-dream-001"}", Encoding.UTF8, "application/json");
var response = await httpClient.PostAsync(apiUrl, content);
if (response.IsSuccessStatusCode)
{
string responseString = await response.Content.ReadAsStringAsync();
MessageBox.Show(responseString);
}
else
{
MessageBox.Show($"HTTP Hata Kodu: {response.StatusCode}");
// HTTP hata kodunu burada işleyebilirsiniz.
}
}
catch (Exception ex)
{
MessageBox.Show("İstek gönderilirken bir hata meydana geldi: " + ex.Message);
}
}
}
}
46 Replies
Idk, what is the problem?
Does it crash? BSOD your PC? Make your plants wither?
if clicked buton1 say in the html code : SyntaxError: Unexpected token
in JSON at position 71
at JSON.parse (<anonymous>)
at parse (/home/runner/Cluster-2/node_modules/body-parser/lib/types/json.js:92:19)
at /home/runner/Cluster-2/node_modules/body-parser/lib/read.js:128:18
at AsyncResource.runInAsyncScope (node:async_hooks:203:9)
at invokeCallback (/home/runner/Cluster-2/node_modules/raw-body/index.js:238:16)
at done (/home/runner/Cluster-2/node_modules/raw-body/index.js:227:7)
at IncomingMessage.onEnd (/home/runner/Cluster-2/node_modules/raw-body/index.js:287:7)
at IncomingMessage.emit (node:events:513:28)
at IncomingMessage.emit (node:domain:489:12)
@ZZZZZZZZZZZZZZZZZZZZZZZZZ
You're doing some JSON parsing somewhere, not anywhere within this code though
And that's throwing an error
Because whatever JSON you're trying to handle is not valid
at parse (/home/runner/Cluster-2/node_modules/body-parser/lib/types/json.js:92:19)this is a clientside error
ı am how to fix the problem (my english bad sorry :D)
I'd start by sending actual proper JSON, and not a string masquerading itself as JSON
how?
You should make a model for the data you're sending, then send that using
PostAsJsonAsync()
For example:
thanks
@ZZZZZZZZZZZZZZZZZZZZZZZZZ
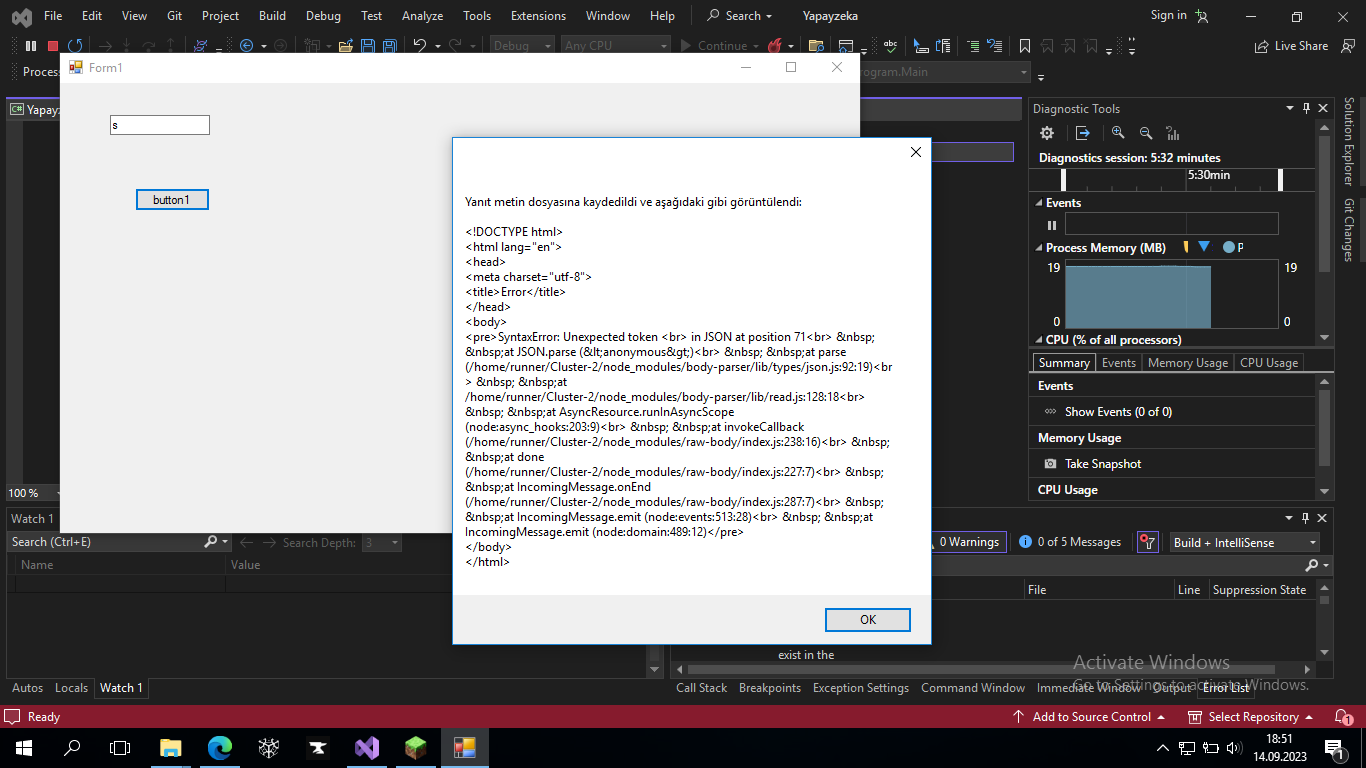
Show code
using System;
using System.Net.Http;
using System.Threading.Tasks;
using System.Windows.Forms;
using System.Net.Http.Json; // Uzantı kitaplığı
namespace GenesisAPIFormApplication
{
public partial class Form1 : Form
{
private string apiUrl = "https://cluster-2.pudochu.repl.co/api/genesis";
private HttpClient httpClient = new HttpClient();
public Form1()
{
InitializeComponent();
}
private async void button1_Click(object sender, EventArgs e)
{
// Sabit bir metin kullanarak API'ye metin gönderme işlemi
string prompt = "Senin adın Jarvis. Her şeyi bilen üstün bir yapay zekasın.\n\nBen: selam\nJarvis:";
try
{
