Precondition error
Pastebin
Error - Pastebin.com
Pastebin.com is the number one paste tool since 2002. Pastebin is a website where you can store text online for a set period of time.
23 Replies
messageRun
needs to return this.ok()
, not just call it
(would be caught by ts 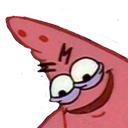
still returns the error
if (!interaction.guild) return interaction.reply({ content: "You cannot use this on a DM.", ephemeral: true, });This path also doesn't return this.ok() or this.error()
wdym
All paths have to return this.ok() or this.error()
ah
I would suggest you leave the sending an error message to the *CommandDenied event though
so you could just do
this.error({ message: "You cannot..." })
in all your preconditions, and then the event would take the message and send the erroralright
messageRun
should return ok()
, not some()
. Use your text editor's type hints
What is this ðŸ˜
- if no guild
- return ...
- else, if guild
- return ...
- else, if no guild
- return ...
That could just be
Anyways, check what's happening in contextMenuDenied, make sure it's firing, log interaction.guild
to see if it's what you expect it to be... take some steps to debug on your ownit still lets me run message commands on dms
which is weird since I have a global precondition (position 2) where it checks if the command is being ran in a guild or not
Yes... if your messageCommand method returns
this.ok()
then it will pass
why do you have 2 dm preconditionswdym 2
the other one I was talking about also checks for certain channels ids
Ok
Could you send your updated code?
looks like i fixed it, just had to return this.error() on messageRun
Yes. But I assume you added in functionality to check if there was a guild and didn't have it return this.error() always?
wdym
nvm it now doesn't let me run commands on guilds anymore
lol
as I said here, you need to add the same functionality in messageRun that you did in contextMenuRun
added
return !message.guild ? this.error() : this.ok();
and it worksRight, but note you're not sending the same error message as you are in the contextMenu function. I suggest consistency.
(also nit but it's generally a good idea for readability to not make the condition a negative if there's an "else", example:
alright thanks
idk which message to put as an answer
42
^ do that one /j
no
just gonna put that