✅ Converting String To DateTimeOffset & Getting Average Days To Goal
I started a new thread as this is a new thing. I need to create a helper function that takes in 3 parameters to calculate the number of days the user needs to spend coding based off the number of hours per day they want to put towards this goal in order to get the number of days it will take them to achieve said goal. I've never done this before, not even in Python, so I have no idea how to start or what to do. Thanks in advance
Edit: I started giving it a shot, and this is where I'm at
69 Replies
Why is hoursperday a string?
That seems silly
Again, why is it a string?
Use the correct data types everywhere
my database stores string so I'm using string
Why does your database store it as strings...?
Yeah, that just means you have one more place it's stored incorrectly
it's not "stored incorrectly" just because I chose to convert my information to strings. They're easier for me to handle and work with when it comes to formatting and such for console display so instead of trying to convert everything when I'm ready to display, it's already stored as needed
Yes it is.
It absolutely is
but give me 20 minutes and I'll delete my database file, redo all of my stuff to "the correct types" and put information back in
I'm sorry to be blunt, but you will write better code this way
It's honestly quite frustrating helping you, as you very often "fight back" against the suggestions you yourself ask for, given by people with many many years of professional experience.
formatting and parsing should be processes outside of code that does the actual "work"
also, as someone who inherited a database that stores everything as text instead of correct data types it will bite you later
I had a response typed, but honestly it's just not worth it. The position I'm in is either to conform to everyone else, or don't and if I don't then I get threatened with not getting help, or getting questioned about my code that has nothing to do with the question I asked. I understand that there's a preferred method and a preferred way of doing things, but at the end of the day it's my code and I'm responsible for it. I don't work for any major companies and I do this as a hobby just to learn things while I'm in school. Sorry to waste everyone's time, but I'm not the conform-to-everyone type. Sorry to waste your time
doesn't proper database design (or data modeling in general) fall under "learn things?"
"Hey, I used the garden hose to fill up my car with water instead of fuel, and now the lights don't work. How do I change the lightbulb?"
Of course people will question why you filled your car's gas tank with water
And will, rightfully, recommend to not do so
And when we suggest something and you say "no, give me a new suggestion, one that better adheres to my ideas of what is correct" that... well it kills all enthusiasm I have for helping
Because like it or not, you're in the wrong here, and then blaming us/me for not wanting to help when you obviously don't care about what we say, only that we fix your program...
never said I wasn't in the wrong and never blamed anyone
I've probably personally spent close to 20 hours helping you. Why do you think I did that?
also, fwiw this code does have a lot to do with the question you asked because it's causing problems when you're trying to do calculations and you have strings instead of proper dates/numbers
if you get to a point where you have more complex SQL queries that filter based on these columns you will run into additional issues
Yeah, strings can't be compared easily, or have math done on it
And databases are really good at doing comparisons
well to render this all mute so everyone can continue with their day instead of being busy debating with me, I never fought back. That's just how it was viewed and taken. All I did was state that I store my information as string as it's easier for me to work with. I never blamed anyone, I just stated that my code gets questioned about things that have nothing to do with my question. As far as conforming to the "like mindset", even though I'm not that type of person, I've gone in and changed my data types in my code. I just have to do it on database creation. As per being in the wrong, I never said I wasn't. As far as everything else, it is what it is. Again, I've gone back and changed the data types and will figure it out from there. Thanks and sorry to be such a headache to everyone
redoing code isn't a big deal and doesn't make you some kind of "conformist" since that seems to be the pain point for you
like, i just spent all afternoon ripping out what i thought was a great system at the time but it turned out to be shit
We spend more rime changing existing code than adding new for sure
also especially in programming, if everyone is doing something a certain way that's probably an indicicator that it's a good way to do it
that's why there are so many memes about stealing code, code you didn't write, etc
^ that's part of the reason I don't write my code like a vast majority of other programmers do. It helps prevent that
prevents what?
stealing code
how?
and would you rather write lower quality code just so nobody wants to "steal" it?
I mean, I guess people are less likely to steal a rusted Multipla with missing doors than they are to steal a brand new Rivian...
But if you're writing the Multipla of code, it's unlikely it's even worth stealing it in the first place
(which seems against your best interests if your goal is to learn)
Yeah
if you have an application that is valuable enough to steal, you use legal options to deal with it
the style of code won't stop anyone
well like I said before, I went back and changed my data types, and worked through figuring out the conversion myself and I have this for the code
So, we have the amount of hours they want to work per day... but we don't heve the total amount of hours necessary to complete the task
I don't need that part. I just needed the user's input of how many hours per day they wanted to work and then return the number of days it'll take to complete based off that input
Yeah, aight, but... how do you plan to calculate it?
that's in the code block
Right
So you get the amount of time between two days
Then you divide it by how much time per day the developer can write code
And what you get back is...?
a double
Well, yeah
But it's not days
It's not hours
It's "units of coding"?
