✅ How to get confirmation from user about reading the notification?
Notifications work easy in my case. I just send notification message to all clients using broker. UI (it is vue.js in my case) subscribes to topic and receives message than send it to clients.
Let's see some code:
But I don't understand how I can add confirmation for messages. For example, I want to send a notification and see if a person has viewed it. Can someone give me an idea, how to make this?
9 Replies
that's a very... broad question
you obviously know how to send messages back and forth between your clients and server
what part of "confirming that a notification was read" is your sticking point?
hmmm
maybe I just asked weird question
you asked right questions!
I can just send message to special topic in broker and wait for response on this topic
but what if I need to constantly send messages until I get a signal that the message has been read?
now I just run Task and don't await it
ah! the main problem is I don't understand when I should read response
it looks like long polling problem
why would you use long polling?
it's 2023, what browser do you need to support that doesn't support websockets?
i mean "long polling"
I can send notification to message broker and vue.js will catch notification with websockets
But I need to get response from client that notification has been read and I don't understand how to do it correctly
All I can do rn is ask message broker about a new response on the right topic
ah maybe I get the idea
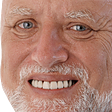
yes, I know you mean long-polling, that's why I asked why you would use it
what is the "message broker" here?
is that something running client-side?
rabbitmq
which means what?
https://www.rabbitmq.com/ you can check
this is a message broker which we use
which does what?