✅ ✅ Issues With Input Validation
I'm asking the user for information when they select to update an entry within the database. Their instruction is to just press enter and leave the entry blank if they want to keep the information the same, however, I also need this function to check a date format and a time format (two separate checks) and I can't figure it out. To clarify, The first entry is asking them for an adjusted date, or to leave it empty. When they put in something, it needs to check if that entry is empty or not and whether it matches
MM/dd/yyyy
format. Then it asks them for the updated start time, or to leave it empty. That needs to check if the entry is empty and whether it matches HH:MM
format and same for the third check. Thanks68 Replies
so like
When it prompts and the user enters their information, or leaves it blank and the validation function is ran, it needs to check
1. Is the string empty
2. If not empty, does it match
MM/DD/YYYY
3. If not empty, does it match HH:MM
and return the string when conditions are metSounds like you want three different methods instead of just one
Since you call them distinctly anyways
Yes but no. If it’s possible to have 3 checks in 1 call then id rather go that route tbh. I’m already having to write 100+ lines per file and I’ve only gotten 2/8 menu options completed besides this minor set back. I’d rather not have to write 15 more lines if I don’t have to
That's the wrong mindset tbh
Methods should be specific rather than broad
You certainly can do this with one method, but it'll be harder to reason about
Like, it will accept a date when you really expected a time
Or opposite
Tbh you’re right. I just wanted an easier way of it but frankly 15 lines in the same function or 15 lines between 3 functions are still 15 lines
yup
And most importantly, you prevent a time from being saved in the date field (or crashing because you expected the value to be parsable when it wasnt)
True. Thank you 🥰
ok so I'm having a problem with getting the order of calls right
this is the primary function. When it asks the user for the newDate, it checks to see if the input is empty or not.
these are the validation functions that I created for checking if the string is empty, or not. Check if it's the correct date format and time format. So since the first question deals with the date, how would I do the call order to check if the string is empty or not, and if not then check it's date format?
i uh... what
those methods are... what?
looped AND recursive AND returns
bool?
when it never returns null?
this is confusingok I'll fix it
your null annotations are all wrong in general
newEntry is flagged as never being null, but you check it for null
so I suggest you mark it as
string? newEntry
yep. I already fixed that
the
string? newEntry
part that isright.
I'm trying to wrap my head around your original issue now.
Ah okay I see it.
so it checks if the newEntry is empty or if the new entry matches the current entry. If it is empty or does match, it returns the current entry. Otherwise, it returns the new entry
you need the "allow null/empty to keep same value", while still validating dates/times
my gut instinct is to use higher order functions, but that might be... hard?
its the idea of passing functions as parameters to other functions
I haven't learned that yet
Would you be okay with learning it now, or prefer a simpler approach?
also, just so I can see all the types involved here can you show me the
CodeSession
class?and I'm not entirely sure on how to go about that. There are 3 entry inputs. newDate, newStartTime, and newEndTime.
1. all 3 check for if the newEntry is empty or matches the oldEntry so that if the newEntry is empty or matches the oldEntry, it can keep the same value that's currently recorded in the database.
2. newDate needs to check against the
mm/dd/yyyy
format to follow the requirements You should tell the user the specific format you want the date and time to be logged and not allow any other format.
3. newStartTime and newEndTime needs to check against the HH:MM
format to follow the same requirements.
The 3 are checked against the String.Format()'s in the event the user wants to change the information and doesn't leave the newEntry blank
they're all strings except the Id because of the Tip on the projects page Sqlite doesn't support dates. We recommend you store the datetime as a string in the database and then parse it using C#. You'll need to parse it to calculate the duration of your sessions.
okay
The simplest way to do this is to have your Date/Time validations allow a blank string
ie, they allow valid times/dates or blank string
so "" is a "valid time"
and would mean keep the old time
you could then call it like
updatedSession.StartTime = VerifyEmptyOrChanged(currentSession.StartTime, VerifyTimeInput(input));
so as long as the user is trying to use a non-blank value, its validated
but if its blank, we just say "oh you wanted to keep the old value, thats fine"
that solves your "order of operations" problem
input != String.Format("MM/dd/yyyy")
isnt valid code btw, as I'm sure you know
or actually it might be, but it doesnt do what you think it doesso then what's the better way to write it
the format check?
yea if it doesn't do what I think it's doing then how am I supposed to write it?