"Sprints"?
It can't be any unit of time, because the amount of time from start date to end date is fixed
Mekasu0124
REPL Result: Success
Console Output
Compile: 731.908ms | Execution: 59.567ms | React with ❌ to remove this embed.
this returns 3.25 days until the goal is achieved
Uh, no, it does not
ok yea I see that now
It calculates how many times the developer has to sit down and work for
hoursPerDay
hours, to finish the project started at startDate
by endDate
wait
that's weeks. apologies. 3.25 weeks
30 - 4 = 26 days / 8 hrs/day = 3.25 weeks
now I done got myself backwards.
this is the challenge that's supposed to match, but I've got myself backwards
ok so I'm requesting the user's input for the start and end date of the goal
Yep
and hours per day is the output, not the input
I'm returning the days until the end of the goal here
so that they know how long they have until the end of the goal
Yeah
these are the requirements. I've fulfilled all of those
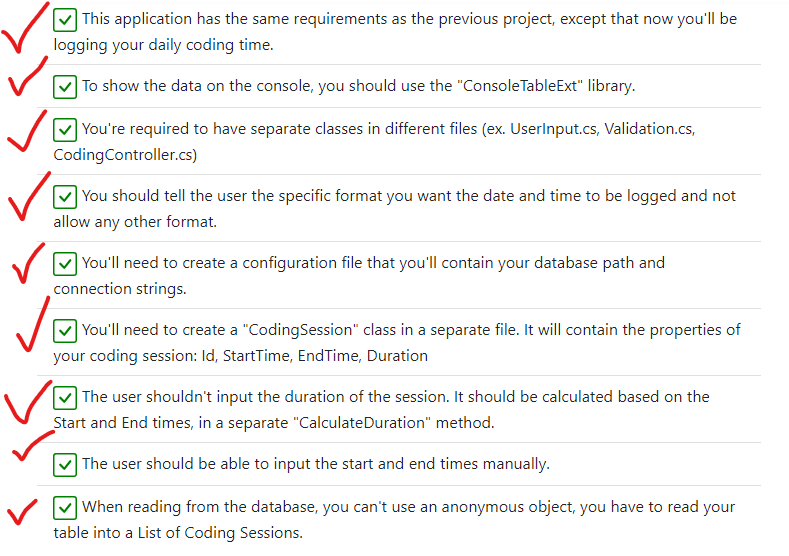
these are the challenges to the project. I've got the first one done
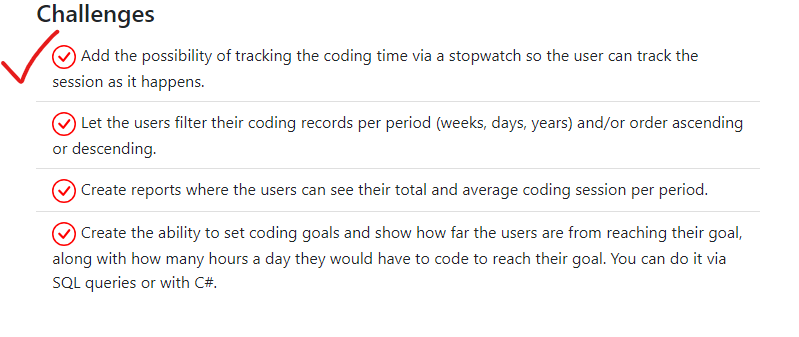
so the trick with the bottom part
Create the ability to set coding goals and show how far the users are from reaching their goal, along with how many hours a day they would have to code to reach their goal. You can do it via SQL queries or with C#since I am storing the
DaysToGoal
and HoursPerDay
in the database, I'll need to have a check system that checks the current date against the end date so that it can update the database once per day to keep the DaysToGoal
and HoursPerDay
updated so that when the user selects the menu option View All Goals
, that information is updated accurately
but it doesn't say anything about setting a limit on the user of how many days they should or can spend coding so the hours per day would just be done with a number that I choose to base it off of, right?The way I understand it, the goal is something like "between today and next week I want to spend 60 hours doing X"
Then you need to calculate how many hours each day will the user need to spend to meet this goal
ok. I can see that. The way that I'm understanding it though is
1.
