Fragment with multiple 'from' items.
I'm trying to implement search similar to: https://leandronsp.com/a-powerful-full-text-search-in-postgresql-in-less-than-20-lines where the query looks like this:
I think I would write a fragment like:
on the read action.
However for the
FROM
side of things, do you think those items should be in a custom calculation?
I had tried:
but get this:
which makes sense but I"m wondering how to make them work together.28 Replies
what is
websearch_to_tsquery
?
oh, thats the postgres function
Regardless, the problem is referencing fields raw in the fragment
So arguments referenced in calculations go on the calculation itself
And then when using them you provide them as a keyword list
awesome ty I'll try that out!
So you can compose calculations using that tool
Just remember that if you're using AshGraphql and the like to mark calculations that are just meant for composing with other calculations as private
brooooo it worked!! Man this is awesome. Thank you so much!
Below is the calcuations and filters used.
Would sorting on this be possible?
yes
its a bit of a weird format
calc_name: %{...input}
or calc_name: {:order, %{...input}}
Thanks so much @Zach Daniel ! That actually worked perfectly for
similarity
! The rank_title is giving me a problem. I've tried solving it 3 ways without luck:
1. This way recalculates 'query' in the rank_title calculation in an attempt to return the rank:
but it fails where I'm trying to bind the word 'query' there.
I've also tried:
and then use it like:
but then in the prepare step I get: undefined function query/1 (there is no such import)
3. I've also tried prepare(build(load: query and having rank_title defined as:
but query isn't around.š¤
So, the main issue with the first attempt is that you can't nest calculations in the sort like that
you can only provide arguments to one calculation
well, technically you can, and maybe this is what you want to do, actually
So that is one way
the other way is to make a calculation like
where it calls
query
automatically and passes the arguments down
I would probably choose the latter šty! I'm trying them both right now
Weirdly I'm getting:
Ah, š¤ yeah, right
its because the
argument :string
š¤ š¤ š¤
one sec
maybe
can I see what exactly you've set up that gives you that?I seemed to have received that same error after trying both ways just fyi. below is the current setup:
This code runs in postgres directly:
so I know ts_rank exists.
Yeah, that makes sense
The basic problem is this:
The calculation says it returns a
:string
And so we tell postgres its a ::text
because that is how we handle stringsGot it. Where it should be either a float or null. I should just be able to switch it to float then
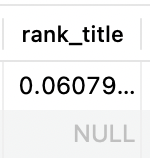
Ah no luck.
gotta drop off for a meeting here
Yeah, the problem is that its a
tsquery
I'll have a fix for you soon š
The easiest fix for this at the moment is creating a wrapper type for tsquery
called AshPostgres.Tsquery
So then what you'd do is make your argument argument :query, AshPostgres.Tsquery
and calculate :query, AshPostgres.Tsquery, ...
A new release of ash is out that has some needed fixes for this, and I'll push up the postgres side to ash_postgres
main
When you get a chance, try out ash_postgres
main and latest ash version and LMK if that resolves the issue š
okay, releasedWow thanks man. That was fast š
I just tried it and looks like I'm stilling hitting.. somthing. In mix:
I updated to AshPostgres.Tsquery:
Rank title:
Query:
and getting:
two things
the
:rank_title
doesn't need to be updated to Tsquery
that returns a float or something, IIRC
Where is the @@
coming from?
Feels like there is a part of the calculation failing that I can't see currentlyOkay I updated rank title to float and it's still doing the same.
The @@ is from postgres.
WHERE query @@ document OR similarity > 0
is the vanilla way to do it.
I've seen it elsehwere like this:
WHERE to_tsvector('english', query) @@ websearch_to_tsquery('english', 'natural loofah')
š¤
So you don't have
@@
in one of your calculations?correct
just the filter expression
š¤
oh
I'm pretty sure thats where its coming from
two things
that's the whole read action.
Best practice to surround fragments in parenthesis
we can't do it automagically for various reasons (but we might someday, when we can make sure it works for all variations of expressions)
second thing is what is
document
? IS that the string attribute on the resource?
tsvector @@ tsquery
is the type signature for that operatorOh shoot ya good point
so that shouldn't be a string then maybe
ah, okay
š looks like you'd also want a
tsvector
type along the same vein šAh nooo I missed that earlier man so sorry
no worries
not your fault
There should actually be some kind of escape hatch here to avoid needing to define type modules to map to underlying types
its easy to do, but not super easy to know how or that you need to do it
I've just pushed it up to
ash_postgres
main
give that a shot and see how it works for you šwow man lightning. š ty okay
it works!!!! šÆ
thanks so much man. that's amazing.
š„³