❔ Bytes to UInt64
I am having a issue trying to turn the hex output to ulong, (UINT64). I keep getting 6687895829614943646 from 5CD02DD2B303DD9E
I know 5CD02DD2B303DD9E should be 11447309898705915996 since my hex editor is right. I am using Little Endian.
Any help would be appreciated, thank you.
Here is my code:
32 Replies
That's in PowerShell
6687895829614943646 is the correct value
mtreit
REPL Result: Success
Console Output
Compile: 391.088ms | Execution: 25.128ms | React with ❌ to remove this embed.

how? o_O
mtreit
REPL Result: Success
Console Output
Compile: 826.622ms | Execution: 65.431ms | React with ❌ to remove this embed.
You are mixing up the endian-ness it seems.
Hmm
So what should I do?

this is how its supposed to look like etc
in hex anyway
i think my editor has the UInt64 as LE
even if the hex is BE
but i need the LE version after the BE input is done
In the code sample I pasted you can see the BinaryPrimitives type. You can use that to do either LittleEndian or BigEndian conversions, so just use that.
I will give it a shot, thank you so much 🙂
In the code, above, should I start over? A lot of stuff now.
I will keep my FNV1/FNV1A hash class of course
What is the intent of the code?
Generate a 64-bit hash?
That already works yes 🙂
But I want to convert it to ulong (UInt64)
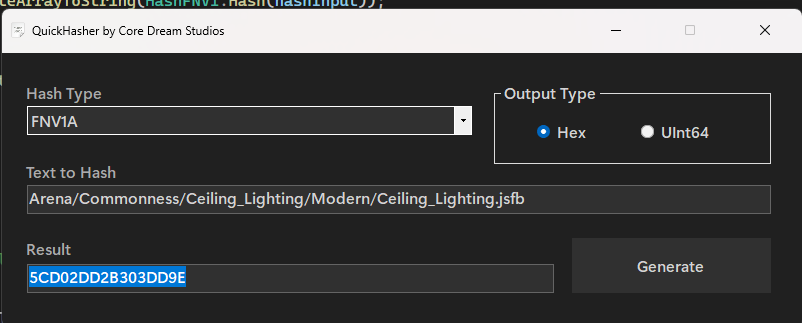
my dumb little app lol
doesnt need wpf for a simple 2 functions
By the way, output type of "hex" and output type of "UInt64" doesn't make sense. One is a data type and the other is a representation.
Like UInt64 can be represented as decimal, hexadecimal, binary, octal, whatever.
So I think you mean "Decimal" not UInt64
This feels like it could be three lines of code or so.
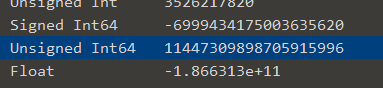
This is LE format though
what i have so far
need to figure out the foreach for the textbox, doing now
updated
issue with val, trying to figure out why it doesnt like the conversion, 1min
doesn't like string for some reason
long val = Convert.ToInt64(hashString, 16);
fixed that one so far
😐
Use ReadUInt64BigEndian or ReadUInt64LittleEndian
To turn the bytes into a number
5C
D0
2D
D2
B3
03
DD
9E
ok, thanks
made it worse, bleh, bbiab
not having any luck atm, but will try later
Wait, you just want the string representation of the hash as decimal or hex?
That's literally just this:
Sorry ,good morning. My internet was out the entire day. XD
I still need the Uint64, its not showing up
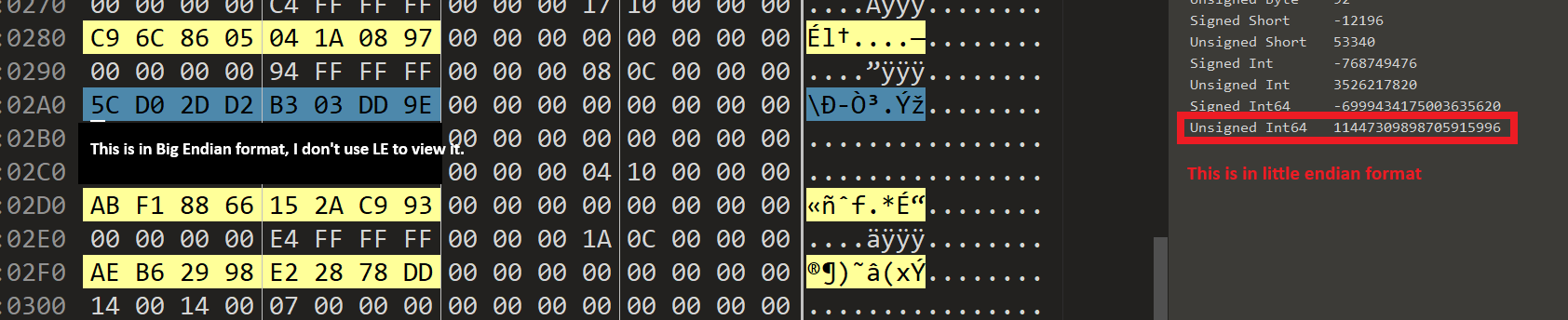
Hope this explains it more 😦
nm i got it
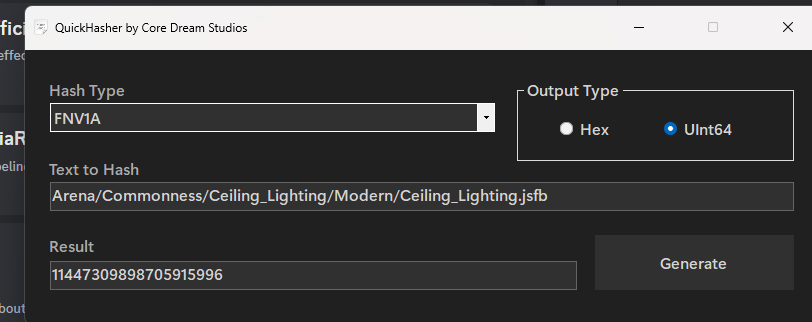
Thank you again @mtreit 🙂
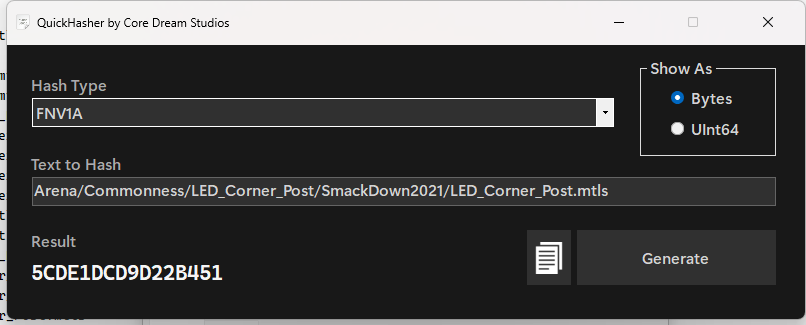
All set, thanks again for your help.
Was this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.