✅ ✅ C# Academy Calculator Project Challenge -- Counting Times Used
Calculator.mekasu0124/Program.cs - https://pastebin.com/Wvz9eHUM
ClassLibrary/CalculatorLibrary.cs - https://pastebin.com/qucuTXXm
This project had us create 2 projects in one. The calculator and the class library. One of the challenges is to keep track of the number of times the calculator was used. I want to do this based off when the user receives a result, not when the program may error due to
Invalid Inputs
, This operation will result in a mathematical error
, or Oh no! An exception occurred trying to do the math.\n - Details: ", + e.Message
. This project had a requirement of following the tutorial on https://learn.microsoft.com/en-us/visualstudio/get-started/csharp/tutorial-console?view=vs-2022 and so I did which is the same code in the top two links. In CalculatorLibrary.cs
we use
however in Program.cs
we use this try case
to determine the result and whether it errors or not. Under the line Console.WriteLine("Your result: {0:0.##}\,", result);
I want to add an UpdateCount();
function. I attempted to do
but this didn't work. So, following the same code patterns and such in the files, how would I increment the usage variable that is wrttien in at the top of the CalculatorLibrary.cs
file in the public Calculator()
function where I have writer.WritePropertyName("Usage"); writer.WriteValue(0);
?10 Replies
Well, uh, a json serializer serializes/deserializes... Json, not files. It can take a stream, but not a file path
So... read file -> deserialize to a class -> increment a property -> serialize it back -> write it back to the file
Alternatively, the same but with a stream
ok so the tutorial doesn't use model classes within it
so here's where I'm at in Program.cs
Ah, they're just discarding type safety and rawdogging it with JObject or something, gotcha
Well, not the way I'd do it lol
I fixed my code. It should've been