well for one, I can't find an overload for
string.Format
that takes 1 argument
what you probably want to do here, is check if the provided string input can be parsed as a date or time given a specific format
references:
https://learn.microsoft.com/en-us/dotnet/api/system.string.format?view=net-7.0
https://learn.microsoft.com/en-us/dotnet/api/system.datetime.parseexact?view=net-7.0
https://learn.microsoft.com/en-us/dotnet/api/system.timespan.parseexact?view=net-7.0
wait, should obviously be TryParseExact
https://learn.microsoft.com/en-us/dotnet/api/system.timespan.tryparseexact?view=net-7.0
https://learn.microsoft.com/en-us/dotnet/api/system.datetime.tryparseexact?view=net-7.0so like this
Something like that
try it out
you still have the weird loop+recursion thing thou
just remove the recursion?
yes because while the input isn't what it's supposed to be, it's to prompt the user for another attempt
your loop body does that
you gain nothing by calling the method again
brb 15-20 min
if their first input doesn't match, then it triggers the while statement and then they enter another input which triggers the while loop again as the while loop is the validation check
The loop is all you need
ok I removed the return statement
Just remove the recursive call inside the loop
I'd also urge you to use the debugger more
I do use the debugger
I just couldn't figure out the order of calls
here's where I'm at
so with testing it, it works
so now I have
the problem I have now, and yes I tested it before writing this, is that if I just press enter to leave the prompt empty, it doesn't allow for the string to be empty. So now the user has to input a date even if it's the same date to keep the same value so how can I edit the validation function to allow the string to be empty as well?
Pobiega
The simplest way to do this is to have your Date/Time validations allow a blank string
Quoted by
<@105026391237480448> from #✅ Issues With Input Validation (click here)
React with ❌ to remove this embed.
Im sure you can figure that out
so wrap it in an if statement to check if null or empty and if not then run validation loop
no
remove the
?
from the parameter?how does that help?
I guess it doesn't. was just a thought
what part of your validation method is doing the actual validation?
like, what is the condition that is being checked and decides if an input is valid or not?
the while loop
yep
so change it to say
while (!DateTime.TryParseExact(input, format, provider, style, out result) && !String.IsNullOrEmpty())
almost
well input belongs in the second check
yes 🙂
ok so then question
nevermind
so this function is ok as it is then, yea?
pretty much
ok thank you ❤️
last question for a while I promise
this is the function that gives the user a list of items in the database and asks them for the id of which one they want to delete and then a simple yes or no for confirmation before deleting. When I test this function, everything does fine except for where it asks the user Yes or No. When it gets to this part, it immediately goes straight to the error. What do I have wrong?
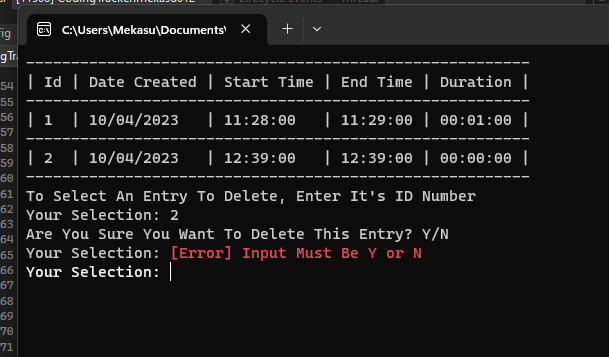
Y
is not the same as y
also.. uh
shouldn't this method return bool
?
and you have the recursion thing again
here is my helper method for "yes/no" prompts
for inspirationyou're right here. I just went through the entire validation file and removed all recurrsions within the while loops that were occuring
true. I've changed it to
string? selectedAction = Console.ReadLine().ToLower();
👍
I supposed it could. Not a bad idea
I'd probably do that inside the method btw
makes it easier to use the method
I just realized my mistake too
I have the validation set equal to where the selected action is, but never prompt the user for the initial input
😄
this is pretty neat. I didn't know about ToLowerInvariant to allow a check for capitolized or not
ToLowerInvariant is just like ToLower, but ignores your current culture
its just a habit I have
I like switches like this thou
and the pattern matching you can do is nice
ok so not going to lie. It looked a lot smarter than what I had so I used most of it. Hope that's ok. My question is, if neither case happens, like the user enters Dog or cat instead of y, yes, n, or no. What makes it iterate again and ask for another input like the while loops?
yep!
while(true) loops forever
so the only way out is to type one of the valid cases, so the
return
statements triggerand the break makes it iterate again
that makes sense
the break is related to the switch
not the while
oh ok maybe I didn't quite grasp it then 😂
while loop lasts forever
correct condition between the two case statements returns out of the switch and breaks the loop as well?
so if the switch case doesn't return out, then the loop never breaks
return
is always "out of the method"
only methods can return
so return stops the entire methodright
and break allows the rest of the code to continue as it only breaks out of the switch statement itself and not the function as a whole
yes
so it hits the while loop again
that's pretty dope 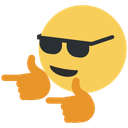
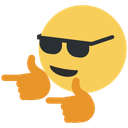
Was this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.