Create the ability to set coding goals and show how far the users are from reaching their goal
so get user input of start and end date for goal and return number of days in between
2. along with how many hours a day they would have to code to reach their goal
is quite open-ended. We can't control how long a person wants to spend coding, so either set a limit or base if off of like 8 or 10 hours spent per day.Well, no, we can't control it
But we can tell them how many hours they would need to work
Say, the goal is to workout 10 hours in 5 days
That would mean the user will need to workout for 2 hours a day
so I still need to get the number of hours the user wants to work as a user input?
The total amount of hours, yes
At least to my understanding
ok so I've asked the user for the Goal Name, Start Date, End Date, and Hours Per Day. I've calculated and returned an integer instead of a double on the number of days until the goal is complete
so with them deciding on how many hours per day they want to spend coding, there's nothing left for me to do
except write a method that checks todays date against the end date of each goal in the database, and updates the Days Until Goal count so that when the user wants to view all of their goals, the information is accurate
I have a question if that's alright do you mind if I dm you? it's harmless. promise
Sure
Ah, no, my DMs stay closed
oh ok. no problem.
I wanted to ask if you'd be willing to do a code review on something else that I built. It's a console based trivia game and I'm a bit shakey on it
that's what I was gonna dm you. I just didn't want anyone to do a code review and then I have to filter through multiple reviews
There's #code-review if you need it
I appreciate it. I just wanted one person to do a review, but I'll take a look at the channel
ok so I've ran into another problem and I'm not quite sure how to fix it
When going through testing, I get this back as a return
is something wrong with my validation?
also, tbh, it's my first time doing variables with question marks to negate null information. idk if that helps at all
I'm going to go through and remove all of the
?
from my code. I'm not entirely sure on how/when to use them, so I'm just not goign to use them yetC# expects the date to be in a specific format
ISO8601 to be more precise
So
yyyy-MM-dd HH:mm
that's my bad. I didn't share all the code
if the user presses enter to leave the current value the same, it's supposed to return the current value
this is the user entry part full code https://pastebin.com/GsrdbkXr
Interesting. Even with passing format as
MM/dd/yyyy
this actually formats the date in a different format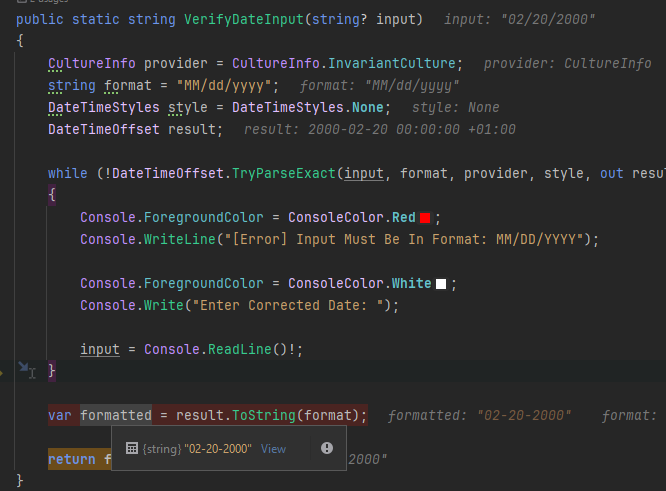
right and even with removing all of my
?
's from various variables and parameters that I was using them in, still didn't fix it. It's still returning 01/01/0001please tell me you didnt actually do that
you just ruined your null annotations
writing them as you write code is fine, putting them in on existing code is a painful process
also, unless you have enabled nullable warnings as errors they don't really affect the compilation/behavior of your code so i wouldn't expect anything to change regardless
their purpose is to help you avoid null references in places they shouldn't be allowed (for reference types, anyway)
I can put them back no problem
so I've got it all put back. With my issue of the date thing though, I think my solution is to break those back out. So instead of having
I should have
or I could have
VerifyEmptyOrChanged
return a true/false condition like
I'll be back later. I have to go take a midterm
so I did some playground testing, and have decided to turn the function that checks null or empty and if the conditions match into a boolean return. This actually returns what is expected given different conditions. So now to implement it into the program and test it with those conditions
sorry to bother you, however, I need a smidge of help. I'm trying to mock up getting the total time and average time of coding sessions in my database. I've created a small mock session, but I'm getting format issues from my second function https://pastebin.com/hw8dd8wX would you mind to take a look?
nvm I got it
ok so I don't got it
so I'm trying to get all of the session durations, add them together and show the total time and then take that total time and divide it by the number of durations to get the average total time between all the sessions, but I just keep getting "04:00:00
for both print statements.
ok so I'm actually just an idiot. There's nothing wrong with the conversions. I thought there was because each session has the same time duration. 4 hours time 7 sessions is 100,800 and then divide that by 7 and you get 14,400 back which is 4 hours