Inventory Maintence Project Issue
I have two forms, one that lets you enter data for a new item. Once you hit Save, it should create a new InvItem object and add it to a list of inventory items and display it in the list. I can't seem to figure out how to get it to add the item. Any suggestions?
13 Replies
someListOfItems.Add(newItem)
There's a helper class called InvItemDB.cs and I'm somehow supposed to use a method from it to add the item and save it.
Pastebin
InvItemDB.cs - Pastebin.com
Pastebin.com is the number one paste tool since 2002. Pastebin is a website where you can store text online for a set period of time.
Here's the new item form
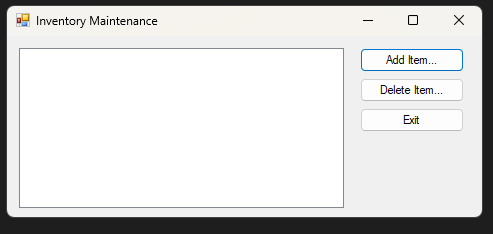
That helper class lets you save a list of items to a file, yes
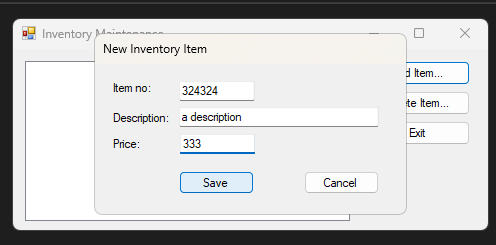
So you need to read items from that file, add the new item, then save items to that file again
Yeah but the list isn't updating once I save and load the items back.
On
btnSave
click you get the items from file
I don't see you saving themI've taken a long break from coding and this is very frustrating that I can't figure this out
FillItemListBox();
should do it, right?Where do you call
InvItemDB.SaveItems(items)
?
Because that is what saves items to a file🤦♂️ oh
lemme try that