var response = await httpClient.PostAsJsonAsync(apiUrl, new { prompt = prompt, model = "text-dream-001" });
if (response.IsSuccessStatusCode)
{
var responseString = await response.Content.ReadAsStringAsync();
MessageBox.Show(responseString);
}
else
{
MessageBox.Show($"HTTP Hata Kodu: {response.StatusCode}");
// HTTP hata kodunu burada işleyebilirsiniz.
}
}
catch (Exception ex)
{
MessageBox.Show("İstek gönderilirken bir hata meydana geldi: " + ex.Message);
}
}
}
}
$code
To post C# code type the following:
```cs
// code here
```
Get an example by typing
$codegif
in chat
If your code is too long, post it to: https://paste.mod.gg/$codegif
@ZZZZZZZZZZZZZZZZZZZZZZZZZ
Everything seems fine, which means it really is an error on the server's side
or .net version?
No
oke
The error is on the server's side
Since it says the error occured on line 71 of some JSON, and you're sending 4 lines at most
So it probably handles some different JSON, and that's what throws the error
@ZZZZZZZZZZZZZZZZZZZZZZZZZ or bad json connection?
There's no such thing as "json connection"
You send valid JSON to the server
The server has an error due to some other JSON that's at least 71 lines long
The issue is with the server code
@angius he said bad json connection
@pobiega
dont ping people
okey
Well, you're not sending JSON with 71 lines, all your code is correct, it really doesn't seem like anything wrong with your code
hmm
Is there an API documentation or what kind of API is this?
question answer
Do you have a working example from Postman or any other source?
example proggaming languege is JavaScript
Show me a working example from this API
because if we don't know if the API works at all, there is no sense in going any further
Well, the data you're sending with C# doesn't contain any
messages
Is it an optional property?Just did a small test with JSON, the API itself works, that's the JSON I sent:
Response is:
So it probably needs messages
Yep, removed messages, returns still 200 but with an error message, a bit weird API behaviour but we can't change that I guess
So, oddly, not the error we've seen in this thread
What if instead of messages you have the
prompt
property?let me check
So if that's the JSON in the POST request:
We get this:
ah wait, forgot the comma
sorry!
So that's the valid JSON we sent:
Response:
So it seems like the structure is supported
Where do I need to enter it then?
Well, this code looks just fine
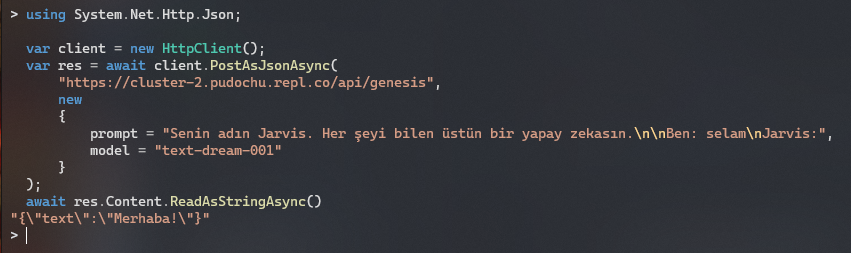
So
Did a small test myself, works also:

Was this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.