❔ Defining base impl ctor in interface
So currently for some testing I have the following code:
my ctor
Foo
is pretty generic, in fact I'd probably want all my derived classes to have that exact ctor. How'd I achieve this?91 Replies
Foo a = new Foo { Name = "John", Description = "A person" };
You can use the
: base()
Or an abstract class igabstract class is the same as in C++ I assume?
at least one pure virtual method
and its abstract?
There's
abstract
modifier
You can have an empty abstract class just as wellah right
Angius
REPL Result: Failure
Exception: CompilationErrorException
Compile: 454.425ms | Execution: 0.000ms | React with ❌ to remove this embed.
mhm fair enough
do ppl even use the interface keyword often
seems terribly limited
honestly
Yes, interfaces are very common
C# has no multiple inheritance
But a class can implement many interfaces
unless its an interface no?
yy
eh, that blows, what about my
init;
approach, would that be a acceptable approach?
or do people avoid this altogetherYeah, initializer syntax is commonly used
And yeah, you can use required
required as in?
there's a keyword?
ah
so we force them to set it
since i have a non-nullable type its actually pretty relevant
Angius
REPL Result: Failure
Exception: CompilationErrorException
Compile: 478.418ms | Execution: 0.000ms | React with ❌ to remove this embed.
Angius
REPL Result: Success
Result: Foo
Compile: 522.218ms | Execution: 62.802ms | React with ❌ to remove this embed.
required
does not work within an interface
it 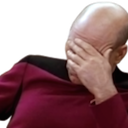
$getsetdevolve
can be shortened to
can be shortened to
can be shortened to
can be shortened to
though, in practice the
get;
and set;
are much like changing the visiblity, rather than utilizing a setter/getterThey're literally getter and setter
See above
ye with some magic
bcs when you are actually setting the value its literally
obj.val = 34;
not obj.SetVal(34)
, I dont really see the whole setter/getter
back in practiceWell, yes, with some magic
We ain't writing Java, we don't write useless code lol
its literally just like a accessibility modifier
in C++ you'd mark it
public
and call it a day
because thats essentially what it isIt's about encapsulation
You expose the internal state of the object via this proxy
I assume it has no additional checks
for
set;
?Well, it can if you want
You can have
{ private set; protected get; }
if you want
Or you can implement some checks in the setter or getter
what's the difference? Pratically none
Yeah, you're exposing the internal state directly
so it
get;
no?
only difference is that you cannot set;
, its split up and one protects the other from happeningAnd if you wanted to not have the ability to set it, or not have the ability to get it, or return
0
if the member is < 0
you will have to break the contract by introducing getter/setter methods
Not the case with C#Fair
guess it has some use, a fany accesibility modifier I'll call it
protected doing what in this context
is it so only the derived can use the
get;
i assume that actually, on second thoughtprivate
makes the setter accessible only in this class, while protected
makes the getter accessible in this class and all derived classesits private by default I assume
It's whatever the property is, by default
which is private unless you make it public?
y
you see, this is what i'd want
however this wouldnt work, but it got rid of the dumb double declarations of the
Name
and Description
, how'd you write this?I'd honestly just use an abstract class instead
Guess I will have to start making more proper distinctions between interfaces and abstract classes
in my decision making
lol
oh well, not the worst, safe to say im not a fan of interfaces in C#.
Interfaces are best treated as literally just a contract
Yeah, they're very limited
sadly
guess Ill have to respect their use case more
and stop trying to reinvent their use-case
@angius So having members (or fields whatever they're called), is typicall not something you'd see back very often in an interface
they're purely just: methods one has to implement in its child classes, maybe some default methods that can be overrided if wanted)
and thats it
Fields are an implementation detail, as they're usually private
Properties and methods you would absolutely see in an interface
properties that have to be re-declared a tenfold?
might as well omit them in the interface no?
I suppose they enforce you to
define them
in the child classes
Well... anything an interface declares has to be redeclared in the class that implements it
but its duplicate code
disgusting, might as well opt for a abstract class instantly?
