❔ ngl ion really get what this does
string[,] beach = {
{"" , "" , "" , ""},
{"farmer", "wolf", "rabbit", "carrot"}
}; so arent we suppsoed to use an array or lsit for several strings/objects? how does the string data type hold all of those 4 strings? or like... shi idk what i dont know anymore
}; so arent we suppsoed to use an array or lsit for several strings/objects? how does the string data type hold all of those 4 strings? or like... shi idk what i dont know anymore
51 Replies
a
string[,]
is a multidimensional array
This means it's like a grid, it has rows and columnswhats the point of even using arrays then
or like
are they the same thing
a string can be an array, and they are not mutually exclusive?
If you space out the items a bit, you can see this
the first pair of
{}
is the first row, the second is the second row
A string isn't an arraybut... can be?
You can have an array of strings
I.e. an array which contains zero, one, two, or any other amount of strings
okay i think i get it
right so
what i see
is a array inside an array
(hope i used array correctly here)
Pretty much, you can think of a
string[,]
as an array which contains several arrays which in turn contain several strings
The array here contains two arrays, each of which contains four stringsokay so
yea
we have 2 arrays
with 4 strings in em each
first array is empty
second has the stuff in it
well, the first array isn't empty, it just contains four empty strings
ye thats what i meant
mb
how do i like
refer to the first array
beach[1] or osmething?
well uhhh using multidimensional arrays, you can't
i already forgot everything i learned 😭
If you wanted to refer to the entire first array, you'd have to use a
string[][]
which is actually just an array of arrays of strings
a string[,]
is like a grid, but you can't easily refer to entire rows/columnsThinker
REPL Result: Success
Result: string[]
Compile: 386.044ms | Execution: 36.839ms | React with ❌ to remove this embed.
hmm
thats what i was trying figure out
Generally,
string[][]
is more useful than string[,]
so basically... its a way to take information from somewhere..?
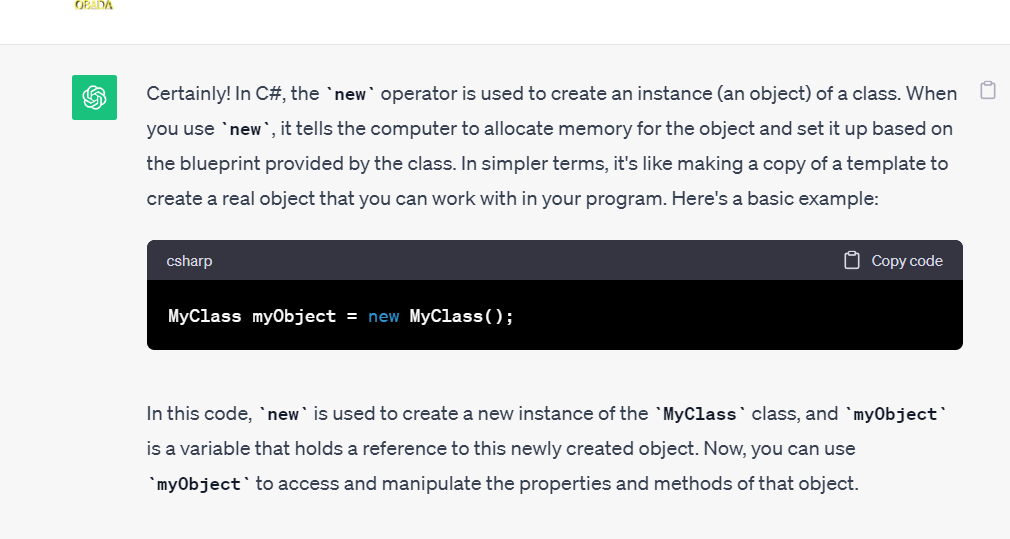
god chatgpt
although it's not really wrong
yeah so that's for classes, though in this case it's for arrays
its worth it to mention all i have regarding experience is a tiny tiny tiny tiny bit of experience with python
and python neve did me dirty liek c#
yeah so arrays in C# work a bit differently than in Python
also il still tryina understand string[][] beach = new[]
im*
why we gotta put [][] after string[][] beach
isnt beach already an array with 2 arrays?
there are two ways to create an array
In the first example, we tell C# to create an array which has four elements off the bat. We then set each of the four elements individually to a string.
In the second example, we create a new array, but we immediately give the elements we want to fill the array with. This makes us not have to specify how big the array is, because C# sees that we want to fill the array with four elements.
However, the second example can actually be simplified:
This does the exact same thing as the second example, except we don't need to specify that we want to create an arrays of strings, because C# can figure that out for us based on what we want to fill the array with. In technical terms, this is called type inference and is something you'll see in some other places in C#.
i think i understand
Now, what if the want to create an array of arrays? Well, it's very similar
This looks kind of odd, but it's really the same thing. We want to create a new array, of
string[]
s. So the elements we give are also string arrays.putting string infront of new resticts us to only have strings in the array, which is not needed cuz c# as u said canfigure out whats in the array
yeah
Although do note that this does not mean we can put everything inside the array, unlike in Python
but uhm
does it become impractical if we wanna say have an array of 50 arrays?
then we gotta spam []
Well, you would have to do that if you want an array of arrays of arrays of arrays of ... of arrays of strings
But in practice you never need that many nested arrays
i mean like an array which has say 50 arrays
not that they are all insdie eachother
right
Well, that is when something like a for loop could come in handy.
Say you want to create an array of 10 strings, but don't want to write it out manually
Thinker
REPL Result: Success
Result: string[]
Compile: 435.057ms | Execution: 51.387ms | React with ❌ to remove this embed.
Here we create a new string array of length 10, then fill it with
"a"
using a for loop.dont we have to write it manually?
like if i have 2 arrays containign 10 arrays each,
i name the 2 arrays school a and the other school b
then the 10 arrays are classes, which have strings of names of students
Well, you should probably use a class for that, but sure
i see
we still havent learned about class so i think i should hold off for now
what i wanted to do to begin with is move the info from line 1 to line0, then line 0 becomes blank
but i gotta learn to do it
so no spoilers
now that we have used new operator to get the info
Thinker
REPL Result: Success
Result: string[]
Compile: 470.938ms | Execution: 57.404ms | React with ❌ to remove this embed.
i can only assume we will need a way to assign said info to line 0
then maybe use a loop to set all the balues in 1 to blanks
am i somewhere in the right direction
hot or cold
I don't really understand what you mean
the values or objects in the array[1] (aka {"farmer", "wolf", "rabbit", "carrot"})
i want to assign those values to array[0]
then assign blanks to array [1] (so that array[1] becomes "", "", "", "" )
basically an ol' switcharoo
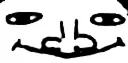
Well, you could do this (if you already have the array
strings
) well there goes the no spoilers
but lets see
i see
we break it down into 2 arrays
and simply swap values
correct?
yep
so fill code would be
idk if we need curly brackets or not
well, no, because you're still using
string[,]
we prob need
which has to change to string[][]
?
if you want to be able to swap entire arrays like this, yes
and doing so makes it like fully 2 seperate arrays rather than.. a grid..?
yep
aight so
the solution is beautiful and all
but for learning and knowledge purposes
what if like
we keep it as string[,]
use new operator to get the info out
and in some way assign it to the other array
then use loops to make array[1] have blanks
i know the soluition isnt good but for learning purposes
but i assume here we would have to split it so like string[][] to we ca nactually assing a variable to it so the nwe can actually assign stuff to it..?
Was this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.