public List<OperationsModel> Operations
isntead
I, honestly, wouldn't do it the way the tutorial said to do it either. Especially when using models. I don't even use the JsonWriter that they used here either. I just use the regular wayI'm gonna be honest, I never worked with type-unsafe Json handling, so not sure if I'll be of any help there
I do not mind making this into type-safe classes whatsoever. I'm just not familiar with how they used JsonWriter to do the storing of the data so I have next to zero idea on how to convert the code
in the CalculatorLibrary.cs file I could made a save operation function that would take the num1, num2, operation, and result and put it through the OperationsModel class and then write that information, but I don't know if that would fail me for the project
Right, yeah
Some teachers can really be fossilized hard in their ways
I'll be honest, no clue
I think I'm going to ask them if I can do the calculator in a different way than the tutorial that way I can include type-safe classes
I'm waiting for a response, but I'm just going to re-write it anyways and attempt to submit while I"m waiting for a response. Thank you for attempting to help ❤️
So the purpose of the project is learning documentation and following it. Which is understandable. I still am unsure of how to use the StreamWriter the JsonWriter the way they have to keep track of the number of times the calculator is used, however, without the challenges included I’ve already passed the project so I’m going to move on for now to the next project. On a later date, I may re-write my own calculator to do the things they’re offering as challenges and incorporate type-safe data storage and usage, but for now I’m not going to worry with it
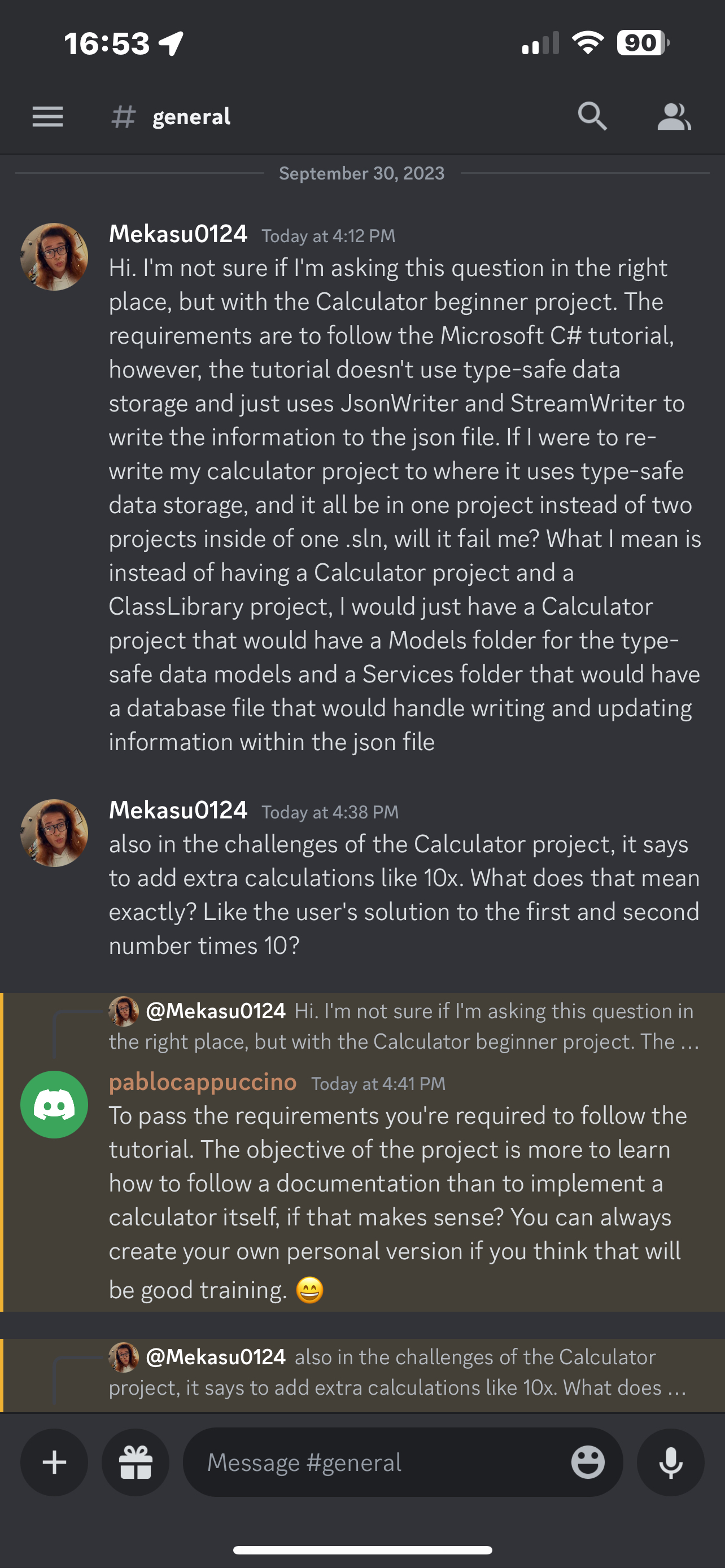
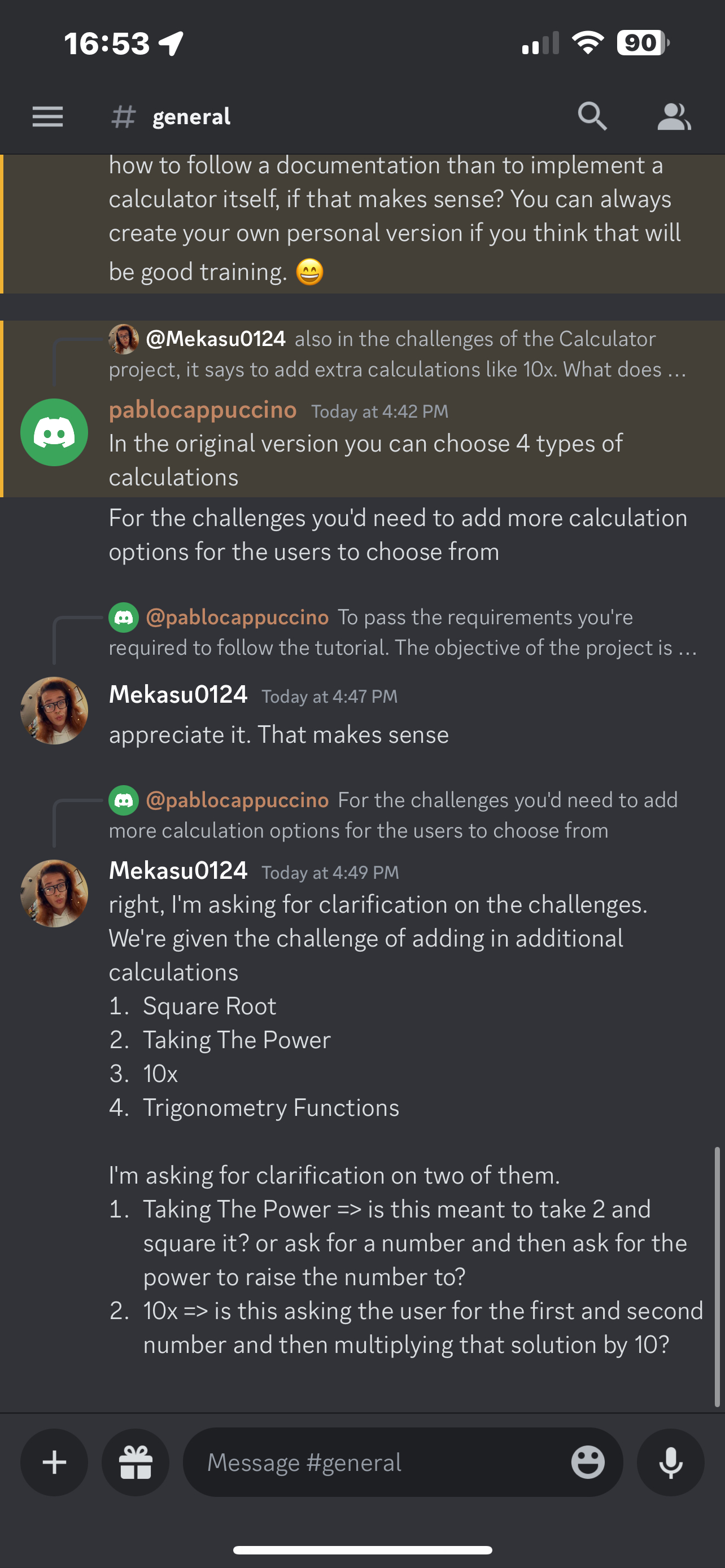
Was this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.