How to create a product with a category id?
The setup:
lib/app/shop/resources/category.ex
lib/app/shop/resources/product.ex
Question: How can I create a new Product which includes a Category?
This is what I tried:
The
category_id
is nil
both times.9 Replies
use manage_relationship
roughly something like that ( i think you may have to swap the first two params in the manage_relationship)
or directly on the changeset
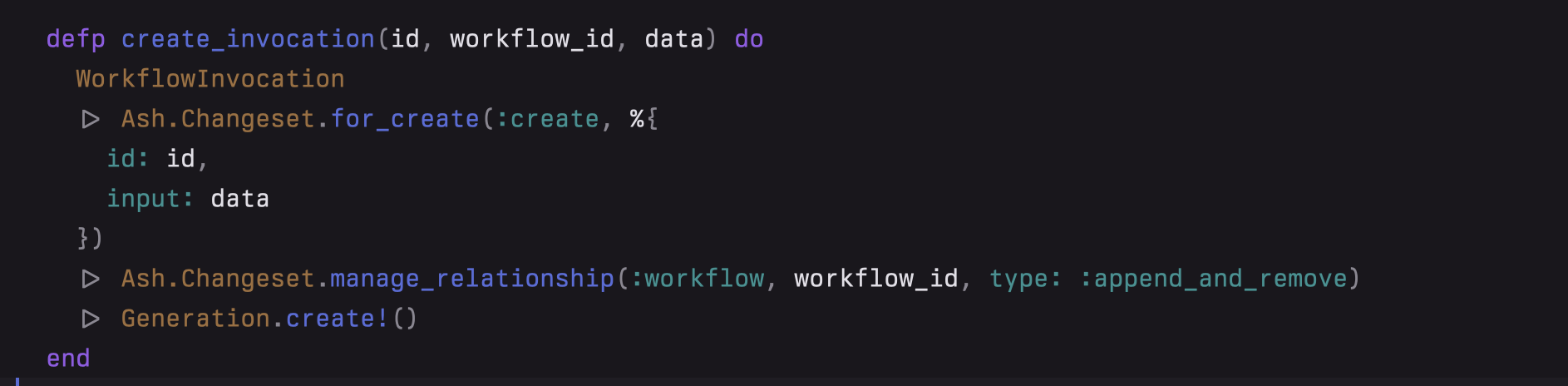
If you wan't to set the id directly you need to make the attribute writeable https://ash-hq.org/docs/dsl/ash-resource#relationships-belongs_to-attribute_writable-
What is the right syntax for that? https://ash-hq.org/docs/dsl/ash-resource#relationships-belongs_to-attribute_writable- doesn't contain an example. This doesn't work:
I think it should be
attribute_writable?
not just writeable?
inside the do\end
block you never have to use :
Thanks @barnabasj!
For the archive. Here's the fixed code and how it's working:
This doesn't work:
Should it but I configured the resource wrong or shouldn't it never because this isn't ActiveRecord?
No it shouldn't work out of the box. If you wan't that you need to use manage_relationship like kernel suggested
@barnabasj Since I am writing beginners documentation I'd like to know if I understand it right:
Code like
App.Shop.Product.create!(%{name: "Orange", price: 0.20, category: %{name: "Fruits"}}
is not the Ash way or is it?
I am not so much interested in what is possible by opening all escape hatches but what is the intended way of doing it. I have one new Category and I have one new Product. What is the fastest way of creating it? What is the Ash way of doing it?I think both ways are equally valid and Ash just doesn't assume what you want. You have to be more explicit by telling ash how you would like to manage your relationships
I use both ways in my code. Depending on the life-cycle of the resources and which resource I want to manage the relationship and so on.