❔ ✅ JWT signatures not working
I took the public and private key as well as the jwtMessage from sample data on jwt.io. I created my own siganture for the token using the private key from their, and tried to verify the signature using the matching public key I took from there. What am I doing wrong?
4 Replies
I also tried
RSASignaturePadding.Pkcs1
, output stays false
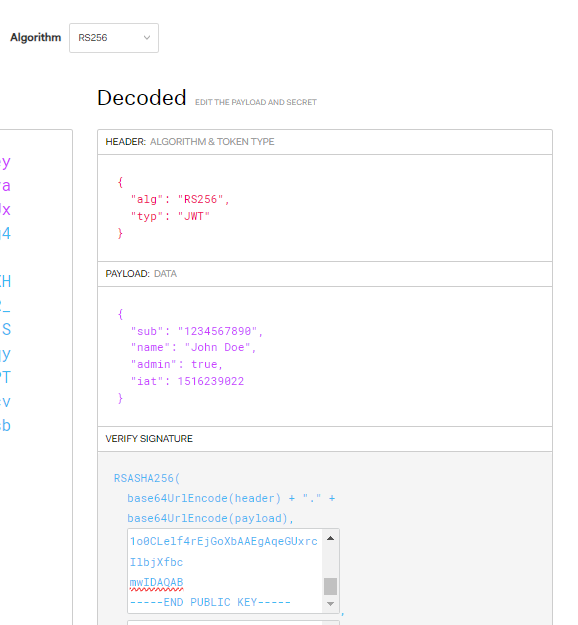
jwtMessage
is equal to base64UrlEncode(header) + "." + base64UrlEncode(payload)
This code works, I had to take decoded signature but encoded message together with Pkcs1 paddingLooks like nothing has happened here. I will mark this as stale and this post will be archived until there is new activity.