✅ ✅ Using SQLiteDataReader to return one item
I'm wanting to return just one item that is pulled from the database by it's ID. The only way I know how to use reader.Read() is inside of a while-loop and have it iterate through all items selected from the database table and then added to a list and returning the list, but I don't know how to use the reader.Read() to just return one item. Thanks
7 Replies
don't put it in a loop and just call Read once before constructing the object?
I found a stack overflow that showed it used in an if statement and so far that's working. Thank you 🙂
I have another question if that's alright
When I put in
18:53
for 6:53PM, it kicks this error. This is supposed to calculate the difference in time stamps. So if I put in 18:53 for the start time and 19:33 for the end time, it's supposed to give back the difference between those two times, however, it's saying that input isn't in the correct format for 18:33. What do I have wrong?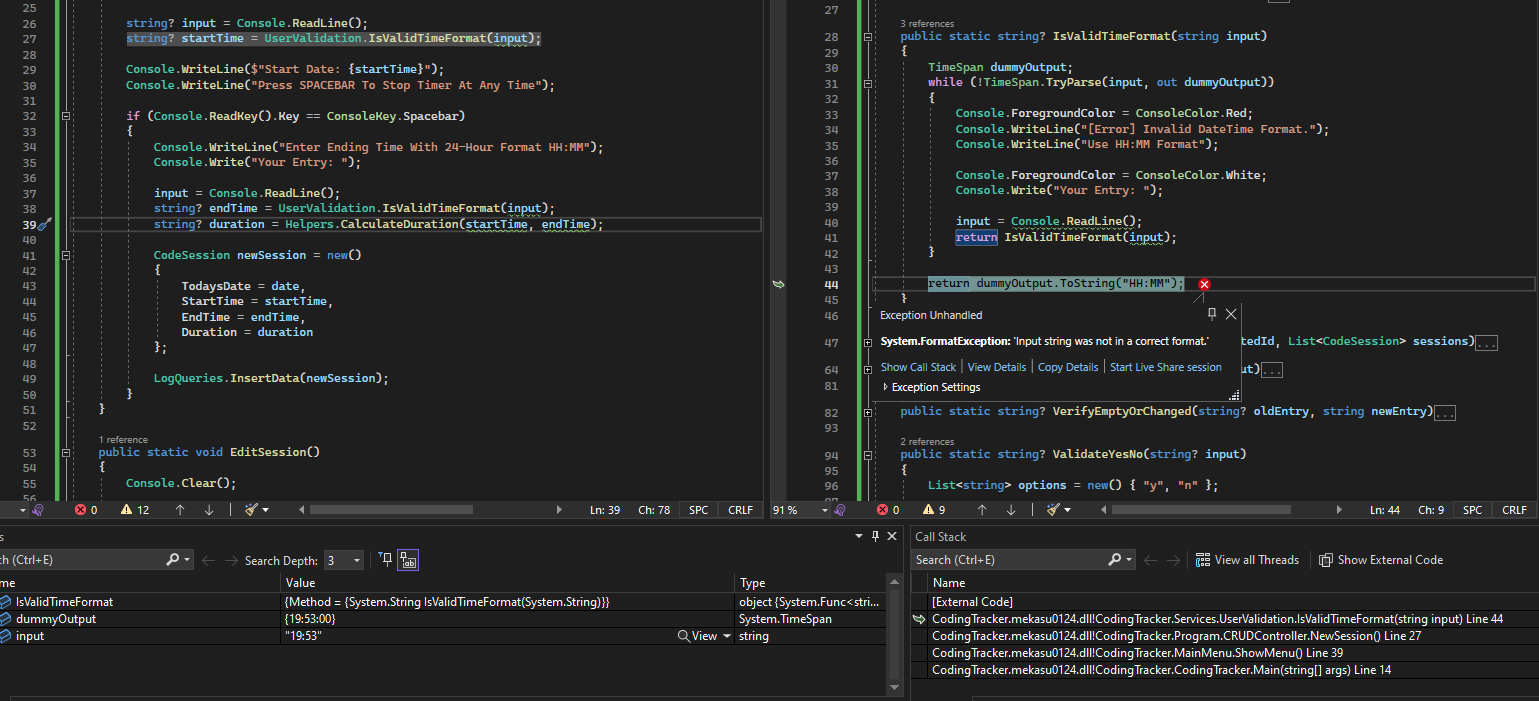
Mekasu0124
REPL Result: Failure
Exception: FormatException
Compile: 701.471ms | Execution: 43.111ms | React with ❌ to remove this embed.
!e
Was this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.