Unless you use the default implementation, which is probably one of the biggest mistakes C# did lol
why's that, whats the tea here
An interface should be just that, a contract
It should contain no implementation detail
Implementation is up to the class
fair actually
considering they have
abstract class
tooDefault implementation was added so it's easier to refactor code, you can throw a
NotImplementedException
for example
But people abuse it for more that that
any other important details im missing
I assume thats all, pretty straight forward since its so limited yet simple.
I suppose this'd be a proper use-case, my example.
I assume dtor's are also not possible in the interface, right?
LGTM
Just to be sure, this whole interface contract goes as deep as the compiler right? There's no case of vtables being generated, polymorpish, etc?
And no, I don't think deconstructors (or do you mean destructors – finalizers?) are possible in an interface
you can create instance of
ISoundMaker
interface as I tested, this makes me suspect there's smth going on
destructors
, and I cant I just tested! Apologies I forget to share my finding.Not sure about the deep workings, but you cannot instantiate interfaces
Angius
REPL Result: Failure
Exception: CompilationErrorException
Compile: 448.304ms | Execution: 0.000ms | React with ❌ to remove this embed.
No, just save its child classes inside it
whats the command?
to compile
;compile
!e
joren
REPL Result: Success
Compile: 472.861ms | Execution: 57.902ms | React with ❌ to remove this embed.
ye
, and then when you'd call a method on
f
, it'll call the respective method of the underlying type
thats polyphism concepts applied
without vtables, virtual methods?Well, you're not creating ain instance of an
IFoo
yes
It's still an
A
so it just treats it as
A
, but allows it to be represented by IFoo
?Angius
REPL Result: Success
Result: bool
Compile: 445.172ms | Execution: 33.085ms | React with ❌ to remove this embed.
Yep
that 

It's an upcast
And
is
is for pattern matching
It's extremely useful, believe me
Especially with list patterns and allbut how do virtual methods differ in C#
do they create vtables as in C++
or is that knowlegde not applicable
A
virtual
method is an explicit signal that it's expected to be overriddenthis is absurd, the moment one would
ISoundMaker dog = new Dog() { Name = "Doggo" };
you'd get a compiler error
in C++
unless its a pointerWell
Dog
is a reference type
So kinda-sorta a pointer lolthat would also not compile
in C++
its weird to me that
ISoundMaker dog = new Dog() { Name = "Doggo" };
is basically treated as a pointer
but if that were the case
are there vtables generated, I assume not?
it just holds an alias to Dog()
instance
and allows it since its a reference type and its a child class of ISoundMaker
..?Honestly, not sure about the nitty-gritty of how it works
People in #allow-unsafe-blocks might have a better idea about vtables and what not
I'll have a look at that channel after I've read the whole polymorpishm post on the officials docs
for now my knowledge of interfaces should suffice I guess
im just being pragmatic for no reason, it matters when I have to dive deep which isnt soon
joren
REPL Result: Failure
Exception: CompilationErrorException
Compile: 408.788ms | Execution: 0.000ms | React with ❌ to remove this embed.
why's this though, since its just a contract you'd think just declaring it would be ok
Well, yeah, it's a field. An implementation detail
You'll need to make it a property
my terminology is not up-to-date with the csharp conventions
$structure
For C# versions older than 10, see
$StructureOld
Here's a cheat sheet
so a field is basically a class member
Everything inside of a class is this class's member
a property is basically a class member with certain properties, like
get;
and set;
?
by C++'s terminology you have: class members and class methods
and thats itRight, yeah
its just to compare for me, sorry!
a property just being a class member with extra utility
so to say?
properties being
set; get; init;
etc?
basically when the { ... }
is introducedYeah
so in this case the property
Name
in ISoundMaker
tells us that you have to define get;
in its child classes correct, and then we can add others like init
ourselves if we seem fitjoren
REPL Result: Failure
Exception: CompilationErrorException
Compile: 664.426ms | Execution: 0.000ms | React with ❌ to remove this embed.
shouldnt work
nice
ok, makes sense
Was this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.