What are the differences? Produce vs regular setStore
This is Astro + SolidJS components.
Question:
Besides produce allowing to neatly update specific properties of an object, are there any other differences? Any performance nuances between these 2 approaches dealing with stores?
P.S. sorry for bad naming, was just playing around.
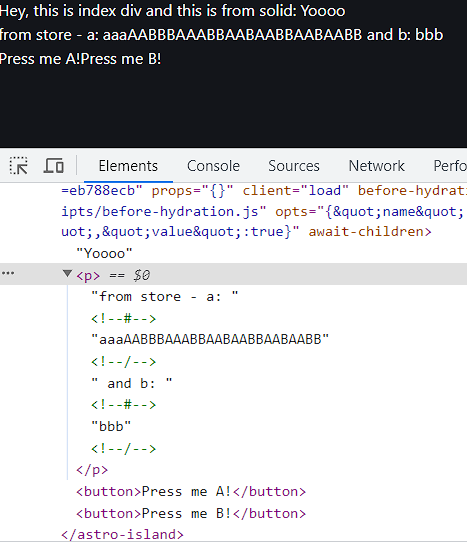
1 Reply
produce uses a proxy to map the mutations of the object to the underlying signals (similar to how immer does it), so presumably there is some overhead there.
There are some minor differences in setting a property in produce in comparison to setting it without, see p.ex https://discord.com/channels/722131463138705510/1153225388370186330
I suppose the proxy u get from produce is (a lot like)
createMutable
, but that's just me assuming.